How to use Go language for chatbot development?
With the rapid development of artificial intelligence technology, chatbots are playing an increasingly important role in business applications. Many companies have regarded chatbot development as an important development direction. The Go language has become the preferred language for more and more developers to develop chat robots due to its fast running speed, strong concurrency capabilities, and concise code. This article will introduce how to use Go language for chatbot development.
1. Introduction to chatbots
Chatbots, also called conversational robots, are programs that simulate human behavior for dialogue. It recognizes user input through natural language processing technology, and autonomously generates answers based on internal intent recognition and knowledge reserves. Chatbots can not only be used in intelligent customer service, marketing promotion, content recommendation, etc., but can also help users solve problems that are complex or cannot be accurately understood.
2. Characteristics of Go language
Go language is an open source, concurrent, compiled programming language developed by Google. It has the following characteristics:
- High concurrency: The concurrent processing mechanism of Goroutine (coroutine) can perform multiple tasks without thread overhead, greatly improving the concurrent processing capabilities of the program.
- GC (garbage collection) mechanism: Go language provides an automatic garbage collection mechanism to ensure the stability and reliability of the program when it is running.
- Easy to learn: The number and ambiguity of grammatical regulations are reduced in the Go language, and the code structure is clear and easy to read and maintain.
3. Go language chatbot development practice
Next, we will introduce the process of chatbot development using Go language through a simple example.
- Build a project
Before developing a chatbot, you first need to create a Go language project, which can be created through the following command:
$ mkdir chatbot $ cd chatbot $ go mod init chatbot
Use the above command to create After you initialize the project directory and initialize the go.mod file, you can write code in the project.
- Chatbot core code implementation
In the core code of Go language chatbot, there are two main functions: natural language processing and information reply. In this example, we will use Tencent Cloud API for natural language processing and achieve instant communication through sockets.
(1) Natural Language Processing
Tencent Cloud provides an API interface for natural language processing, which can help us implement text analysis, text error correction, sentiment analysis and other functions. In the Go language, we can realize the text analysis function by calling the API interface provided by Tencent Cloud. The sample code is as follows:
func nlp(text string) string { appID := "your app id" appKey := "your app key" nonceStr := uuid.NewV4().String() timeStamp := strconv.Itoa(int(time.Now().Unix())) sigStr := "app_id=" + appID + "&nonce_str=" + nonceStr + "&text=" + text + "&time_stamp=" + timeStamp + "&app_key=" + appKey sign := strings.ToUpper(md5.Sum([]byte(sigStr))) reqUrl := fmt.Sprintf("http://api.ai.qq.com/fcgi-bin/nlp/nlp_textchat?app_id=%s&nonce_str=%s&time_stamp=%s&question=%s&sign=%s", appID, nonceStr, timeStamp, url.QueryEscape(text), sign) resp, err := http.Get(reqUrl) if err != nil { log.Println(err) } defer resp.Body.Close() body, err := ioutil.ReadAll(resp.Body) if err != nil { log.Println(err) } type Result struct { Response string `json:"response"` Intent string `json:"intent"` Msg string `json:"msg"` Ret int `json:"ret"` } var result Result err = json.Unmarshal(body, &result) if err != nil { log.Println(err) } respText := "" if result.Ret == 0 { respText = result.Response } else { log.Println(result.Msg) } return respText }
By calling the above code, we can get the chatbot reply corresponding to the entered text.
(2) Message reply
In message reply, we realize instant communication with the user through socket connection. The following is an implementation of a simple socket connection service.
package main import ( "fmt" "log" "net" ) func handleConn(conn net.Conn) { defer conn.Close() buf := make([]byte, 1024) for { n, err := conn.Read(buf) if err != nil { log.Println(err) return } text := string(buf[:n]) log.Println(text) respText := nlp(text) conn.Write([]byte(respText)) } } func main() { listen, err := net.Listen("tcp", ":8080") if err != nil { log.Fatal(err) } for { conn, err := listen.Accept() if err != nil { log.Println(err) continue } go handleConn(conn) } }
With the above code, we can establish a server-side socket connection, and by calling the nlp function, we can get a reply from the robot every time we receive a message.
- Run the chatbot project
After the above code is written, we can start the chatbot server through the following command:
$ go run main.go
After the chatbot server is started , we can connect to the server through the local client and obtain instant communication with the chatbot
$ telnet localhost 8080 Trying ::1... Connected to localhost. Escape character is '^]'. hello 早上好 world 你好啊
Enter hello, the robot will reply good morning, enter world, the robot will reply hello.
At this point, the development of a simple chatbot using Go language is completed.
4. Summary
This article introduces how to use Go language to develop chatbots. Chatbots are currently a very popular application field and have a wide range of application scenarios in commercial applications. Through the chatbot development practices provided in this article, readers can initially master the skills of chatbot development using the Go language, and further explore and develop more complex commercial chatbot applications.
The above is the detailed content of How to use Go language for chatbot development?. For more information, please follow other related articles on the PHP Chinese website!
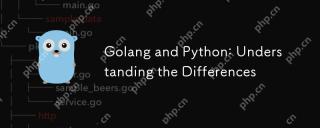
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
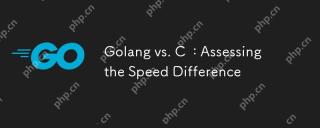
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
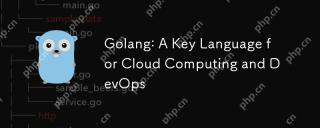
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.
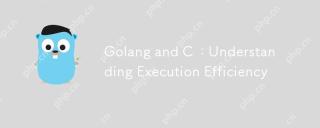
Golang and C each have their own advantages in performance efficiency. 1) Golang improves efficiency through goroutine and garbage collection, but may introduce pause time. 2) C realizes high performance through manual memory management and optimization, but developers need to deal with memory leaks and other issues. When choosing, you need to consider project requirements and team technology stack.
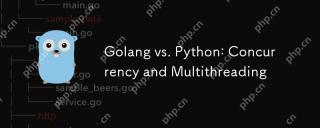
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
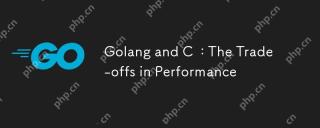
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
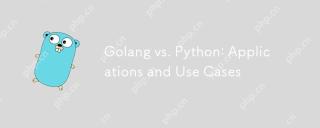
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
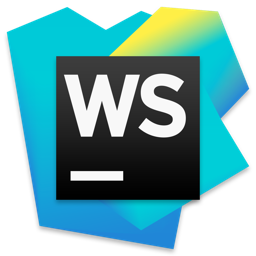
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor