Practical sharing on using vuex to manage global status in Vue
Vue is a popular front-end framework. Its basic idea is to divide the page into different components, each component has its own state. However, there are situations where we need to share state between multiple components. To deal with this situation, Vue provides vuex, a third-party library specifically designed to manage the global state of the application. In this article, we will share some practical experiences using vuex in Vue projects.
What is Vuex?
Vuex is the official state management tool for Vue.js. It uses a centralized storage to manage the state of all components of the application, implemented through a single object.
The core concepts of Vuex include:
- State: Stores application-level state.
- Getter: Get the value stored in state and perform calculations.
- Mutation: Change the value in state.
- Action: Submit mutation and perform asynchronous operation.
- Module: Decompose the store into multiple sub-modules. Each module can have its own state, mutation, action
Use Vuex in the Vue project
For To demonstrate the process of using Vuex in a Vue project, we will create a simple sample application we call "ToDo List" that allows us to add and remove tasks and display them in a task list. Below we will introduce how to use Vuex to implement this ToDo List application.
First, in order to use Vuex, we need to import it in our Vue application. We can import and inject the Vuex object in main.js as follows:
import Vue from 'vue' import Vuex from 'vuex' import App from './App.vue' Vue.use(Vuex) const store = new Vuex.Store({ state: { tasks: [] }, mutations: { addTask(state, task) { state.tasks.push(task) }, removeTask(state, index) { state.tasks.splice(index, 1) } } }) new Vue({ store, render: h => h(App) }).$mount('#app')
Here, we first import Vuex and inject it into the Vue object. Next, we will create a constant called store that creates a new Vuex storage instance using the Vuex.Store constructor. Here we define the state and mutations in our store. Our status is the tasks array, which stores the tasks in our ToDo List. Our mutations include addTask and removeTask, which are used to add and remove tasks respectively.
We also inject the store into the Vue instance so we can use it in all components of our application. Now, we can access the state and mutations in the store in our component.
In our sample ToDo List application, we have a component called AddTask.vue that contains a form that allows the user to add tasks. In this component, we can use $store to access the state and mutations in the store, and trigger our addTask mutation to add the task to the store. The code is as follows:
<template> <form @submit.prevent="addTask"> <input type="text" v-model="task" /> <button type="submit">Add Task</button> </form> </template> <script> export default { data() { return { task: '' } }, methods: { addTask() { this.$store.commit('addTask', this.task) this.task = '' } } } </script>
Here, we use a form in the component, and when the form is submitted, we call the addTask method and pass the task name as a parameter to the $store.commit method. This triggers the addTask mutation we defined earlier to add the task to the store.
Next, we have a TasksList.vue component that displays a list of tasks and allows the user to delete tasks. In this component, we can use $store to access the state and mutations in the store, and trigger our removeTask mutation to remove the task from the store. The code is as follows:
<template> <ul> <li v-for="(task, index) in tasks" :key="index"> {{ task }} <button @click="removeTask(index)">Remove Task</button> </li> </ul> </template> <script> export default { computed: { tasks() { return this.$store.state.tasks } }, methods: { removeTask(index) { this.$store.commit('removeTask', index) } } } </script>
Here, we use a computed attribute to obtain the tasks array status in the store. We also use the v-for directive in the template to display each task and add a delete button after each task. When the user clicks a delete button, we call the removeTask method and pass the task's index as a parameter to the $store.commit method. This triggers the removeTask mutation we defined previously, which removes the task from the store.
Conclusion
In this article, we learned how to use Vuex to manage the global state of our application and demonstrated how to actually use it in a Vue project through a ToDo List application it. In a Vue project, using Vuex allows us to manage and share state more easily, and makes our code more modular and readable. If you are also developing a Vue project, you may wish to consider using Vuex to better manage the state of your application!
The above is the detailed content of Practical sharing on using vuex to manage global status in Vue. For more information, please follow other related articles on the PHP Chinese website!

Netflix's choice in front-end technology mainly focuses on three aspects: performance optimization, scalability and user experience. 1. Performance optimization: Netflix chose React as the main framework and developed tools such as SpeedCurve and Boomerang to monitor and optimize the user experience. 2. Scalability: They adopt a micro front-end architecture, splitting applications into independent modules, improving development efficiency and system scalability. 3. User experience: Netflix uses the Material-UI component library to continuously optimize the interface through A/B testing and user feedback to ensure consistency and aesthetics.

Netflixusesacustomframeworkcalled"Gibbon"builtonReact,notReactorVuedirectly.1)TeamExperience:Choosebasedonfamiliarity.2)ProjectComplexity:Vueforsimplerprojects,Reactforcomplexones.3)CustomizationNeeds:Reactoffersmoreflexibility.4)Ecosystema

Netflix mainly considers performance, scalability, development efficiency, ecosystem, technical debt and maintenance costs in framework selection. 1. Performance and scalability: Java and SpringBoot are selected to efficiently process massive data and high concurrent requests. 2. Development efficiency and ecosystem: Use React to improve front-end development efficiency and utilize its rich ecosystem. 3. Technical debt and maintenance costs: Choose Node.js to build microservices to reduce maintenance costs and technical debt.

Netflix mainly uses React as the front-end framework, supplemented by Vue for specific functions. 1) React's componentization and virtual DOM improve the performance and development efficiency of Netflix applications. 2) Vue is used in Netflix's internal tools and small projects, and its flexibility and ease of use are key.

Vue.js is a progressive JavaScript framework suitable for building complex user interfaces. 1) Its core concepts include responsive data, componentization and virtual DOM. 2) In practical applications, it can be demonstrated by building Todo applications and integrating VueRouter. 3) When debugging, it is recommended to use VueDevtools and console.log. 4) Performance optimization can be achieved through v-if/v-show, list rendering optimization, asynchronous loading of components, etc.

Vue.js is suitable for small to medium-sized projects, while React is more suitable for large and complex applications. 1. Vue.js' responsive system automatically updates the DOM through dependency tracking, making it easy to manage data changes. 2.React adopts a one-way data flow, and data flows from the parent component to the child component, providing a clear data flow and an easy-to-debug structure.

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.
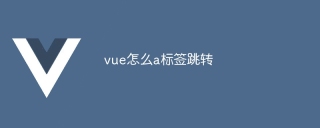
The methods to implement the jump of a tag in Vue include: using the a tag in the HTML template to specify the href attribute. Use the router-link component of Vue routing. Use this.$router.push() method in JavaScript. Parameters can be passed through the query parameter and routes are configured in the router options for dynamic jumps.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
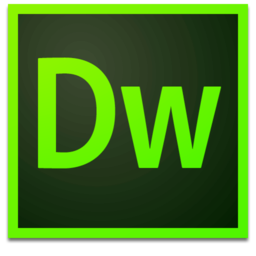
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.