Swoole is a high-performance asynchronous network-oriented programming framework based on PHP. It can realize asynchronous IO, multi-process multi-threading, coroutine and other features, and can greatly improve the performance of PHP in network programming. In many real-time and high-concurrency application scenarios, Swoole has become the first choice for developers. This article will introduce how to use Swoole to implement high-concurrency large file uploads.
1. Problems with traditional solutions
In traditional file upload solutions, the HTTP POST request method is usually used, that is, the file data is submitted through the form, and then the backend receives the request. Then upload by reading the file data. This method is sufficient when processing small files, but many problems will occur when processing large files:
- The process takes time
When the file is uploaded During the process, the data of the entire file needs to be read into the memory before uploading. When the transferred files are relatively large, the reading time will be very long, and PHP is a single process. When there are a large number of file upload requests, the service process will be blocked and the performance of the entire server will be affected.
- Memory usage
Since the entire file data needs to be read into memory for uploading, a large amount of server memory will be occupied, further affecting performance.
- Long response time
Since the entire file data needs to be read and uploaded before a response is returned, the response time will be very long, resulting in poor user experience. good.
2. Large file upload solution based on Swoole
- Principle introduction
Swoole can handle network requests in two ways: HTTP server and TCP server. The former is more suitable for web applications, while the latter is used for various custom network applications and protocols. In this article, we use HTTP server to implement large file upload scenario. Swoole provides two built-in objects, swoole_http_request and swoole_http_response, through which information about HTTP requests and responses can be obtained.
- Specific implementation
a. Client request
The client uploads the file data to the server through a POST request, and the server obtains the uploaded data through the swoole_http_request object File data.
b. Server-side processing
For each file request on the server side, we can obtain the file upload information through the swoole_http_request object, including file name, file type, file size, etc. Afterwards, the file can be uploaded through the asynchronous coroutine provided by Swoole, and the file can be read in chunks and transferred to the target server (such as Alibaba Cloud Object Storage OSS). What needs to be noted when uploading files is that you can use the coroutine method provided by Swoole for streaming data transmission, which can ensure that the memory footprint is relatively small.
c. Server response
After the file upload is completed, the server needs to give the client a successful upload and uploaded file information. Since Swoole provides the swoole_http_response object to directly respond to http requests, we can directly use it to respond to the client.
3. Code Example
The following is a simple example code for a large file upload solution based on Swoole.
<?php use SwooleHttpRequest; use SwooleHttpResponse; $http = new SwooleHttpServer("127.0.0.1", 9501); $http->on("request", function(Request $request, Response $response) { $filename = $request->files['file']['name']; $filepath = '/path/to/your/file' . $filename; $filesize = $request->header['content-length']; $tempPath = $request->files['file']['tmp_name']; $filetype = $request->files['file']['type']; $response->header("Content-Type", "application/json"); $response->header("Access-Control-Allow-Origin", "*"); $fp = fopen($tempPath, 'r'); $client = new SwooleCoroutineClient(SWOOLE_SOCK_TCP); $client->connect('your-oss-cn-addr', 'your-oss-cn-port'); $client->send("your-key"); $client->send("your-secret"); $client->send($filename); $client->send($filesize); $client->send($filetype); while (!feof($fp)) { $client->send(fread($fp, 8192)); } fclose($fp); $client->close(); $response->end(json_encode([ 'success' => true, 'message' => '文件上传成功' ])); }); $http->start();
4. Notes
- Start the PHP extension
Using Swoole requires starting the corresponding PHP extension, which can be installed through the following command:
pecl install swoole
- Configuring the Swoole server
When using Swoole to upload files, you need to configure the relevant parameters of the Swoole server. For example, you need to set the number of worker processes, the level of log information recording, the port number, etc., which can be set according to specific needs. In the above sample code, we used the following code for configuration:
$http = new SwooleHttpServer("127.0.0.1", 9501);
- Memory usage
When uploading a file, the uploaded data needs to be cached and processed. Therefore, a large amount of memory may be used when processing file uploads. In order to avoid memory overflow problems, you can consider reading the file in chunks, transmitting each piece of data after reading it, and then reading the next piece of data after the transmission is completed.
5. Summary
This article introduces how to use Swoole to achieve high-concurrency uploading of large files. Compared with the traditional file upload method, using Swoole can greatly improve the efficiency of file upload and improve the performance of the server. In actual applications, the appropriate upload scheme and Swoole parameter configuration can be selected according to specific needs.
The above is the detailed content of Swoole implements high-concurrency large file upload solution. For more information, please follow other related articles on the PHP Chinese website!
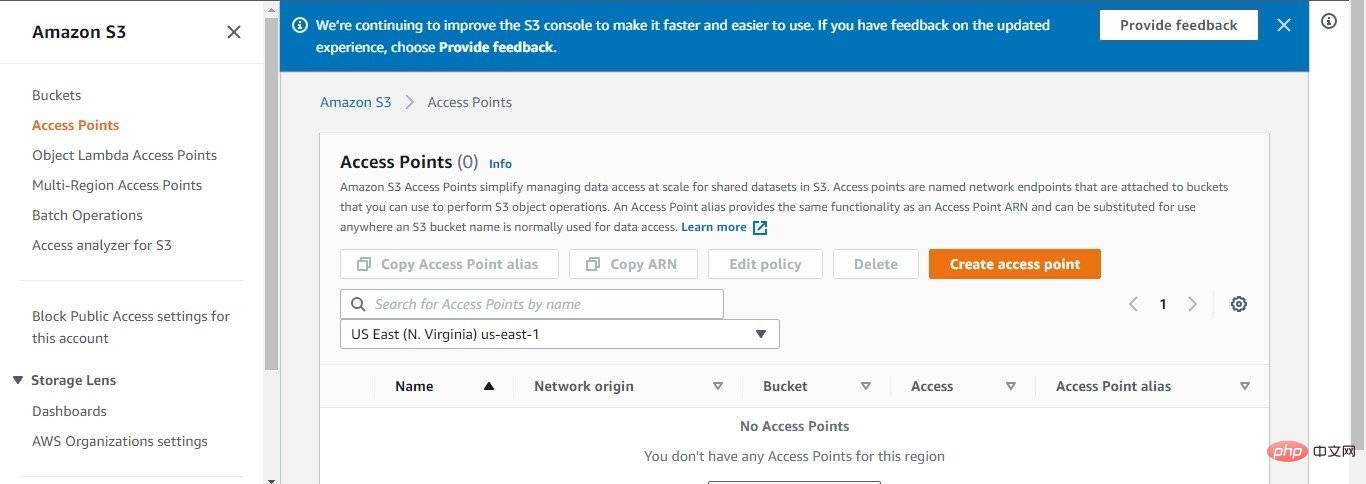
Amazon Simple Storage Service,简称Amazon S3,是一种使用 Web 界面提供存储对象的存储服务。Amazon S3 存储对象可以存储不同类型和大小的数据,从应用程序到数据存档、备份、云存储、灾难恢复等等。该服务具有可扩展性,用户只需为存储空间付费。Amazon S3 有四个基于可用性、性能率和持久性的存储类别。这些类包括 Amazon S3 Standard、Amazon S3 Standard Infrequent Access、Amazon S3 One
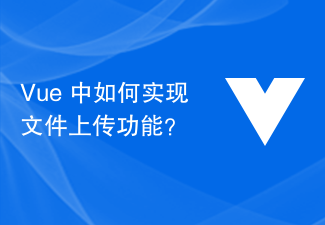
Vue作为目前前端开发最流行的框架之一,其实现文件上传功能的方式也十分简单优雅。本文将为大家介绍在Vue中如何实现文件上传功能。HTML部分在HTML文件中添加如下代码,创建上传表单:<template><div><formref="uploadForm"enc
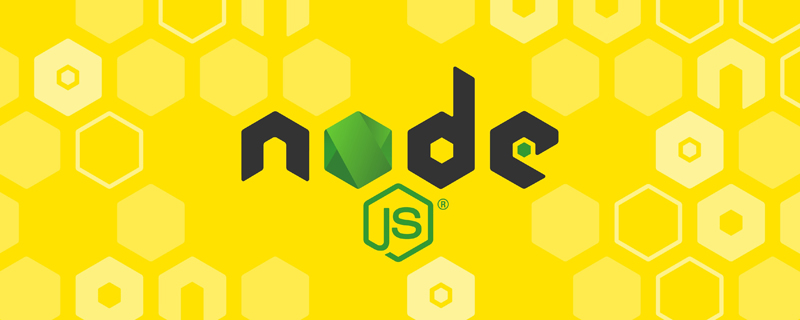
怎么处理文件上传?下面本篇文章给大家介绍一下node项目中如何使用express来处理文件的上传,希望对大家有所帮助!
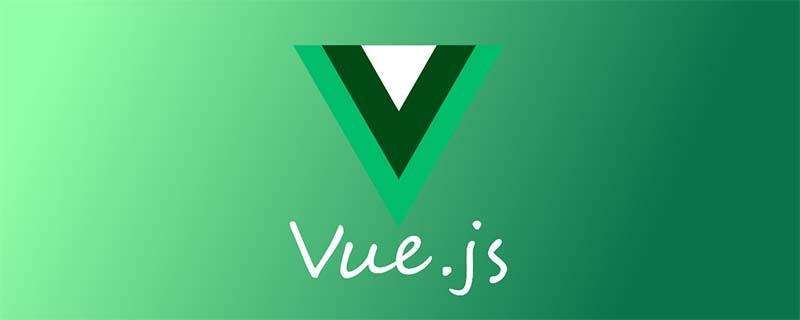
在实际开发项目过程中有时候需要上传比较大的文件,然后呢,上传的时候相对来说就会慢一些,so,后台可能会要求前端进行文件切片上传,很简单哈,就是把比如说1个G的文件流切割成若干个小的文件流,然后分别请求接口传递这个小的文件流。
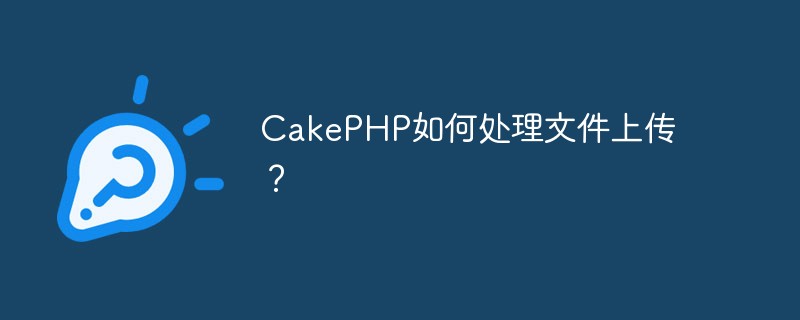
CakePHP是一个开源的Web应用程序框架,它基于PHP语言构建,可以简化Web应用程序的开发过程。在CakePHP中,处理文件上传是一个常见的需求,无论是上传头像、图片还是文档,都需要在程序中实现相应的功能。本文将介绍CakePHP中如何处理文件上传的方法和一些注意事项。在Controller中处理上传文件在CakePHP中,上传文件的处理通常在Cont
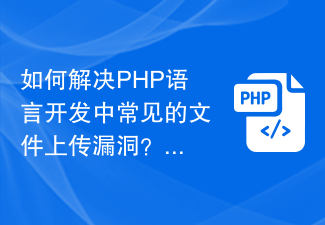
在Web应用程序的开发中,文件上传功能已经成为了基本的需求。这个功能允许用户向服务器上传自己的文件,然后在服务器上进行存储或处理。然而,这个功能也使得开发者更需要注意一个安全漏洞:文件上传漏洞。攻击者可以通过上传恶意文件来攻击服务器,从而导致服务器遭受不同程度的破坏。PHP语言作为广泛应用于Web开发中的语言之一,文件上传漏洞也是常见的安全问题之一。本文将介
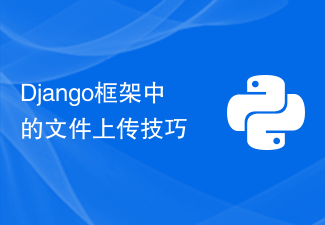
近年来,Web应用程序逐渐流行,而其中许多应用程序都需要文件上传功能。在Django框架中,实现上传文件功能并不困难,但是在实际开发中,我们还需要处理上传的文件,其他操作包括更改文件名、限制文件大小等问题。本文将分享一些Django框架中的文件上传技巧。一、配置文件上传项在Django项目中,要配置文件上传需要在settings.py文件中进
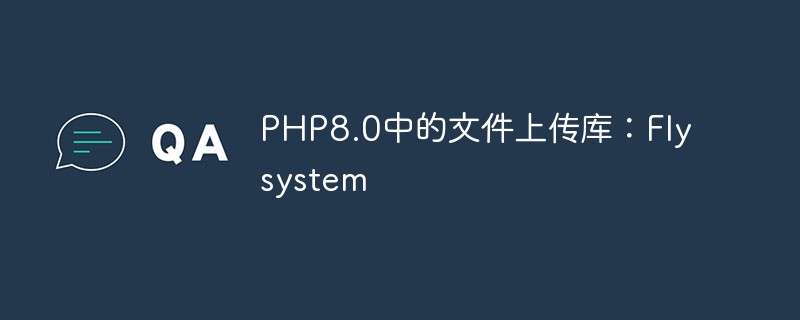
随着互联网的发展和普及,文件上传功能已经成为现代网站开发的必备功能之一。不论是网盘还是社交平台,文件上传都是必不可少的一环。而在PHP领域,由于其广泛的应用和易用性,文件上传的需求也非常常见。在PHP8.0中,一个名为Flysystem的文件上传库正式出现,它为PHP开发人员提供了更加高效、灵活且易于使用的文件上传和管理解决方案。Flysystem是一个轻量


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
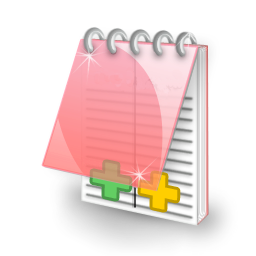
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool