With the rapid development of the Internet, people can obtain the information they need through various channels. In this information age, web crawlers have become an indispensable tool. In this article, we will introduce the actual crawler in Python-58 city crawler.
1. Introduction to crawlers
A web crawler is an automated program that accesses web pages through the HTTP protocol and extracts the required data. On the Internet, there is a lot of data, but not all of it is available through APIs. Therefore, crawlers have become an important means of obtaining data.
The workflow of a crawler is generally divided into three steps:
- Download web pages: download web pages through HTTP protocol, usually implemented using the requests library;
- Parse web pages: The downloaded web page parses and extracts the required data, which is generally implemented using the BeautifulSoup4 library;
- Storage data: Save the required data locally or in a database.
2. Practical crawler combat: 58 city crawler
58 city is a national classified information website, where users can publish product information, rental information, recruitment information, etc. This article will introduce how to implement the 58 city crawler through Python to obtain rental information.
- Analyze the website
Before crawling, you need to analyze the 58 city website. By entering the rental page and selecting the desired city, you can find that the URL contains city information. For example, the URL of the rental page is: "https://[city pinyin].58.com/zufang/". By modifying the city pinyin in the URL, you can crawl rental information in other cities.
After opening the rental page, you can find that the page structure is divided into two parts: the search bar and the rental information list. The rental information list includes the title, rent, area, geographical location, housing type and other information of each rental information.
- Writing a crawler
After analyzing the 58.com website, just write a crawler. First, you need to import the requests and BeautifulSoup4 libraries. The code is as follows:
import requests from bs4 import BeautifulSoup
Next, obtaining the rental information in each city requires constructing the correct URL. The code is as follows:
city_pinyin = "bj" url = "https://{}.58.com/zufang/".format(city_pinyin)
After obtaining the correct URL, you can use the requests library to obtain the HTML source code of the page. The code is as follows:
response = requests.get(url) html = response.text
Now that you have obtained the HTML source code of the rental page, you need to use the BeautifulSoup4 library to parse the HTML source code and extract the required data. According to the page structure, the rental information list is contained in a div tag with a class of "list-wrap". We can obtain all div tags with class "list-wrap" through the find_all() function in the BeautifulSoup4 library. The code is as follows:
soup = BeautifulSoup(html, "lxml") div_list = soup.find_all("div", class_="list-wrap")
After obtaining the div tag, you can traverse the tag list and extract the data of each rental information. According to the page structure, each piece of rental information is contained in a div tag with class "des", including title, rent, area, geographical location, housing type and other information. The code is as follows:
for div in div_list: info_list = div.find_all("div", class_="des") for info in info_list: # 提取需要的租房数据
In the for loop, we used the find_all() function to obtain all div tags with class "des". Next, we need to traverse these div tags and extract the required rental data. For example, the code to extract the title and other information of the rental information is as follows:
title = info.find("a", class_="t").text rent = info.find("b").text size = info.find_all("p")[0].text.split("/")[1] address = info.find_all("p")[0].text.split("/")[0] house_type = info.find_all("p")[1].text
Through the above code, we have successfully obtained each piece of rental information on the 58 city rental page and encapsulated it into variables. Next, by printing the variables of each rental information, you can see the data output on the console. For example:
print("标题:{}".format(title)) print("租金:{}".format(rent)) print("面积:{}".format(size)) print("地理位置:{}".format(address)) print("房屋类型:{}".format(house_type))
3. Summary
This article introduces the actual crawler in Python-58 city crawler. Before the crawler was implemented, we first analyzed the 58 city rental page and determined the URL to obtain rental information and the data that needed to be extracted. Then, the crawler was implemented using requests and BeautifulSoup4 library. Through the crawler, we successfully obtained the rental information of the 58 city rental page and encapsulated it into variables to facilitate subsequent data processing.
The above is the detailed content of Practical crawler combat in Python: 58 city crawler. For more information, please follow other related articles on the PHP Chinese website!
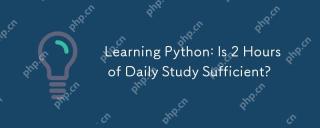
Is it enough to learn Python for two hours a day? It depends on your goals and learning methods. 1) Develop a clear learning plan, 2) Select appropriate learning resources and methods, 3) Practice and review and consolidate hands-on practice and review and consolidate, and you can gradually master the basic knowledge and advanced functions of Python during this period.
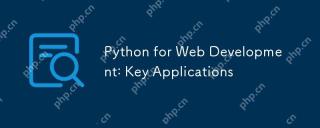
Key applications of Python in web development include the use of Django and Flask frameworks, API development, data analysis and visualization, machine learning and AI, and performance optimization. 1. Django and Flask framework: Django is suitable for rapid development of complex applications, and Flask is suitable for small or highly customized projects. 2. API development: Use Flask or DjangoRESTFramework to build RESTfulAPI. 3. Data analysis and visualization: Use Python to process data and display it through the web interface. 4. Machine Learning and AI: Python is used to build intelligent web applications. 5. Performance optimization: optimized through asynchronous programming, caching and code
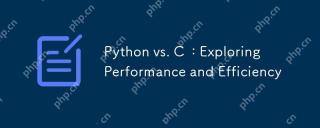
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
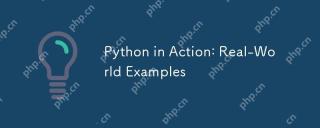
Python's real-world applications include data analytics, web development, artificial intelligence and automation. 1) In data analysis, Python uses Pandas and Matplotlib to process and visualize data. 2) In web development, Django and Flask frameworks simplify the creation of web applications. 3) In the field of artificial intelligence, TensorFlow and PyTorch are used to build and train models. 4) In terms of automation, Python scripts can be used for tasks such as copying files.
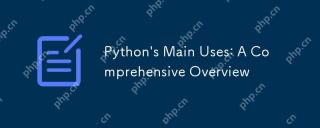
Python is widely used in data science, web development and automation scripting fields. 1) In data science, Python simplifies data processing and analysis through libraries such as NumPy and Pandas. 2) In web development, the Django and Flask frameworks enable developers to quickly build applications. 3) In automated scripts, Python's simplicity and standard library make it ideal.
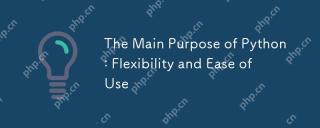
Python's flexibility is reflected in multi-paradigm support and dynamic type systems, while ease of use comes from a simple syntax and rich standard library. 1. Flexibility: Supports object-oriented, functional and procedural programming, and dynamic type systems improve development efficiency. 2. Ease of use: The grammar is close to natural language, the standard library covers a wide range of functions, and simplifies the development process.
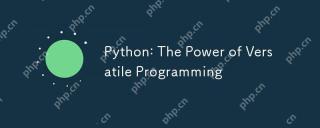
Python is highly favored for its simplicity and power, suitable for all needs from beginners to advanced developers. Its versatility is reflected in: 1) Easy to learn and use, simple syntax; 2) Rich libraries and frameworks, such as NumPy, Pandas, etc.; 3) Cross-platform support, which can be run on a variety of operating systems; 4) Suitable for scripting and automation tasks to improve work efficiency.
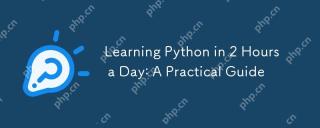
Yes, learn Python in two hours a day. 1. Develop a reasonable study plan, 2. Select the right learning resources, 3. Consolidate the knowledge learned through practice. These steps can help you master Python in a short time.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
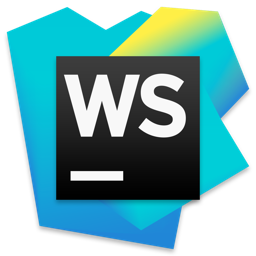
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor