With the continuous development of the Internet and mobile devices, message queues have become an indispensable part of the modern Internet architecture. Message Queuing (MQ) can deliver messages between different applications and achieve decoupling and asynchronous processing in distributed systems, thereby improving the scalability and performance of the entire system. Among message queues, Kafka is a very popular and powerful open source message middleware, while Swoole is a PHP-based asynchronous and coroutine network programming framework that can greatly improve the performance and concurrency of PHP applications.
This article will introduce how to use Swoole and Kafka to build a high-performance MQ system in PHP applications. We will explore the integration of Swoole and Kafka and how they can be used to improve the performance and reliability of your MQ system.
1. Overview of Swoole Framework
Swoole is an asynchronous, event-driven and coroutine network programming framework based on PHP. It provides a set of high-performance, highly scalable and high-concurrency network programming components, including TCP/UDP server and client, HTTP server and client, WebSocket server and client, and powerful asynchronous MySQL client. Swoole's coroutine mechanism can greatly improve the concurrency and performance of PHP applications.
Swoole provides a set of powerful asynchronous programming APIs, including event loops, asynchronous I/O, timers, signal processing, etc. Developers can easily build high-performance web applications using these APIs. In addition, Swoole also integrates a coroutine scheduler, which can combine asynchronous I/O and coroutines to achieve efficient concurrent programming. Compared with the traditional PHP multi-process model, Swoole's coroutine model can greatly reduce thread switching and congestion, improving application performance and throughput.
2. Overview of Kafka message middleware
Kafka is a high-performance, distributed, and persistent message middleware. It can handle high-throughput messages and data flows, supporting large-scale message transmission and storage. Kafka uses a distributed message transmission and storage method and can be easily expanded to hundreds of servers to achieve high availability and distributed message processing. In addition, Kafka also supports persistent storage of messages, ensuring the reliability of message processing.
Kafka provides a set of powerful APIs, including Producer API, Consumer API and Streams API. Developers can use these APIs to easily build distributed message processing systems that support multiple message formats and protocols. Kafka also integrates monitoring and management tools to monitor, manage and optimize message flows, improving the performance and reliability of the entire system.
3. Integration of Swoole and Kafka
Swoole and Kafka can be well integrated to build a high-performance MQ system. Swoole provides a powerful asynchronous programming API to easily communicate and interact with Kafka. Developers can use Swoole's TCP/UDP client and Kafka's Producer API and Consumer API to build asynchronous message processing processes.
The following is a sample code for building an MQ system using Swoole and Kafka:
<?php use KafkaProducer; use SwooleCoroutineHttpClient; // 初始化Kafka Producer $brokers = 'localhost:9092'; $producer = new Producer(); $producer->setBrokers([$brokers]); // 初始化Swoole TCP客户端 $client = new Client('localhost', 9501); // 接收请求并发送消息到Kafka $client->on('receive', function($cli, $data) use($producer) { $topic = 'test'; $message = $data; $producer->send([$topic => [$message]]); }); // 监听TCP连接 $client->on('connect', function($cli) { echo "Connected "; }); $client->connect(); // 初始化Kafka Consumer $consumer = new KafkaConsumer(); $consumer->setBrokers([$brokers]); // 订阅Kafka消息 $consumer->subscribe(['test']); // 处理Kafka消息 while (true) { $message = $consumer->consume(1); if ($message) { $data = $message['test'][0]['message']['value']; echo "Received message: {$data} "; } }
In the above code, we first initialize the Kafka Producer and Consumer. We then use Swoole's TCP client to listen on the port, receive requests and send messages to the Kafka Producer. After the message is sent successfully, we use Kafka Consumer to subscribe to the message and process the received message in a loop.
The benefits of using Swoole and Kafka to build a high-performance MQ system are obvious. First, Swoole provides asynchronous and coroutine support, which can improve application performance and concurrency capabilities. Secondly, Kafka is a high-performance and scalable message middleware that can handle high-throughput messages and data streams. Finally, the integration of Swoole and Kafka can improve the reliability and maintainability of the MQ system, providing better user experience and service quality.
Conclusion
This article introduces how to use Swoole and Kafka to build a high-performance MQ system. We explored Swoole's asynchronous/coroutine programming model and Kafka's distributed message transmission and storage features. We also provide a sample code for building an MQ system using Swoole and Kafka, demonstrating the process of asynchronous message processing. By using Swoole and Kafka, developers can build high-performance, highly reliable, and highly scalable MQ systems to provide users with better service experience and quality.
The above is the detailed content of Integration of Swoole and Kafka: Building a high-performance MQ system. For more information, please follow other related articles on the PHP Chinese website!
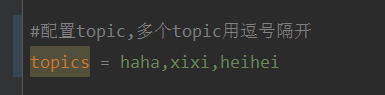
说明本项目为springboot+kafak的整合项目,故其用了springboot中对kafak的消费注解@KafkaListener首先,application.properties中配置用逗号隔开的多个topic。方法:利用Spring的SpEl表达式,将topics配置为:@KafkaListener(topics=“#{’${topics}’.split(’,’)}”)运行程序,console打印的效果如下
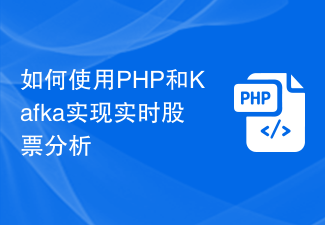
随着互联网和科技的发展,数字化投资已成为人们越来越关注的话题。很多投资者不断探索和研究投资策略,希望能够获得更高的投资回报率。股票交易中,实时的股票分析对决策非常重要,其中使用Kafka实时消息队列和PHP技术实现更是一种高效且实用的手段。一、Kafka介绍Kafka是由LinkedIn公司开发的一个高吞吐量的分布式发布、订阅消息系统。Kafka的主要特点是
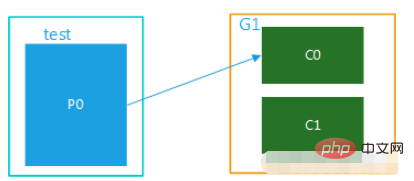
spring-kafka是基于java版的kafkaclient与spring的集成,提供了KafkaTemplate,封装了各种方法,方便操作,它封装了apache的kafka-client,不需要再导入client依赖org.springframework.kafkaspring-kafkaYML配置kafka:#bootstrap-servers:server1:9092,server2:9093#kafka开发地址,#生产者配置producer:#Kafka提供的序列化和反序列化类key
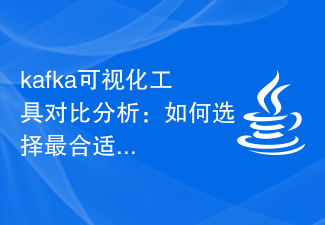
如何选择合适的Kafka可视化工具?五款工具对比分析引言:Kafka是一种高性能、高吞吐量的分布式消息队列系统,被广泛应用于大数据领域。随着Kafka的流行,越来越多的企业和开发者需要一个可视化工具来方便地监控和管理Kafka集群。本文将介绍五款常用的Kafka可视化工具,并对比它们的特点和功能,帮助读者选择适合自己需求的工具。一、KafkaManager
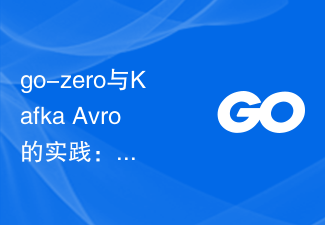
近年来,随着大数据的兴起和活跃的开源社区,越来越多的企业开始寻找高性能的交互式数据处理系统来满足日益增长的数据需求。在这场技术升级的浪潮中,go-zero和Kafka+Avro被越来越多的企业所关注和采用。go-zero是一款基于Golang语言开发的微服务框架,具有高性能、易用、易扩展、易维护等特点,旨在帮助企业快速构建高效的微服务应用系统。它的快速成长得
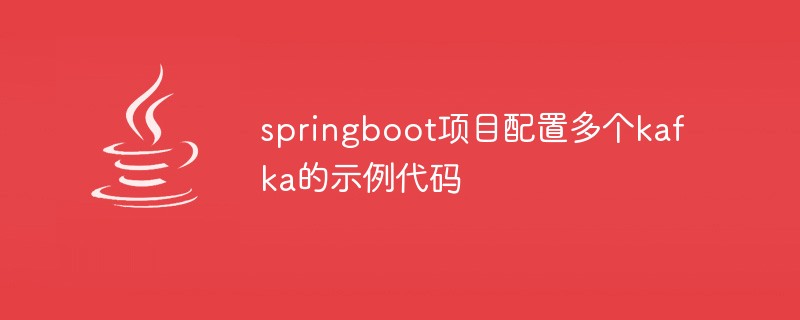
1.spring-kafkaorg.springframework.kafkaspring-kafka1.3.5.RELEASE2.配置文件相关信息kafka.bootstrap-servers=localhost:9092kafka.consumer.group.id=20230321#可以并发消费的线程数(通常与partition数量一致)kafka.consumer.concurrency=10kafka.consumer.enable.auto.commit=falsekafka.boo
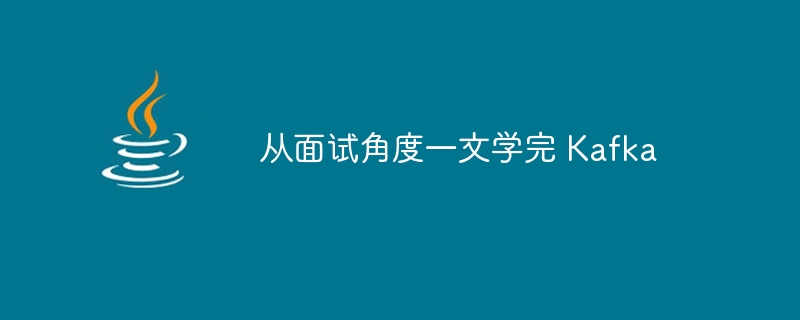
Kafka 是一个优秀的分布式消息中间件,许多系统中都会使用到 Kafka 来做消息通信。对分布式消息系统的了解和使用几乎成为一个后台开发人员必备的技能。
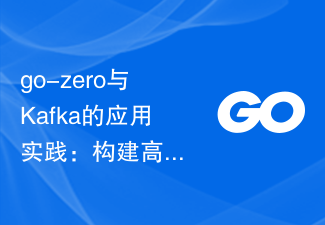
随着互联网的不断发展,对于消息系统的需求也越来越高。在构建高并发、高可靠性的消息系统中,go-zero和Kafka是两个非常好的选择。go-zero是一个基于Go语言的微服务框架,通过简单易用、高性能、可扩展等特点,在很多领域被广泛应用。Kafka是一个开源的分布式流媒体平台,具有高可靠性、高吞吐量、易拓展等特点,在处理大规模数据流和实时数据管道方面得到广泛


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
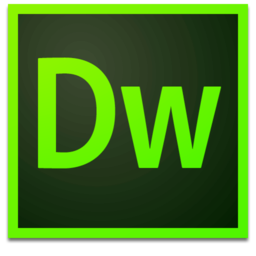
Dreamweaver Mac version
Visual web development tools
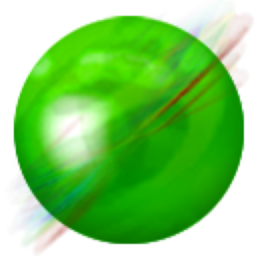
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
