How to implement database migration in ThinkPHP6?
As applications continue to evolve and requirements continue to change, we often need to modify, migrate, and update the database during the development process. However, in the process of updating the database, if it is not carefully considered and maintained, a series of problems such as data conflicts and data loss may occur. In order to effectively solve these problems, we need to use a professional database migration tool to complete these operations.
ThinkPHP6 is a popular PHP framework for building web applications that provides many useful features and tools, including database migration. This article will introduce how to use the database migration function in ThinkPHP6.
1. What is database migration?
Database migration refers to the process of updating and modifying the database structure during the development and operation of applications. In other words, through database migration, we can add, delete, and rename database tables and add, modify, and delete fields.
In ThinkPHP6, the database migration tool is mainly implemented through two concepts: migration class and filling class.
2. How to use database migration?
- Create a migration class
To use database migration in ThinkPHP6, you first need to create a migration class. The migration class is a PHP class that contains two methods, the up method and the down method.
The up method is used to perform database migration operations, such as creating, modifying, deleting database tables or fields, etc. The down method is used to roll back the migration operation, that is, to undo the operation performed by the up method.
The following is a simple example migration class for creating a database table named users:
<?php use thinkmigrationdbColumn; use thinkmigrationMigrator; class CreateUsersTable extends Migrator { public function up() { $table = $this->table('users', ['engine' => 'InnoDB', 'id' => false, 'primary_key' => 'id']); $table->addColumn('id', 'integer', ['signed' => false, 'identity' => true]) ->addColumn('name', 'string', ['limit' => 32]) ->addColumn('email', 'string', ['limit' => 128]) ->addColumn('password', 'string', ['limit' => 60]) ->addColumn('created_at', 'datetime') ->addColumn('updated_at', 'datetime') ->create(); } public function down() { $this->table('users')->drop(); } }
In the above migration class, we first create a database table named users in the up method. Create a database table for users and add some fields. Then in the down method, we delete the table.
- Perform database migration
After creating the migration class, we need to use the php think migrate command to perform the migration operation. If no migration has been performed yet, we need to first use the php think migrate:install command to initialize the related tables for database migration.
Next, we can use the php think migrate:status command to view all current migration statuses. At this point, we can see that the migration class we created has not yet been executed.
Then, we can use the php think migrate:run command to perform the migration operation. At this point, we can see that the system will execute the migration class we just created and output some debugging information.
After execution, we can use the php think migrate:status command again to view all current migration statuses. At this point, we should be able to see that the migration class we created has been successfully executed and a table named users has been created in the database.
If we need to roll back the migration we just created, we can use the php think migrate:rollback command. This command will roll back the last migration operation performed.
In addition, we can also use the php think migrate:reset command to roll back all migration operations that have been performed.
3. How to use filler classes?
In addition to migration classes, ThinkPHP6 also provides a feature called filling classes. The fill class is used to add test data or default data to an already existing database table.
Similar to the migration class, the filling class is also a PHP class containing two methods, which are the up method and the down method.
Here is a simple example fill class to add some test data to the database table named users:
<?php use thinkmigrationSeed; class AddTestUsers extends Seed { public function run() { $data = [ [ 'name' => '张三', 'email' => 'zhangsan@test.com', 'password' => password_hash('123456', PASSWORD_DEFAULT), 'created_at' => date('Y-m-d H:i:s'), 'updated_at' => date('Y-m-d H:i:s'), ], [ 'name' => '李四', 'email' => 'lisi@test.com', 'password' => password_hash('123456', PASSWORD_DEFAULT), 'created_at' => date('Y-m-d H:i:s'), 'updated_at' => date('Y-m-d H:i:s'), ], [ 'name' => '王五', 'email' => 'wangwu@test.com', 'password' => password_hash('123456', PASSWORD_DEFAULT), 'created_at' => date('Y-m-d H:i:s'), 'updated_at' => date('Y-m-d H:i:s'), ], ]; $this->table('users')->insert($data)->save(); } public function down() { $this->execute('TRUNCATE TABLE users;'); } }
In the above fill class, we use in the up method The insert method adds three pieces of test data to the database table. Then in the down method, we use the execute method to delete these three test data.
The method of executing the filling class is similar to the method of executing the migration class. We can use the php think seed:run command to execute the up method of the filling class. If you need to roll back the filling class task, you can use the php think seed:rollback command.
4. Summary
This article introduces the method of using database migration and filling in ThinkPHP6, and demonstrates through sample code how to create migration classes and filling classes, and how to execute and rollback migrations. and filling operations. If you are developing and maintaining an application and need to modify and update the database, please be sure to use this professional database migration tool to ensure the security and correctness of your data.
The above is the detailed content of How to implement database migration in ThinkPHP6?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use

Atom editor mac version download
The most popular open source editor
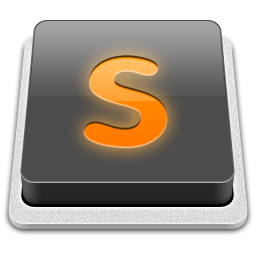
SublimeText3 Mac version
God-level code editing software (SublimeText3)