How to use mixins to implement component code reuse in Vue
How to use mixins to achieve component code reuse in Vue
As applications become more and more complex, we need more componentization and code reuse to improve development efficiency. In Vue, mixin is a very simple and very useful tool that can help us reuse component code.
Mixins are a mixin-like concept that allow the same code to be shared between multiple components. In Vue, we can think of a mixin as an object that contains some reusable properties and methods that can be referenced by multiple components.
The following is an example of using mixin to achieve component code reuse:
// 定义一个mixin const myMixin = { data() { return { count: 0 } }, methods: { increment() { this.count++ } } } // 定义一个组件 Vue.component('my-component', { mixins: [myMixin], template: `<div> <p>Count: {{ count }}</p> <button @click="increment">Increment</button> </div>` }) // 创建Vue实例 new Vue({ el: '#app' })
In the above example, we first define a mixin object named myMixin, which contains a count data and a method called increment. Next, we defined a component named my-component and used the mixins attribute in the component to pass the myMixin object as a parameter, so that the components can share the properties and methods in myMixin.
Finally, we create a Vue instance and mount it on the DOM element with the id app.
When we use the my-component component in the page, we will find that it not only contains its own template code, but also inherits the count data and increment method in myMixin. This way we can reuse the same code across multiple components without having to write the same code for each component.
In addition to using the mixins attribute in the component to reference the mixin as above, we can also use the Vue.mixin() method to register the mixin in the global scope. This way, all components have access to the same mixin and share its properties and methods.
The benefits of using mixins are obvious. It allows us to share code between multiple components, thereby improving code reusability and maintainability. When we need to update some code, we only need to update it in the mixin, so that all components that reference it will be updated automatically. This greatly improves development efficiency and code quality.
Of course, there are some things to pay attention to when using mixin. First, we need to avoid defining the same properties and methods in components and mixins at the same time, as this can lead to naming conflicts and unpredictable behavior. Secondly, we need to ensure that when using mixins, there will be no interaction between components, such as different components using the same data source or method name.
In short, mixin is a very powerful and simple tool in Vue. It can help us reuse and share component code, thereby improving development efficiency and code quality. As applications become more and more complex, using mixins becomes an integral part of our lives.
The above is the detailed content of How to use mixins to implement component code reuse in Vue. For more information, please follow other related articles on the PHP Chinese website!

Netflix uses React as its front-end framework. 1) React's componentized development model and strong ecosystem are the main reasons why Netflix chose it. 2) Through componentization, Netflix splits complex interfaces into manageable chunks such as video players, recommendation lists and user comments. 3) React's virtual DOM and component life cycle optimizes rendering efficiency and user interaction management.

Netflix's choice in front-end technology mainly focuses on three aspects: performance optimization, scalability and user experience. 1. Performance optimization: Netflix chose React as the main framework and developed tools such as SpeedCurve and Boomerang to monitor and optimize the user experience. 2. Scalability: They adopt a micro front-end architecture, splitting applications into independent modules, improving development efficiency and system scalability. 3. User experience: Netflix uses the Material-UI component library to continuously optimize the interface through A/B testing and user feedback to ensure consistency and aesthetics.

Netflixusesacustomframeworkcalled"Gibbon"builtonReact,notReactorVuedirectly.1)TeamExperience:Choosebasedonfamiliarity.2)ProjectComplexity:Vueforsimplerprojects,Reactforcomplexones.3)CustomizationNeeds:Reactoffersmoreflexibility.4)Ecosystema

Netflix mainly considers performance, scalability, development efficiency, ecosystem, technical debt and maintenance costs in framework selection. 1. Performance and scalability: Java and SpringBoot are selected to efficiently process massive data and high concurrent requests. 2. Development efficiency and ecosystem: Use React to improve front-end development efficiency and utilize its rich ecosystem. 3. Technical debt and maintenance costs: Choose Node.js to build microservices to reduce maintenance costs and technical debt.

Netflix mainly uses React as the front-end framework, supplemented by Vue for specific functions. 1) React's componentization and virtual DOM improve the performance and development efficiency of Netflix applications. 2) Vue is used in Netflix's internal tools and small projects, and its flexibility and ease of use are key.

Vue.js is a progressive JavaScript framework suitable for building complex user interfaces. 1) Its core concepts include responsive data, componentization and virtual DOM. 2) In practical applications, it can be demonstrated by building Todo applications and integrating VueRouter. 3) When debugging, it is recommended to use VueDevtools and console.log. 4) Performance optimization can be achieved through v-if/v-show, list rendering optimization, asynchronous loading of components, etc.

Vue.js is suitable for small to medium-sized projects, while React is more suitable for large and complex applications. 1. Vue.js' responsive system automatically updates the DOM through dependency tracking, making it easy to manage data changes. 2.React adopts a one-way data flow, and data flows from the parent component to the child component, providing a clear data flow and an easy-to-debug structure.

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
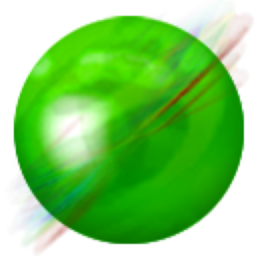
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
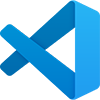
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft