Python is a powerful and flexible programming language that is widely used in various fields. Among them, the generator is a key element in Python. It has many advantages and can effectively improve the running efficiency of the code. This article will give you a detailed introduction to generators in Python.
1. What is a generator?
A generator is a special type of function that allows you to iterate over a sequence without building a complete sequence beforehand. This means that the generator will only generate each element when it is requested. This process is also known as the "iterator protocol".
Using generators can greatly save memory usage and computing resources, which is very useful for processing large-scale data and complex computing tasks.
You can create a generator in two ways:
1. Change the function definition to a generator function and use the yield keyword instead of the return keyword.
2. Use generator expressions, similar to list comprehensions.
The following is an example of a generator function:
def fibonacci(limit): # 初始值 a, b = 0, 1 while a < limit: yield a a, b = b, a + b
In this example, we define a generator function named fibonacci. It uses a while loop to generate the Fibonacci sequence and uses the yield keyword to return the generator in each loop.
When this function is called, it will not be executed immediately, but will return a generator object. As you iterate over this generator object, it generates the next Fibonacci number on each iteration.
For example:
fib = fibonacci(10) for i in fib: print(i)
The output result of this code will be 0, 1, 1, 2, 3, 5, 8.
2. Generator expression
Generator expression is a simpler way to create a generator. It is similar to a list comprehension, but returns a generator object. The syntax of a generator expression is:
(表达式 for 变量 in 序列 if 条件)
where the expression is the value to be returned by the generator, the variable is a loop variable, the sequence is the sequence to be traversed by the variable, and the condition is optional.
For example:
gen = (i ** 2 for i in range(10) if i % 2 != 0)
This generator expression returns the square of all odd numbers from 0 to 9. By calling the next() method, we can obtain these values one by one:
print(next(gen)) # 输出1 print(next(gen)) # 输出9 print(next(gen)) # 输出25
3. Advantages of the generator
1. It takes up small memory
The generator is lazy A calculation mechanism that generates calculation results only when needed. Therefore, a lot of memory space is saved.
2. High operating efficiency
The generator avoids the large computational and storage overhead required to calculate all results. This makes the generator very useful for processing large data sets and periodic tasks.
3. Easy to implement
Python provides a simple and flexible generator mechanism, making it very easy to write generators.
4. Mutable
A generator can be modified, such as appending elements to it or removing elements from it.
5. Summary
Generator is a very useful data type in Python, which can greatly improve the running efficiency of the code. It generates calculation results only when needed and can be modified. This article introduces you to generators in Python, including generator functions and generator expressions. By understanding the benefits and workings of generators, you can better utilize this efficient programming technique.
The above is the detailed content of Detailed explanation of generators in Python. For more information, please follow other related articles on the PHP Chinese website!
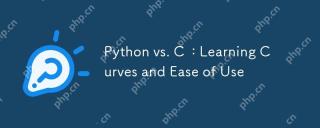
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.

Python and C have significant differences in memory management and control. 1. Python uses automatic memory management, based on reference counting and garbage collection, simplifying the work of programmers. 2.C requires manual management of memory, providing more control but increasing complexity and error risk. Which language to choose should be based on project requirements and team technology stack.
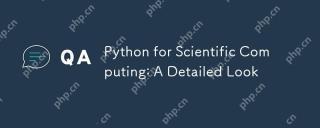
Python's applications in scientific computing include data analysis, machine learning, numerical simulation and visualization. 1.Numpy provides efficient multi-dimensional arrays and mathematical functions. 2. SciPy extends Numpy functionality and provides optimization and linear algebra tools. 3. Pandas is used for data processing and analysis. 4.Matplotlib is used to generate various graphs and visual results.
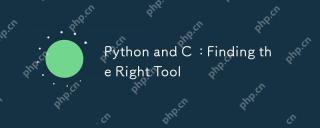
Whether to choose Python or C depends on project requirements: 1) Python is suitable for rapid development, data science, and scripting because of its concise syntax and rich libraries; 2) C is suitable for scenarios that require high performance and underlying control, such as system programming and game development, because of its compilation and manual memory management.
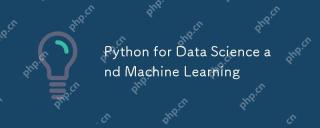
Python is widely used in data science and machine learning, mainly relying on its simplicity and a powerful library ecosystem. 1) Pandas is used for data processing and analysis, 2) Numpy provides efficient numerical calculations, and 3) Scikit-learn is used for machine learning model construction and optimization, these libraries make Python an ideal tool for data science and machine learning.
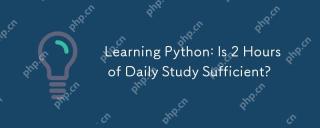
Is it enough to learn Python for two hours a day? It depends on your goals and learning methods. 1) Develop a clear learning plan, 2) Select appropriate learning resources and methods, 3) Practice and review and consolidate hands-on practice and review and consolidate, and you can gradually master the basic knowledge and advanced functions of Python during this period.
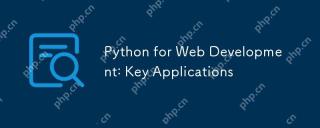
Key applications of Python in web development include the use of Django and Flask frameworks, API development, data analysis and visualization, machine learning and AI, and performance optimization. 1. Django and Flask framework: Django is suitable for rapid development of complex applications, and Flask is suitable for small or highly customized projects. 2. API development: Use Flask or DjangoRESTFramework to build RESTfulAPI. 3. Data analysis and visualization: Use Python to process data and display it through the web interface. 4. Machine Learning and AI: Python is used to build intelligent web applications. 5. Performance optimization: optimized through asynchronous programming, caching and code
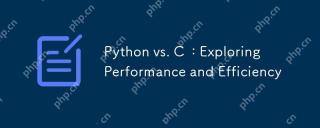
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
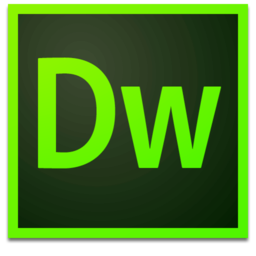
Dreamweaver Mac version
Visual web development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use
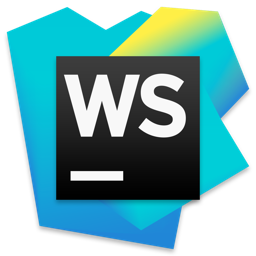
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.