Go language is a powerful programming language that has been widely used in many fields. Error handling is an integral part of Go. Because various errors and exceptions will inevitably occur in programs, how to effectively capture and handle these errors greatly affects the reliability, stability, and maintainability of the program. This article will introduce error handling methods in the Go language, as well as their application scenarios, advantages and disadvantages, etc.
- Error handling overview
In Go, an error is treated as a value, which is typically used to represent the reason why a function or method call failed. The error type is one of the built-in predeclared types. Its definition is as follows:
type error interface {
Error() string
}
A value that implements the error interface can be viewed An error indicating the reason why a function or method call failed. Error information can be passed by returning the error value. The sample code is as follows:
func doSomething() error {
if someErrorOccurs { return errors.New("some error occurred") } return nil
}
In the Go language, error handling is generally used way to return. The advantage of this approach is that it can avoid the overhead of exception throwing/catching and improve program performance. In addition, Go also provides a variety of ways to handle errors, including panic/recover, defer, and custom error types.
- Error handling method
(1) Error return
In Go, the most commonly used error handling method is to use the error return method. Typically, a function or method returns two values: one is the actual return value of the function or method, and the other is an error value of type error. The caller can determine whether the function or method call is successful based on the returned error value. The sample code is as follows:
func doSomething() (string, error) {
if someErrorOccurs { return "", errors.New("some error occurred") } return "ok", nil
}
After calling the doSomething() function, you can handle possible errors in the following way:
result, err := doSomething()
if err != nil {
// 处理错误 fmt.Println("error:", err) return
}
// Process the correct result
fmt.Println("result:", result)
(2) panic/recover
The panic/recover mechanism in Go language Can also be used for error handling. When an error or exception is encountered during the execution of a function or method, an error/exception can be thrown by calling the panic() function; the error/exception can be captured and processed through the defer keyword and recover() function. .
It should be noted that when using the panic/recover mechanism, it should only be used when it is very necessary, because it will have a greater impact on program performance, and improper use may cause program instability.
The sample code is as follows:
func doSomething() {
defer func() { if err := recover(); err != nil { // 处理错误 fmt.Println("error:", err) } }() if someErrorOccurs { panic("some error occurred") }
}
When calling the doSomething() function, you can use the recover() function to To capture possible errors, the sample code is as follows:
doSomething()
(3) defer
The defer mechanism is one of the very practical mechanisms in the Go language. It is usually Used for resource release, error handling, etc. The defer statement will postpone the execution of subsequent function calls until the function returns, and can handle resource release and error handling at the end of function execution.
The sample code is as follows:
func doSomething() (string, error) {
// 打开文件 f, err := os.Open("filename.txt") if err != nil { // 处理错误 return "", err } // 在函数返回之前关闭文件 defer f.Close() // 处理文件内容 // ...
}
In the above code, the defer statement is used to delay Close open files to ensure resources are released before the function returns to avoid problems such as memory leaks.
(4) Custom error types
Sometimes, you may need to customize some error types in the program to better distinguish different error types and provide more precise error prompt information .
In Go, we can implement a custom error type by defining a custom type that implements the error interface. The sample code is as follows:
type MyError struct {
Msg string
}
func (e MyError) Error() string {
return fmt.Sprintf("my error: %v", e.Msg)
}
func doSomething() error {
if someErrorOccurs { return MyError{"some error occurred"} } return nil
}
In the above code, we define a MyError type and implement the Error() method of the error interface to return customized error message. If an error occurs when calling the doSomething() function, an error value of type MyError will be returned.
- Summary
In the Go language, error handling is an important topic. Correctly handling various errors and exceptions that may occur in the program can greatly improve the program. reliability and stability. This article introduces several commonly used error handling methods in the Go language, including error return, panic/recover, defer, and custom error types, and analyzes their advantages, disadvantages, and applicable scenarios. In the application, it is necessary to choose the most appropriate error handling method according to the specific situation, and ensure the correctness, clarity and readability of the error handling code to improve the maintainability and scalability of the program.
The above is the detailed content of What are the error handling methods in Go language?. For more information, please follow other related articles on the PHP Chinese website!
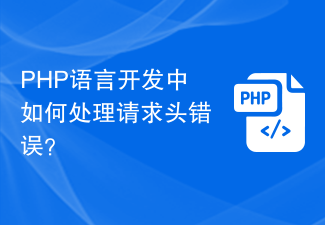
在PHP语言开发中,请求头错误通常是由于HTTP请求中的一些问题导致的。这些问题可能包括无效的请求头、缺失的请求体以及无法识别的编码格式等。而正确处理这些请求头错误是保证应用程序稳定性和安全性的关键。在本文中,我们将讨论一些处理PHP请求头错误的最佳实践,帮助您构建更加可靠和安全的应用程序。检查请求方法HTTP协议规定了一组可用的请求方法(例如GET、POS
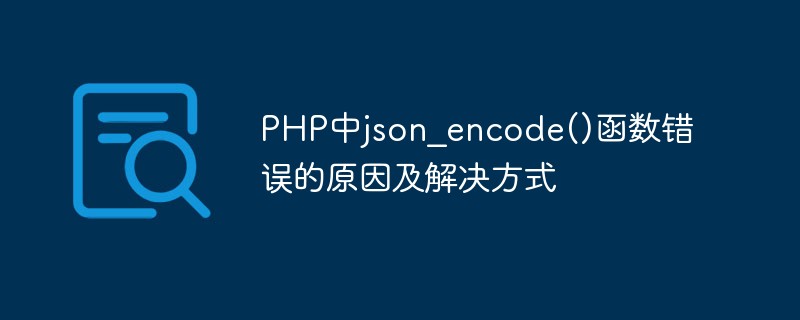
随着Web应用程序的不断发展,数据交互成为了一个非常重要的环节。其中,JSON(JavaScriptObjectNotation)是一种轻量级的数据交换格式,广泛用于前后端数据交互。在PHP中,json_encode()函数可以将PHP数组或对象转换为JSON格式字符串,json_decode()函数可以将JSON格式字符串转换为PHP数组或对象。然而,
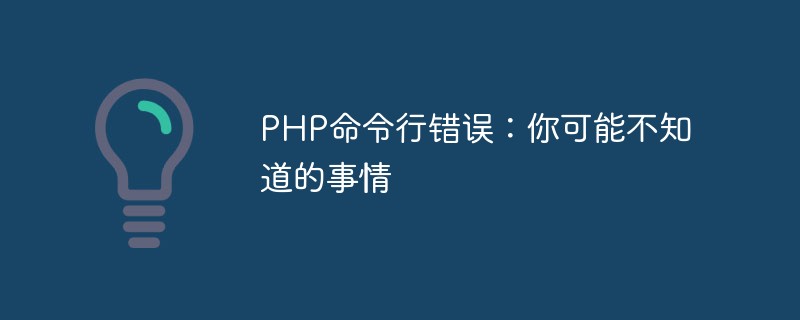
本文将介绍关于PHP命令行错误的一些你可能不知道的事情。PHP作为一门流行的服务器端语言,一般运行在Web服务器上,但它也可以在命令行上直接运行,比如在Linux或者MacOS系统下,我们可以在终端中输入“php”命令来直接运行PHP脚本。不过,就像在Web服务器中一样,当我们在命令行中运行PHP脚本时,也会遇到一些错误。以下是一些你可能不知道的有关PHP命
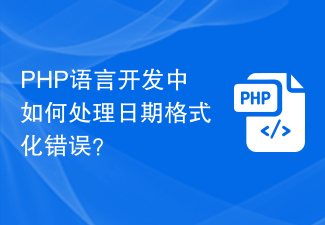
在PHP语言开发中,日期格式化错误是一个常见的问题。正确的日期格式对于程序员来说十分重要,因为它决定着代码的可读性、可维护性和正确性。本文将分享一些处理日期格式化错误的技巧。了解日期格式在处理日期格式化错误之前,我们必须先了解日期格式。日期格式是由各种字母和符号组成的字符串,用于表示特定的日期和时间格式。在PHP中,常见的日期格式包括:Y:四位数年份(如20
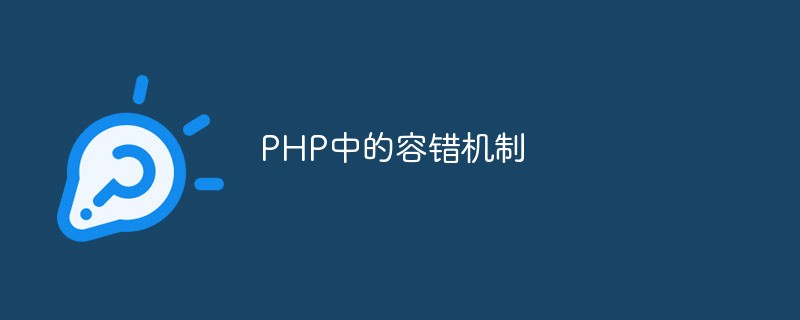
在编写程序时总会存在各种各样的错误和异常。任何编程语言都需要有良好的容错机制,PHP也不例外。PHP有许多内置的错误和异常处理机制,可以让开发者更好地管理其代码,并正确地处理各种问题。下面就让我们一起来了解一下PHP中的容错机制。错误级别PHP中有四个错误级别:致命错误、严重错误、警告和通知。每个错误级别都有一个不同的符号表示,以帮助识别和处理错误:E_ER
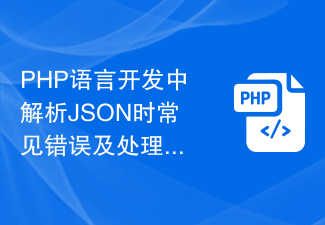
在PHP语言开发中,常常需要解析JSON数据,以便进行后续的数据处理和操作。然而,在解析JSON时,很容易遇到各种错误和问题。本文将介绍常见的错误和处理方法,帮助PHP开发者更好地处理JSON数据。一、JSON格式错误最常见的错误是JSON格式不正确。JSON数据必须符合JSON规范,即数据必须是键值对的集合,并使用大括号({})和中括号([])来包含数据。
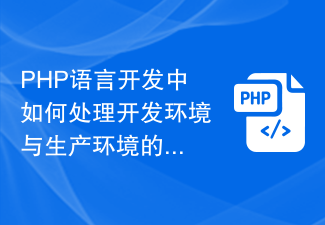
随着互联网的快速发展,开发人员的任务也随之多样化和复杂化。特别是对于PHP语言开发人员而言,在开发过程中面临的最常见问题之一就是在开发环境和生产环境中,数据不一致的错误问题。因此,在开发PHP应用程序时,如何处理这些错误是开发人员必须面对的一个重要问题。开发环境和生产环境的区别首先需要明确的是,开发环境和生产环境是不同的,它们有着不同的设置和配置。在开发环境
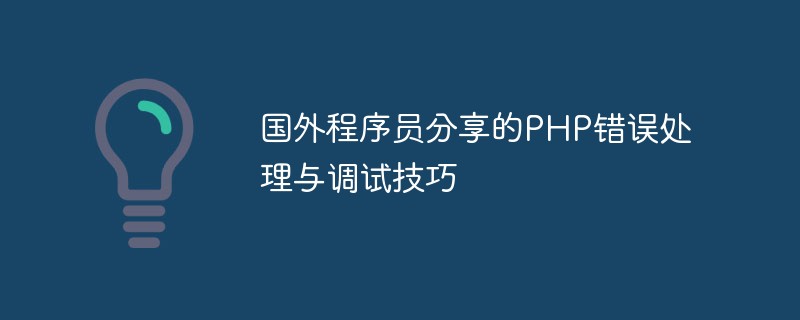
PHP(HypertextPreprocessor)是一种广泛用于Web开发的脚本语言。在开发PHP应用程序时,错误处理和调试被认为是非常重要的一块。国外程序员在经验中积累了许多PHP错误处理和调试技巧,下面介绍一些比较常见和实用的技巧。错误报告级别修改在PHP中,通过修改错误报告级别可以显示或禁止显示特定类型的PHP错误。通过设置错误报告级别为“E_AL


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
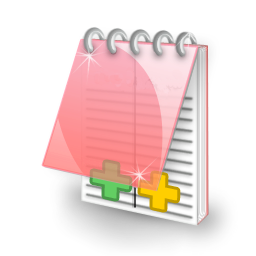
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version
