With the continuous development of Web applications, more and more developers are beginning to pay attention to the performance and concurrent processing capabilities of Web applications. As a scalable, high-performance network communication framework, Swoole is also being paid attention to and used by more and more developers. In this article, we will detail how to implement a scalable HTTP route processor using Swoole.
1. Introduction to Swoole
Swoole is a high-performance network communication framework based on PHP. It provides a variety of programming methods such as asynchronous, coroutine, and parallelism, and can be used to build high-performance network services or Web application. The main features of Swoole include:
1. High performance: Swoole uses underlying asynchronous and non-blocking IO technology, which can greatly improve system performance and concurrent processing capabilities.
2. Simple and easy to use: Swoole provides a rich API and out-of-the-box components, allowing developers to easily build high-performance web applications and API services.
3. Extensible: Swoole supports a variety of programming models and expansion methods, such as coroutines, process pools, Worker processes, Task processes, etc., which can meet the needs of different applications.
4. Stable and reliable: Swoole has been verified for stable operation and reliability in multiple high-concurrency scenarios, and is one of the preferred frameworks for building high-performance network services.
2. Introduction to HTTP routing processor
HTTP routing processor is an important component in web applications. It is used to route HTTP requests to the corresponding processing method or controller. Normally, the HTTP route processor needs to support the following functions:
1.URL parsing: parse the URL of the HTTP request into the corresponding controller and method.
2. Route matching: Match the parsed URL with existing routing rules to find the corresponding controller and method.
3.HTTP request processing: execute the corresponding controller and method and return the result to the client.
In order to facilitate developers to use, there are many mature HTTP routing frameworks on the market, such as Laravel's Router, Symfony's Routing component, Slim's Router, etc. These frameworks provide easy-to-use APIs and highly customizable configurations, allowing developers to quickly build web applications and API services.
3. Swoole implements scalable HTTP routing processor
In Swoole, high concurrency and scalable networks can be achieved through various methods such as coroutines, process pools, Worker processes, and Task processes. Serve. Below we will introduce in detail how to use Swoole to implement a scalable HTTP route processor.
1. Routing configuration
First, we need to define a routing configuration table to map the requested URL to the corresponding controller and method. In the routing configuration table, we can define different request methods (such as GET, POST, PUT, DELETE, etc.), routing rules and corresponding controllers and methods.
The sample code is as follows:
$routes = [ 'GET /user/{id:d+}' => 'UserController@show', 'POST /user' => 'UserController@store', 'PUT /user/{id:d+}' => 'UserController@update', 'DELETE /user/{id:d+}' => 'UserController@destroy', ];
In the above code, we define four routing rules, which are used to handle the viewing, creation, update and delete operations of user information. Among them, {id:d} means that the id parameter must be a number.
2. Route analysis
In Swoole, we can use the SwooleHttpRequest object and SwooleHttpResponse object to handle HTTP requests and responses. After receiving the request, we need to parse the requested URL into the corresponding controller and method, then execute the corresponding method and return the result to the client.
The sample code is as follows:
$http = new SwooleHttpServer('0.0.0.0', 8080); $http->on('request', function ($request, $response) { $routes = [ 'GET /user/{id:d+}' => 'UserController@show', 'POST /user' => 'UserController@store', 'PUT /user/{id:d+}' => 'UserController@update', 'DELETE /user/{id:d+}' => 'UserController@destroy', ]; $method = $request->server['request_method']; $uri = $request->server['request_uri']; $route = $method . ' ' . $uri; if (isset($routes[$route])) { list($controller, $action) = explode('@', $routes[$route]); $params = $this->parseParams($uri, $controller); $response->end((new $controller)->$action(...$params)); } else { $response->status(404); $response->end(); } }); $http->start();
In the above code, we first define an HTTP server and register a request event callback function. In the callback function, we first splice out the corresponding routing rules based on the requested method and URI, and then map it to the corresponding controller and method based on the routing rules. Finally, we call the controller's method and return the parameters and response content to the client.
It should be noted that in actual use, we usually process route resolution and controller method execution separately to increase code scalability and testability. For example, we can encapsulate route resolution in the Router class, encapsulate the execution of the controller method in the Controller class, and then call it in the HTTP request processing function.
3. Controller method execution
In order to achieve better code reuse and testability, we can process the controller method and route resolution separately. For specific implementation methods, please refer to the following sample code:
class UserController { public function show($id) { // TODO: 根据ID查找用户信息 return json_encode(['id' => $id, 'name' => 'Alice']); } public function store(Request $request) { // TODO: 创建新用户 return json_encode(['status' => 'success']); } public function update($id, Request $request) { // TODO: 根据ID更新用户信息 return json_encode(['status' => 'success']); } public function destroy($id) { // TODO: 根据ID删除用户信息 return json_encode(['status' => 'success']); } } class Router { private $routes; public function __construct(array $routes) { $this->routes = $routes; } public function resolve($method, $uri) { $route = $method . ' ' . $uri; if (isset($this->routes[$route])) { list($controller, $action) = explode('@', $this->routes[$route]); return [$controller, $action]; } else { return null; } } } class Controller { protected $request; public function __construct(Request $request) { $this->request = $request; } protected function parseParams($params, $controller, $action) { $ref = new ReflectionMethod($controller, $action); $args = $ref->getParameters(); $results = []; foreach ($args as $arg) { $name = $arg->getName(); if ($arg->getClass()) { $class = $arg->getClass()->name; $results[] = new $class($this->request); } else if (isset($params[$name])) { $results[] = $params[$name]; } else if ($arg->isDefaultValueAvailable()) { $results[] = $arg->getDefaultValue(); } else { throw new Exception("Missing parameter: {$name}"); } } return $results; } public function callAction($controller, $action, $params = []) { if (!method_exists($controller, $action)) { throw new Exception("Undefined action: {$controller}@{$action}"); } return call_user_func_array([$controller, $action], $params); } }
In the above code, we first define a UserController class, which contains four methods for CRUD operations that process user information. Then, we defined a Router class to parse the routing information of HTTP requests. Finally, we defined a Controller class as the base class for all controllers, which is used to implement functions such as controller method invocation and parameter parsing.
In the HTTP request processing function, we first use the Router class to parse the requested routing information, then call the specific controller method through the Controller class, and pass the parameters to the controller method after parsing. In this way, we can process specific business logic in the controller method and return the corresponding response content.
4. Conclusion
Through the above introduction, we can see that it is not difficult to implement an scalable HTTP routing processor using Swoole. Compared with the traditional HTTP routing framework, Swoole provides more powerful and scalable functions, such as coroutines, process pools, Worker processes and Task processes, to meet the needs of different applications. Therefore, we believe that Swoole will play an increasingly important role in future development and become one of the preferred frameworks for building high-performance network services.
The above is the detailed content of Swoole implements scalable HTTP route processor. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
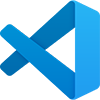
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Chinese version
Chinese version, very easy to use
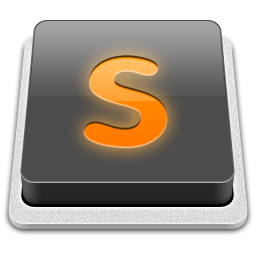
SublimeText3 Mac version
God-level code editing software (SublimeText3)
