What are type assertions in Go language?
Go language is a statically typed programming language. Type assertion (Type Assertion) is one of the ways to determine the specific value type stored in the interface variable in the program. In the Go language, an interface variable can store any type of value, but the type information stored in the interface variable is limited, and all types of operations cannot be performed on the interface variable. Therefore, in actual applications, we need to judge and convert the specific value types stored in interface variables. This is what type assertions do.
Type assertions in the Go language come in two forms: value type assertions and pointer type assertions. Value type assertions and pointer type assertions have slightly different ways of judging and converting the value types of interface variables.
Value type assertion:
The syntax format of value type assertion is as follows:
x.(T)
Among them, x is a variable of interface type, and T represents a specific type. This type assertion is true if x stores a value of type T, and false otherwise.
The result of the type assertion has two values. The first value is the value after x is converted to type T. The second value is a Boolean value indicating whether the result of this type assertion is true. The specific code implementation is as follows:
var x interface{} x = "hello" s, ok := x.(string) if ok { fmt.Printf("x 类型为 string,值为 %s。 ", s) } else { fmt.Printf("x 不是 string 类型。 ") }
In the above code, an empty interface type variable x is first defined, and a string "hello" is assigned to the variable x. The value type assertion statement x.(string) attempts to convert the variable x to a string type, s represents the converted string, and ok represents whether the type assertion is successful. If ok is true, it means that the value type stored in x is a string type, and we can output the converted string s. If ok is false, it means that x is not a string type and the corresponding prompt information can be output.
Pointer type assertion:
The syntax format of pointer type assertion is similar to that of value type assertion, except that the pointer needs to be operated when asserting.
x.(*T)
Among them, *T represents the pointer type of type T. This type assertion is true if the value stored in x is of pointer type T, and false otherwise.
Like value type assertions, pointer type assertions also have two values. The first value is the value after x is converted into a T type pointer. The second value is a Boolean value, indicating the type assertion. Whether the result is true. The specific code implementation is as follows:
type Foo struct { bar string } func main() { var i interface{} = &Foo{"hello"} f, ok := i.(*Foo) if ok { fmt.Printf("i 是指针类型,指向 Foo 类型的变量,f.bar 的值为 %s。 ", f.bar) } else { fmt.Printf("类型断言失败。 ") } }
In the above code, a structure of type Foo is defined, an empty interface variable i is defined in the main function, and a structure pointing to type Foo is defined The pointer is assigned to variable i. Pointer type assertion x.(*Foo) attempts to convert variable x into a pointer type pointing to a structure of type Foo, f represents the converted pointer, and ok represents whether the type assertion is successful. If ok is true, it means that the value type stored in x is a pointer type pointing to a Foo type structure, and we can output the field value in the structure pointed to by the pointer. If ok is false, it means that x is not a pointer type pointing to a Foo type structure, and the corresponding prompt information can be output.
Summary:
Type assertion is a commonly used way to operate interface variables in the Go language. Type assertions can determine the type stored in the interface variable, and then make corresponding adjustments to it. operate. There are two forms of type assertions in Go language, value type assertions and pointer type assertions. When using type assertions, you need to pay attention to error handling to avoid runtime errors.
The above is the detailed content of What are type assertions in Go language?. For more information, please follow other related articles on the PHP Chinese website!
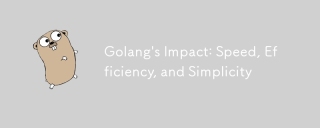
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
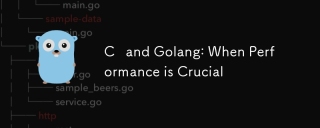
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
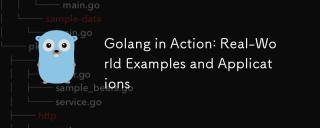
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
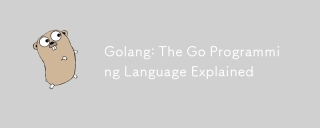
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
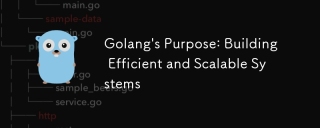
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
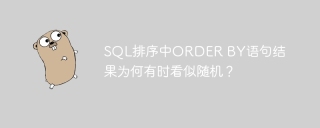
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
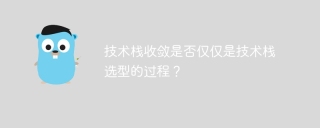
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
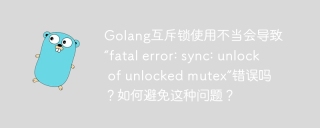
Golang ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
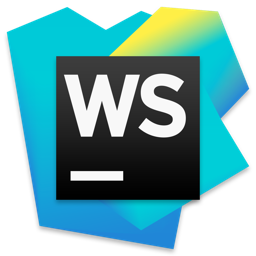
WebStorm Mac version
Useful JavaScript development tools
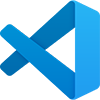
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool