Block storage is an emerging data storage method that has been widely used in blockchain technologies such as Bitcoin and Ethereum. As a commonly used back-end programming language, PHP has relatively powerful data processing capabilities and can also implement block storage functions. This article will introduce how to use PHP to implement block storage.
1. What is block storage
Block storage, also known as a distributed database, is a database composed of multiple nodes scattered on multiple computers. These nodes can be connected together through networks such as the Internet and collaborate with each other to complete data storage and sharing. The reason why block storage is more secure and non-tamperable than traditional databases is that it uses hash chain technology to ensure data integrity and reliability.
2. Steps to implement block storage in PHP
- Create a blockchain class
Define a class named BlockChain and set the following Properties: $chain, $pending_transactions and $mining_reward. Among them, $chain is a chain structure that stores all blocks; $pending_transactions stores transactions that will be packaged; $mining_reward is used to store mining rewards.
- Add genesis block
The genesis block is the first block of the blockchain and needs to be added manually. Define a method named create_genesis_block in the BlockChain class to create the genesis block.
- Add new block
Define a method named add_block in the BlockChain class to add a new block. The function of this method is to add new blocks to the blockchain and update all pending transactions. The code is as follows:
function add_block($new_block) { $new_block->previous_hash = $this->get_last_block()->hash; $new_block->mine_block($this->mining_reward); array_push($this->chain, $new_block); // Update pending transactions $this->pending_transactions = array(); $this->pending_transactions[] = new Transaction(null, $this->mining_reward); }
- Create block data structure
In the BlockChain class, define a class named Block, which includes attributes $index, $timestamp, $transactions , $previous_hash and $hash.
- Implement the mining algorithm
In the Block class, implement the mining algorithm to ensure the security and reliability of the blockchain. The main function of the mining algorithm is to calculate the hash value of the block and adjust the mining speed based on the difficulty coefficient and the number of transactions. The following is the implementation code of the mining algorithm:
function mine_block($mining_reward) { $this->timestamp = time(); $transaction = new Transaction(null, $mining_reward); array_push($this->transactions, $transaction); $this->hash = $this->calculate_hash(); while(substr($this->hash, 0, 4) !== "0000") { $this->nonce++; $this->hash = $this->calculate_hash(); } }
- Implementing transactions
In the Block class, define a class named Transaction, including $from_address, $to_address and $amount three properties. The core function of the transaction is to transfer assets, and its implementation code is as follows:
class Transaction { public $from_address; public $to_address; public $amount; public function __construct($from_address, $to_address, $amount) { $this->from_address = $from_address; $this->to_address = $to_address; $this->amount = $amount; } }
- Implement the hash algorithm
In the Block class, implement the hash algorithm to calculate the hash of the block Hope value. The hash algorithm can use SHA256, and the implementation code is as follows:
function calculate_hash() { return hash("sha256", $this->index . $this->previous_hash . $this->timestamp . json_encode($this->transactions) . $this->nonce); }
3. Example of using PHP to implement block storage
The following is a simple blockchain example that demonstrates how to Use PHP to implement block storage function:
index = $index; $this->timestamp = time(); $this->transactions = $transactions; $this->previous_hash = $previous_hash; $this->hash = $this->calculate_hash(); } function calculate_hash() { return hash("sha256", $this->index . $this->previous_hash . $this->timestamp . json_encode($this->transactions) . $this->nonce); } function mine_block($difficulty) { while(substr($this->hash, 0, $difficulty) !== str_repeat("0", $difficulty)) { $this->nonce++; $this->hash = $this->calculate_hash(); } echo "Block mined: " . $this->hash . " "; } } class BlockChain { public $chain; public $difficulty; public $pending_transactions; public $mining_reward; public function __construct() { $this->chain = array(); array_push($this->chain, $this->create_genesis_block()); $this->difficulty = 2; $this->pending_transactions = array(); $this->mining_reward = 100; } function create_genesis_block() { return new Block(0, array(), "0"); } function add_transaction($transaction) { array_push($this->pending_transactions, $transaction); } function get_last_block() { return $this->chain[sizeof($this->chain)-1]; } function mine_pending_transactions($mining_reward_address) { $block = new Block(sizeof($this->chain), $this->pending_transactions, $this->get_last_block()->hash); $block->mine_block($this->difficulty); echo "Block successfully mined! "; array_push($this->chain, $block); $this->pending_transactions = array(); $this->pending_transactions[] = new Transaction(null, $mining_reward_address, $this->mining_reward); } function get_balance($address) { $balance = 0; foreach($this->chain as $block) { foreach($block->transactions as $transaction) { if($transaction->from_address === $address) { $balance -= $transaction->amount; } if($transaction->to_address === $address) { $balance += $transaction->amount; } } } return $balance; } function is_chain_valid() { for($i = 1; $i < sizeof($this->chain); $i++) { $current_block = $this->chain[$i]; $previous_block = $this->chain[$i-1]; if($current_block->hash !== $current_block->calculate_hash() || $current_block->previous_hash !== $previous_block->hash) { return false; } return true; } } } class Transaction { public $from_address; public $to_address; public $amount; public function __construct($from_address, $to_address, $amount) { $this->from_address = $from_address; $this->to_address = $to_address; $this->amount = $amount; } } $blockchain = new BlockChain(); $blockchain->add_transaction(new Transaction("address1", "address2", 100)); $blockchain->add_transaction(new Transaction("address2", "address1", 50)); echo "Starting the miner... "; $blockchain->mine_pending_transactions("miner1"); echo "Balance of miner1 is " . $blockchain->get_balance("miner1") . " "; echo "Balance of address1 is " . $blockchain->get_balance("address1") . " "; echo "Balance of address2 is " . $blockchain->get_balance("address2") . " "; echo "Starting the miner again... "; $blockchain->mine_pending_transactions("miner2"); echo "Balance of miner1 is " . $blockchain->get_balance("miner1") . " "; echo "Balance of address1 is " . $blockchain->get_balance("address1") . " "; echo "Balance of address2 is " . $blockchain->get_balance("address2") . " "; ?>
4. Summary
This article introduces the method of using PHP to implement block storage, from creating a blockchain class, adding a genesis block, adding a new Blocks, creating block data structures, implementing mining algorithms, implementing transactions, etc. are explained in detail, and specific code implementations are given. I hope this article can help PHP programmers better understand the principles and implementation methods of block storage, and apply it to actual projects in practice.
The above is the detailed content of How to implement block storage using PHP. For more information, please follow other related articles on the PHP Chinese website!
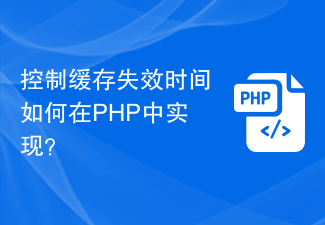
随着互联网应用的普及,网站响应速度越来越成为用户关注的重点。为了快速响应用户的请求,网站往往采用缓存技术缓存数据,从而减少数据库查询次数。但是,缓存的过期时间对响应速度有着重要影响。本文将对控制缓存失效时间的方法进行探讨,以帮助PHP开发者更好地应用缓存技术。一、什么是缓存失效时间?缓存失效时间是指缓存中的数据被认为已经过期的时间。它决定了缓存中的数据何时需
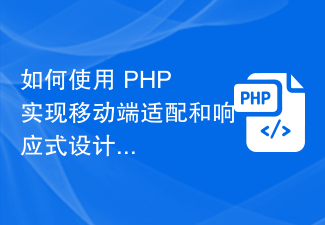
如何使用PHP实现移动端适配和响应式设计移动端适配和响应式设计是现代网站开发中重要的实践,它们能够保证网站在不同设备上的良好展示效果。在本文中,我们将介绍如何使用PHP实现移动端适配和响应式设计,并附带代码示例。一、理解移动端适配和响应式设计的概念移动端适配是指根据设备的不同特性和尺寸,针对不同的设备提供不同的样式和布局。而响应式设计则是指通过使用
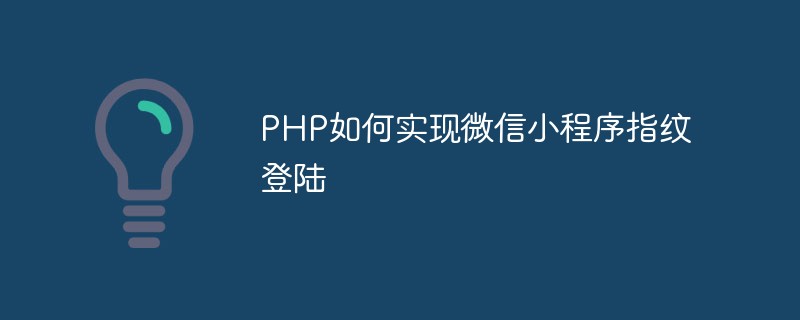
随着微信小程序的不断发展,越来越多的用户开始选择微信小程序进行登陆。为了提高用户的登录体验,微信小程序开始支持指纹登陆。在本文中,我们将会介绍如何使用PHP来实现微信小程序的指纹登陆。一、了解微信小程序的指纹登陆在微信小程序的基础上,开发者可以使用微信的指纹识别功能,让用户通过指纹登陆微信小程序,从而提高登录体验的安全性和便捷性。二、准备工作在使用PHP实现
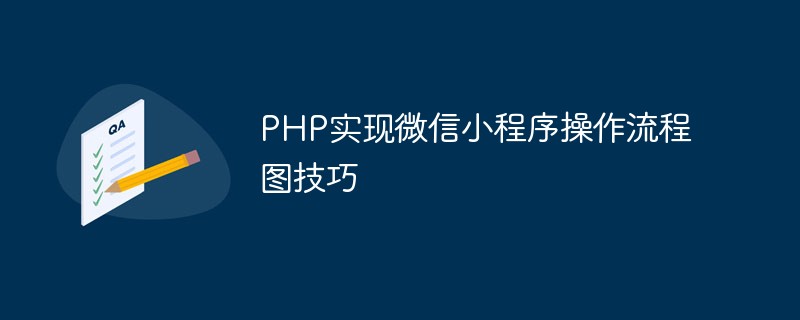
随着移动互联网的快速发展,微信小程序越来越受到广大用户的青睐,而PHP作为一种强大的编程语言,在小程序开发过程中也发挥着重要的作用。本文将介绍PHP实现微信小程序操作流程图的技巧。获取access_token在使用微信小程序开发过程中,首先需要获取access_token,它是实现微信小程序操作的重要凭证。在PHP中获取access_token的代码如下:f
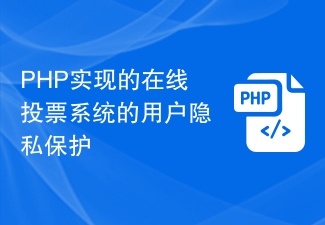
PHP实现的在线投票系统的用户隐私保护随着互联网的发展和普及,越来越多的投票活动开始转移到在线平台上进行。在线投票系统的便利性给用户带来了很多好处,但同时也引发了用户隐私泄露的担忧。隐私保护已经成为在线投票系统设计中的一个重要方面。本文将介绍如何使用PHP编写一个在线投票系统,并重点讨论用户隐私保护的问题。在设计和开发在线投票系统时,需要遵循以下几个原则来保
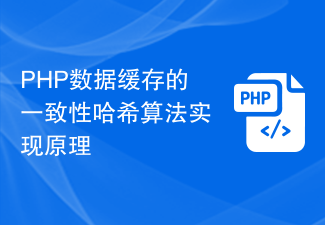
PHP数据缓存的一致性哈希算法实现原理一致性哈希算法(ConsistentHashing)是一种常用于分布式系统中数据缓存的算法,可以在系统扩展和缩减时,最小化数据迁移的数量。在PHP中,实现一致性哈希算法可以提高数据缓存的效率和可靠性,本文将介绍一致性哈希算法的原理,并提供代码示例。一致性哈希算法的基本原理传统的哈希算法将数据分散到不同的节点上,但当节点
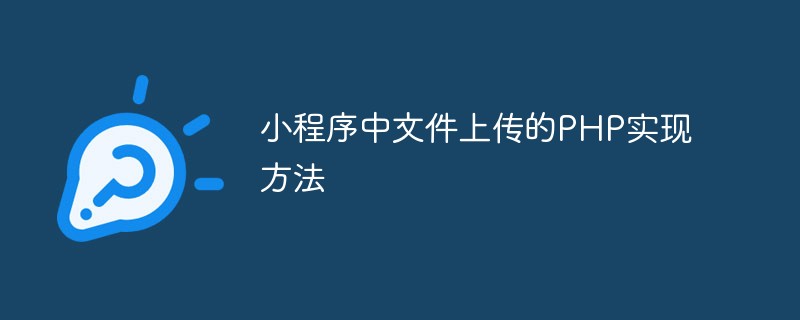
随着小程序的广泛应用,越来越多的开发者需要将其与后台服务器进行数据交互,其中最常见的业务场景之一就是上传文件。本文将介绍在小程序中实现文件上传的PHP后台实现方法。一、小程序中的文件上传在小程序中实现文件上传,主要依赖于小程序APIwx.uploadFile()。该API接受一个options对象作为参数,其中包含了要上传的文件路径、需要传递的其他数据以及
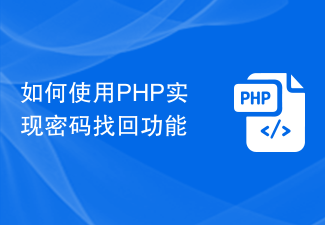
如何使用PHP实现密码找回功能密码是我们在线生活中的重要保护手段,但有时我们可能会忘记密码,特别是在拥有多个在线账号的情况下。为了帮助用户找回密码,许多网站都提供了密码找回功能。本文将介绍如何使用PHP来实现密码找回功能,并提供相关的代码示例。创建数据库表首先,我们需要创建一个数据库表来存储用户的相关信息,包括用户名、邮箱和密码找回的临时令牌等等。下面是一个


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
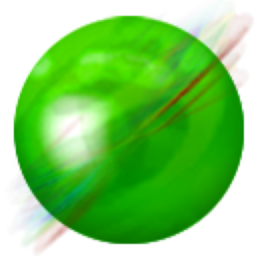
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment
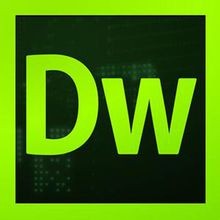
Dreamweaver CS6
Visual web development tools
