


PHP development: How to use JWT for authentication and authorization
In modern web application development, security has become an integral part. Authentication and authorization are crucial in this regard, as they ensure that only authorized users can access protected resources. There are many authentication and authorization mechanisms available, of which JWT (JSON Web Token) is a particularly popular mechanism because it is simple, flexible, scalable and secure. In this article, we will explore how to use PHP and JWT for authentication and authorization.
Part One: JWT Basics
JSON Web Token (JWT) is an open standard for securely transmitting claims and authentication information over the web. It consists of three parts: header, payload and signature.
The header contains algorithm and token type information. An example of it might look like this:
{ "alg": "HS256", "typ": "JWT" }
The payload contains the information that needs to be transmitted. For example, username, role and other information about the user. An example of a payload might look like this:
{ "sub": "1234567890", "name": "John Doe", "iat": 1516239022 }
A signature is an encrypted string containing the key used to verify that the JWT is valid. An example of a signature might look like this:
HMACSHA256( base64UrlEncode(header) + "." + base64UrlEncode(claims), secret)
Part Two: Implementing JWT using PHP
In order to use JWT for authentication and authorization, we need a PHP class to generate and validate the JWT. Let’s look at the implementation of a simple PHP JWT class.
<?php class JWT { private $header; private $payload; private $secret; public function __construct($header, $payload, $secret) { $this->header = $header; $this->payload = $payload; $this->secret = $secret; } public function encode() { $header = $this->base64UrlEncode(json_encode($this->header)); $payload = $this->base64UrlEncode(json_encode($this->payload)); $signature = $this->base64UrlEncode(hash_hmac('sha256', $header . '.' . $payload, $this->secret, true)); return $header . '.' . $payload . '.' . $signature; } public function decode($jwt) { $parts = explode('.', $jwt); $header = json_decode($this->base64UrlDecode($parts[0]), true); $payload = json_decode($this->base64UrlDecode($parts[1]), true); $signature = $this->base64UrlDecode($parts[2]); $result = hash_hmac('sha256', $parts[0] . '.' . $parts[1], $this->secret, true); if (hash_equals($result, $signature)) { return $payload; } else { return null; } } private function base64UrlEncode($data) { return rtrim(strtr(base64_encode($data), '+/', '-_'), '='); } private function base64UrlDecode($data) { return base64_decode(str_pad(strtr($data, '-_', '+/'), strlen($data) % 4, '=', STR_PAD_RIGHT)); } }
In this PHP class, $header
, $payload
and $secret
store the JWT header, payload and secret information. The encode()
method uses header
, payload
, and secret
to generate a JWT string. decode()
Method uses the given JWT string and key to get the payload information. The
base64UrlEncode()
and base64UrlDecode()
methods are used to encode and decode the JWT header and payload.
Part Three: Using JWT for Authentication and Authorization
Now, we have understood the basics of JWT and have a PHP JWT class for generating and validating JWT. So, how do we use JWT for authentication and authorization?
First, when a user logs in, a token can be sent using JWT to authenticate their identity. The server will generate a JWT with the username and password payload and send it to the client. The client will send this token as the Authorization header of the Bearer authentication scheme on every request. The server will extract the JWT from the header and verify its validity if necessary.
The following is a sample JWT implementation for authentication and authorization:
<?php // 使用 JWT 登录 function login($username, $password) { // 检查用户名和密码是否正确 $is_valid = validate_user_credentials($username, $password); if (!$is_valid) { return null; } // 生成 JWT $header = array('alg' => 'HS256', 'typ' => 'JWT'); $payload = array('sub' => $username); $jwt = new JWT($header, $payload, $GLOBALS['secret']); $token = $jwt->encode(); // 返回 JWT return $token; } // 验证 JWT function validate_token($jwt) { $jwt = new JWT($header, $payload, $GLOBALS['secret']); $payload = $jwt->decode($jwt); if ($payload == null) { return false; } // 验证成功 return true; } // 保护资源 function protect_resource() { // 检查 JWT 是否有效 $jwt = get_authorization_header(); if (!validate_token($jwt)) { http_response_code(401); exit(); } // 资源保护 // ... } // 从标头获取 JWT function get_authorization_header() { $headers = getallheaders(); if (isset($headers['Authorization'])) { return $headers['Authorization']; } return null; } // 检查用户名和密码是否正确 function validate_user_credentials($username, $password) { // 验证用户名和密码 // ... return true; } // 密钥 $GLOBALS['secret'] = "my-secret"; // 登录并获取 JWT $token = login("username", "password"); // 将 JWT 添加到标头 $headers = array('Authorization: Bearer ' . $token); // 保护资源 protect_resource($headers);
In this example, the login()
method uses the JWT
class Create a JWT and return it for consumption by the client. validate_token()
method can verify the validity of JWT. If the JWT is invalid, false will be returned.
get_authorization_header()
Method extracts the JWT from the request header. protect_resource()
The method is used to protect web resources. If the JWT is invalid, this function will raise a 401 error.
Finally, a secret is defined in the global scope, $GLOBALS ['secret']
, which should be a long string. Note that this password is a secret password and should not be included in your code base. Typically, this key is stored in an environment variable and managed by the operating system running the web server.
Conclusion
JWT is a very simple, flexible, scalable and secure mechanism that can be used for authentication and authorization in web applications. In this article, we have looked at the basics of JWT and seen a sample PHP class for generating and validating JWT. We also saw how JWT can be used for authentication and authorization. Now you can try JWT in your web applications to ensure your apps are more secure.
The above is the detailed content of PHP development: How to use JWT for authentication and authorization. For more information, please follow other related articles on the PHP Chinese website!
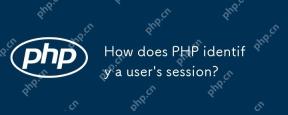
PHPidentifiesauser'ssessionusingsessioncookiesandsessionIDs.1)Whensession_start()iscalled,PHPgeneratesauniquesessionIDstoredinacookienamedPHPSESSIDontheuser'sbrowser.2)ThisIDallowsPHPtoretrievesessiondatafromtheserver.
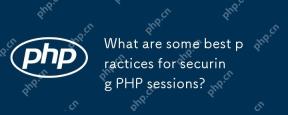
The security of PHP sessions can be achieved through the following measures: 1. Use session_regenerate_id() to regenerate the session ID when the user logs in or is an important operation. 2. Encrypt the transmission session ID through the HTTPS protocol. 3. Use session_save_path() to specify the secure directory to store session data and set permissions correctly.
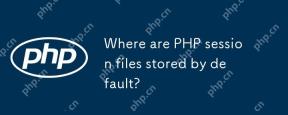
PHPsessionfilesarestoredinthedirectoryspecifiedbysession.save_path,typically/tmponUnix-likesystemsorC:\Windows\TemponWindows.Tocustomizethis:1)Usesession_save_path()tosetacustomdirectory,ensuringit'swritable;2)Verifythecustomdirectoryexistsandiswrita
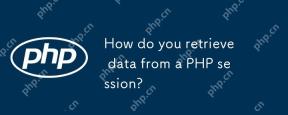
ToretrievedatafromaPHPsession,startthesessionwithsession_start()andaccessvariablesinthe$_SESSIONarray.Forexample:1)Startthesession:session_start().2)Retrievedata:$username=$_SESSION['username'];echo"Welcome,".$username;.Sessionsareserver-si
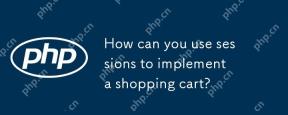
The steps to build an efficient shopping cart system using sessions include: 1) Understand the definition and function of the session. The session is a server-side storage mechanism used to maintain user status across requests; 2) Implement basic session management, such as adding products to the shopping cart; 3) Expand to advanced usage, supporting product quantity management and deletion; 4) Optimize performance and security, by persisting session data and using secure session identifiers.
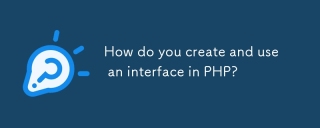
The article explains how to create, implement, and use interfaces in PHP, focusing on their benefits for code organization and maintainability.
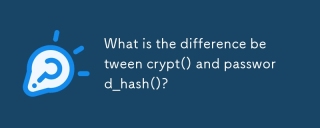
The article discusses the differences between crypt() and password_hash() in PHP for password hashing, focusing on their implementation, security, and suitability for modern web applications.
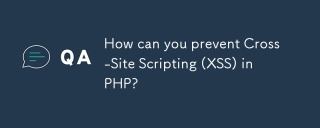
Article discusses preventing Cross-Site Scripting (XSS) in PHP through input validation, output encoding, and using tools like OWASP ESAPI and HTML Purifier.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
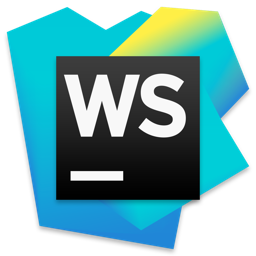
WebStorm Mac version
Useful JavaScript development tools
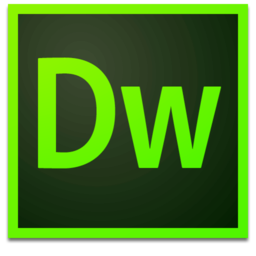
Dreamweaver Mac version
Visual web development tools
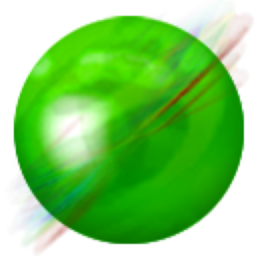
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
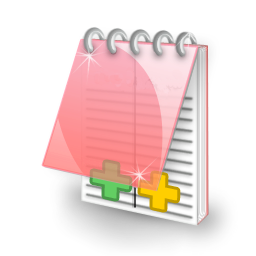
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
