Basic crawler tutorial: Implement basic web crawler using PHP
With the continuous development and progress of Internet technology, people have more and more ways to obtain information. As one of the tools, web crawlers play an increasingly important role in helping people obtain information. A web crawler is an automated program that collects, obtains, analyzes and stores data from web pages on the Internet.
The implementation of web crawlers can be carried out through a variety of programming languages. Among them, PHP language is a language widely used in the field of network development. It has the characteristics of easy to learn, easy to use, and high development efficiency. Therefore, this article will use PHP language As an example, we will introduce how to use PHP to implement a basic web crawler.
1. Overview
You need to understand the following points when starting to learn PHP web crawlers:
1. The basic working principle of web crawlers: web crawlers request pages from the network information, obtain the response, and parse the response data for data capture.
2. Web crawler crawling process: The crawler needs to collect URLs to build a crawler queue, request URLs to obtain HTML pages, parse data in HTML pages, and store data.
3. Web crawler parsing method: After the web crawler obtains the HTML page, it needs to parse the data and store the data. The parsing methods include regular expressions, DOM, XPath, etc.
2. Build a crawler queue
The first step to implement a crawler is to build a crawler queue, that is, construct a list of URLs to be crawled. In PHP, we can use arrays to store these URLs, and then traverse the array to make requests to these URLs. For example:
$url_list = array( 'https://www.example.com/page1.html', 'https://www.example.com/page2.html', 'https://www.example.com/page3.html' ); foreach($url_list as $url){ //请求该URL并进行解析数据的操作 }
3. Request the URL to get the HTML page
In PHP, we can use the cURL extension module to send HTTP requests. cURL is a client-side URL transfer library that supports multiple protocols, allowing PHP web scripts to send files and data to other servers. cURL provides several methods to simulate browser access. Commonly used request methods are GET, POST, PUT, COOKIE and other request methods.
The following is a sample code for using cURL to request a URL:
//初始化cURL $ch = curl_init(); //设置URL和其他请求选项 curl_setopt($ch, CURLOPT_URL, $url); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); //执行HTTP请求 $result = curl_exec($ch); //关闭cURL资源 curl_close($ch);
In this code, we first use the curl_init() function to initialize cURL, and then use the curl_setopt() function to set the request options, where CURLOPT_URL Used to specify the URL of the request, CURLOPT_RETURNTRANSFER sets the request result to be returned as a string, and finally uses the curl_exec() function to make an HTTP request and obtain the request result. Use curl_close() function to close cURL resources.
4. Parse the data in the HTML page
After obtaining the HTML page, you need to extract useful information from it. There are many ways to parse HTML pages. Here we will parse them through DOM.
DOM parsing is a way of analyzing XML/HTML documents. In PHP, we can use the DOMDocument class to parse HTML pages. First, you need to instantiate the DOMDocument class, then use the loadHTML() method to load the HTML page into the parser, and finally use the getElementsByTagName() method to obtain the required element objects.
The following is a sample code for using DOM to parse an HTML page:
//实例化DOMDocument类 $dom = new DOMDocument(); //禁用HTML原始输入 $dom->preserveWhiteSpace = false; //载入HTML页面 $dom->loadHTML($result); //获取所需元素 $element = $dom->getElementsByTagName('div');
In this code, we first create a DOMDocument object, then use the preserveWhiteSpace attribute to remove whitespace characters in HTML, and then use loadHTML () method to load the HTML page, and finally use the getElementsByTagName() method to obtain the specified elements.
5. Store data
After extracting useful information, we need to store this information. In PHP, we can use MySQL database for data storage.
First, you need to use the mysqli_connect() function to connect to the MySQL database. Then use the mysqli_query() function to execute SQL statements to insert data into the database.
The following is a sample code for using MySQL database to store data:
//连接到MySQL数据库 $con = mysqli_connect('localhost', 'root', '', 'test'); //将数据插入到数据库中 mysqli_query($con, "INSERT INTO test (name, age) VALUES ('Tom', 20)");
In this code, we first use the mysqli_connect() function to connect to the MySQL database, and then use the mysqli_query() function to test Insert data into the table.
6. Summary
This article introduces the basic process of using PHP to implement web crawlers, including building a crawler queue, requesting URLs to obtain HTML pages, parsing data in HTML pages, and storing data. At the same time, this article is only a preliminary learning guide. There are many factors that need to be considered in actual development, such as data cleaning, anti-crawler mechanisms, etc. However, I believe that this article can provide a preliminary understanding of the implementation of PHP web crawlers and lay the foundation for further learning.
The above is the detailed content of Basic crawler tutorial: Implement basic web crawler using PHP. For more information, please follow other related articles on the PHP Chinese website!
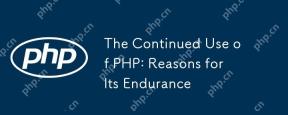
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
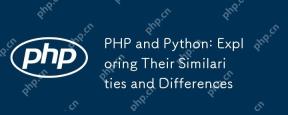
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
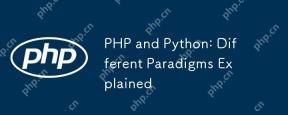
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
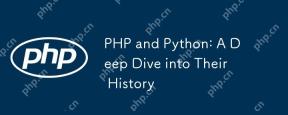
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
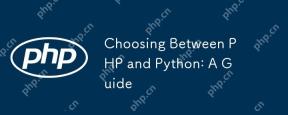
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
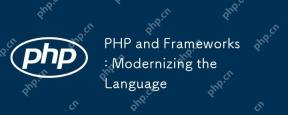
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
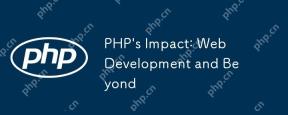
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
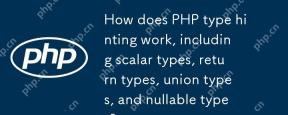
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use

Atom editor mac version download
The most popular open source editor
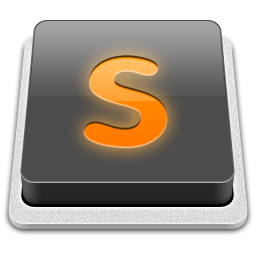
SublimeText3 Mac version
God-level code editing software (SublimeText3)