Getting Started Guide to PHP Exception Handling
In the programming process, exception handling has always been an important topic. The same is true for exception handling in the PHP language. An exception refers to an error or problem during the running of a program. It reminds the programmer of some details that need to be paid attention to in the program. This article aims to introduce the basic concepts of PHP exception handling and how to use exception handling in PHP.
1. What is exception handling
Exception handling refers to the process of catching errors, interruptions or unknown states that may occur during program execution and processing them. Unlike traditional error handling (using return values or similar mechanisms), exception handling uses exception (Exception) objects for identification and delivery.
In PHP, exception handling can be used to handle runtime errors, syntax errors, database operation errors, etc.
2. Exception handling in PHP
In PHP, exception handling consists of two parts: exception throwing and exception catching. The throwing of an exception is to create an Exception object and throw it when an exception is encountered in the program. The engine will terminate the execution of the current code block and immediately jump to the nearest exception handler. Exception catching is to catch the thrown exception through try-catch statement block and handle it.
The following is an example of throwing and catching exceptions:
function divide($dividend, $divisor) { if($divisor == 0) { throw new Exception('Division by zero.'); } return $dividend / $divisor; } try { $result = divide(100, 0); } catch(Exception $e) { echo 'Caught exception: ', $e->getMessage(), " "; }
In the above code, the function divide
determines that if $divisor is equal to 0, an Exception object. The try-catch statement is used to catch this exception and output error information.
3. Custom exceptions
In PHP, you can create custom exceptions by inheriting the Exception class. By customizing exceptions, we can throw different exception objects according to different situations, thereby improving the accuracy and readability of exception handling.
The following is an example of a custom exception:
class UserNotFoundException extends Exception { public function errorMessage() { // 定义错误信息 $errorMsg = 'Error on line '.$this->getLine().' in '.$this->getFile() .': <b>'.$this->getMessage().'</b> is not a valid E-Mail address'; return $errorMsg; } } $email = "john.doe@example.com"; try { if(filter_var($email, FILTER_VALIDATE_EMAIL) === false) { // 抛出自定义异常 throw new UserNotFoundException($email); } } catch (UserNotFoundException $e) { // 处理自定义异常 echo $e->errorMessage(); }
In the above code, a UserNotFoundException exception is customized, which inherits the Exception class and overrides the errorMessage method to return a custom error message. In the try-catch statement, the filter_var function is used to determine whether $email is a valid email address. If not, a custom exception is thrown.
4. Best Practices for Exception Handling
When using exception handling, the following are some best practices:
- When an exception is thrown, try to provide clarity and clarity error message.
- Use try-catch statement blocks to wrap code blocks where exceptions may occur, and handle exceptions in the catch block.
- Avoid throwing new exceptions in catch blocks, which can lead to confusion and more complicated exception handling flow.
- Use different types of exceptions when throwing multiple exceptions so that they can be better distinguished when handling them.
In short, in PHP, exception handling is a way to achieve reliable error handling. It makes it easier for programmers to identify and handle exceptions, and makes code easier to maintain and extend. Mastering the basic concepts and best practices of PHP exception handling is one of the essential skills for every PHP developer.
The above is the detailed content of Beginner's Guide to PHP Exception Handling. For more information, please follow other related articles on the PHP Chinese website!
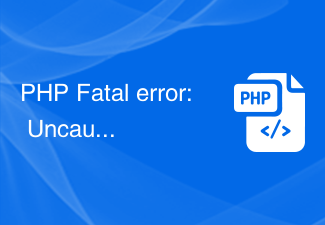
PHP是一种广泛使用的服务器端编程语言,它可以为网站提供强大的动态功能。但是,在实践中,开发人员可能会遇到各种各样的错误和异常。其中一个常见的错误是PHPFatalerror:Uncaughtexception'Exception'。在本文中,我们将探讨这个错误的原因以及如何解决它。异常的概念在PHP中,异常是指程序在运行过程中遇到的意外情况,导致
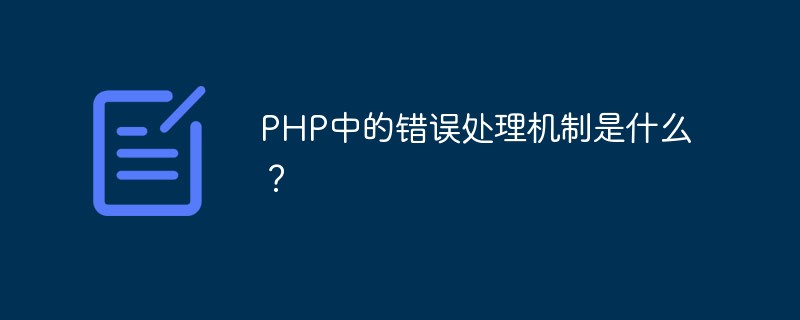
PHP是一种流行而强大的服务器端编程语言,可以用来开发各种Web应用程序。就像其他编程语言一样,PHP也有可能会出现错误和异常。这些错误和异常可能由各种原因引起,如程序错误、服务器错误、用户输入错误等等。为了确保程序的运行稳定性和可靠性,PHP提供了一套完整的错误处理机制。PHP错误处理机制的基本思想是:当发生错误时,程序会停止执行并输出一条错误消息。我们可
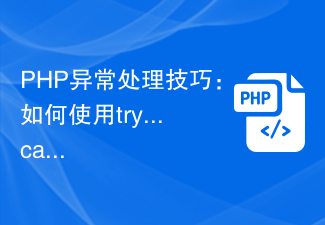
PHP异常处理技巧:如何使用try...catch块捕获和处理多个异常引言:在PHP应用程序开发中,异常处理是非常重要的一环。当代码中发生错误或异常时,合理的异常处理能够提高程序的健壮性和可靠性。本文将介绍如何使用try...catch块捕获和处理多个异常,帮助开发者进行更加灵活和高效的异常处理。异常处理介绍异常是指在程序运行时产生的错误或特殊情况。当异常出
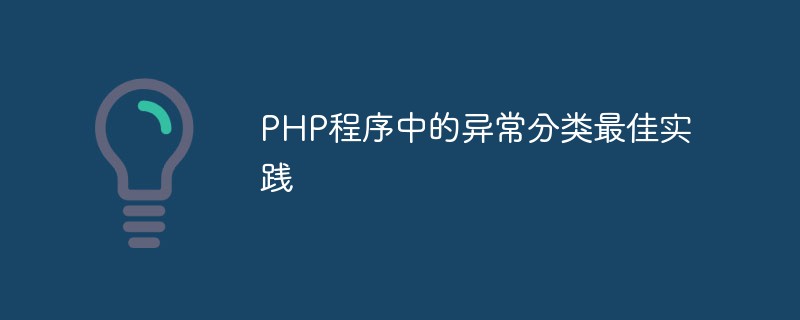
在编写PHP代码时,异常处理是不可或缺的一部分,它可以使代码更加健壮和可维护。但是,异常处理也需要谨慎使用,否则就可能带来更多的问题。在这篇文章中,我将分享一些PHP程序中异常分类的最佳实践,以帮助你更好地利用异常处理来提高代码质量。异常的概念在PHP中,异常是指在程序运行时发生的错误或意外情况。通常情况下,异常会导致程序停止运行并输出异常信息。
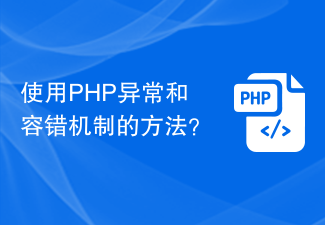
如何使用PHP的异常处理和容错机制?引言:在PHP编程中,异常处理和容错机制是非常重要的。当代码执行过程中出现错误或异常的时候,可以使用异常处理来捕获和处理这些错误,以保证程序的稳定性和可靠性。本文将介绍如何使用PHP的异常处理和容错机制。一、异常处理基础知识:什么是异常?异常是在代码执行过程中出现的错误或异常情况,包括语法错误、运行时错误、逻辑错误等。当异
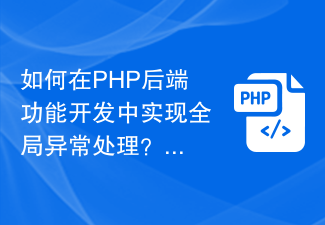
如何在PHP后端功能开发中实现全局异常处理?在PHP后端开发中,异常处理是非常重要的一环。它可以帮助我们捕获程序中的错误,并进行适当的处理,从而提高系统的稳定性和性能。本文将介绍如何在PHP后端功能开发中实现全局异常处理,并提供相应的代码示例。PHP提供了异常处理的机制,我们可以通过try和catch关键字来捕获异常并进行相应的处理。全局异常处理指的是将所有
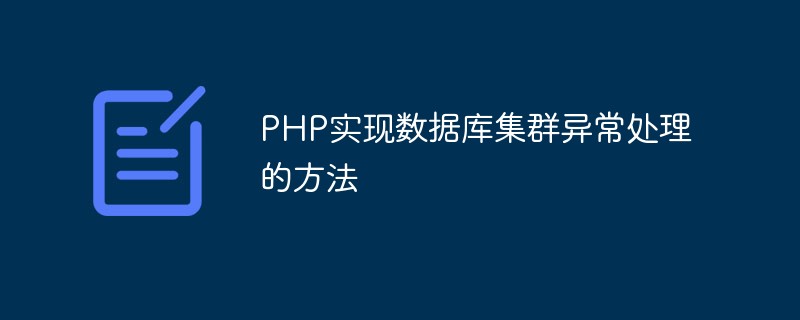
随着互联网的不断发展,越来越多的企业和组织开始规划数据库集群来满足其数据处理需求。数据库集群可能包含数百甚至数千个节点,因此在节点之间确保数据同步和协调非常重要。在该环境下,存在着很多的异常情况,如单节点故障,网络分区,数据同步错误等,并且需要实现实时检测和处理。本文将介绍如何使用PHP实现数据库集群异常处理。数据库集群的概述在数据库集群中,一个单独的
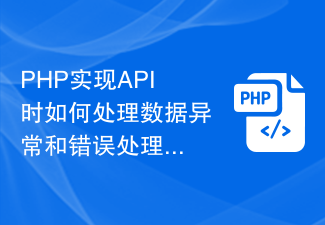
随着API的使用越来越广泛,我们在开发和使用API过程中也需要考虑到数据异常和错误处理的策略。本文将探讨PHP实现API时如何处理这些问题。一、处理数据异常数据异常出现的原因可能有很多,比如用户输入错误、网络传输错误、服务器内部错误等等。在PHP开发时,我们可以使用以下方法来处理数据异常。返回合适的HTTP状态码HTTP协议定义了很多状态码,可以帮助我们在处


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
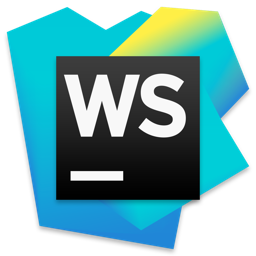
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
