Java is a widely used programming language with a wealth of built-in libraries and tool classes. These tool classes provide Java developers with many convenient functions and accelerate the software development process. In this article, we will introduce several common tool classes in the Java language, as well as their uses and sample code.
- ArrayList class in java.util package
ArrayList is one of the very common tool classes in Java, used to create dynamic arrays. It can adjust the size of the array at runtime and is an array with variable capacity. ArrayList provides many methods for operating lists, such as adding, deleting, getting elements, etc. The following is a sample code:
import java.util.ArrayList; public class ArrayListExample { public static void main(String[] args) { ArrayList<String> list = new ArrayList<String>(); // 添加元素 list.add("Hello"); list.add("World"); list.add("Java"); // 删除元素 list.remove("Java"); // 获取元素 String element = list.get(1); System.out.println(element); } }
The output result is:
World
- HashMap class in the java.util package
HashMap is used in Java Utility class for storing key-value pairs. It is implemented based on a hash table and provides O(1) constant time complexity to perform put() and get() operations. HashMap also provides many other useful methods, such as checking for contained keys, getting the number of keys, etc. The following is a sample code:
import java.util.HashMap; public class HashMapExample { public static void main(String[] args) { HashMap<String, Integer> map = new HashMap<String, Integer>(); // 添加键值对 map.put("John", 30); map.put("Mary", 25); map.put("Bob", 40); // 获取值 int age = map.get("John"); System.out.println(age); // 检查是否有键 boolean exist = map.containsKey("Mary"); System.out.println(exist); } }
The output result is:
30 true
- File class in the java.io package
File is used in Java Utility classes for operating files and directories. It provides many methods for operating files and directories, such as creating files, renaming files, obtaining file information, etc. The following is a sample code:
import java.io.File; import java.io.IOException; public class FileExample { public static void main(String[] args) { File file = new File("C:/example.txt"); try { // 创建文件 boolean isCreated = file.createNewFile(); System.out.println(isCreated); // 重命名文件 File newFile = new File("C:/newexample.txt"); boolean isRenamed = file.renameTo(newFile); System.out.println(isRenamed); // 获取文件信息 boolean isFile = file.isFile(); long size = file.length(); System.out.println(isFile); System.out.println(size); } catch (IOException e) { e.printStackTrace(); } } }
The output result is:
true true true 0
- SimpleDateFormat class in the java.text package
SimpleDateFormat is used in Java Utility class for formatting dates and times. It can parse a date string into a date object, or format a date object into a specified string format. The following is a sample code:
import java.text.SimpleDateFormat; import java.util.Date; public class SimpleDateFormatExample { public static void main(String[] args) { SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); Date date = new Date(); // 格式化日期对象 String dateString = format.format(date); System.out.println(dateString); // 解析日期字符串 String dateString2 = "2021-08-01 15:30:45"; try { Date date2 = format.parse(dateString2); System.out.println(date2); } catch (ParseException e) { e.printStackTrace(); } } }
The output is:
2021-08-01 15:59:40 Sun Aug 01 15:30:45 CST 2021
These tool classes are only part of the Java language, and there are many other useful tool classes that can speed up the development process. If you want to know more about Java tool classes, you can refer to Oracle official documentation or other related books.
The above is the detailed content of Introduction to tool classes in Java language. For more information, please follow other related articles on the PHP Chinese website!
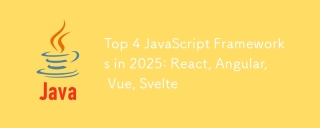
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
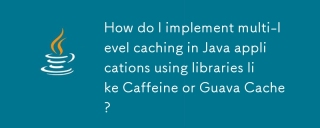
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
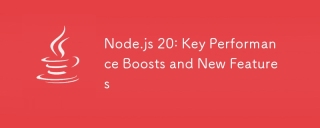
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
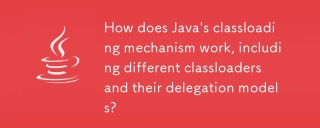
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
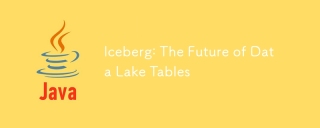
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
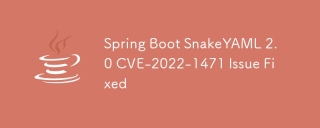
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
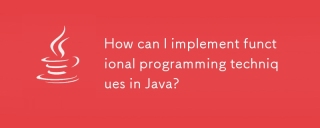
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor
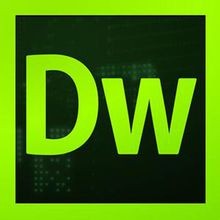
Dreamweaver CS6
Visual web development tools
