How to do functional programming with PHP
PHP is a widely used server-side language. One of the reasons why many web developers like to use PHP is its rich function library and simple and easy-to-use function syntax. Functional programming is a programming paradigm that well encapsulates data and behavior, making the code more modular and easy to maintain and test. In this article, we will introduce how to use PHP for functional programming.
- Functional Programming Basics
The core idea of functional programming is to treat functions as first-class citizens, and functions themselves can be passed, returned, and composed like variables. In functional programming, we don't modify mutable state, but we transform and filter it through functions.
PHP itself supports functional programming, and there are many built-in functions that can be used for functional processing. For example array_map, array_filter, etc. Below we will demonstrate how to use these functions to implement common functional programming operations.
- Higher-order functions
Higher-order functions refer to functions that can accept functions as parameters or return functions. Such functions can be used to compose and reuse code. In PHP, commonly used higher-order functions include array_map, array_filter, array_reduce, etc.
The array_map function can accept a function and an array as parameters and return a new array. The elements of the new array are the values obtained after the elements in the original array are converted by the function.
For example, we have an array $x=[1,2,3,4]$ and want to square each element in the array. You can use the following code:
function square($x) { return $x * $x; } $array = [1, 2, 3, 4]; $new_array = array_map('square', $array); var_dump($new_array); // 输出 [1, 4, 9, 16]## The #array_filter function can accept a function and an array as parameters and return a new array. The elements in the new array are the elements in the original array that meet the conditions. For example, we have an array $x=[1,2,3,4]$ and want to get all the even numbers in the array. You can use the following code:
function is_even($x) { return $x % 2 == 0; } $array = [1, 2, 3, 4]; $new_array = array_filter($array, 'is_even'); var_dump($new_array); // 输出 [2, 4]array_reduce function can accept Takes a function, an array, and an initial value as arguments and returns an accumulated result. The reduce function passes each element in the array to a function for calculation, and then gets a cumulative result. For example, we have an array $x=[1,2,3,4]$ and want to accumulate all elements in the array. You can use the following code:
function add($a, $b) { return $a + $b; } $array = [1, 2, 3, 4]; $result = array_reduce($array, 'add'); var_dump($result); // 输出 10
- Anonymous function
$square = function($x) { return $x * $x; }; $result = $square(3); var_dump($result); // 输出 9Arrow function syntax can further simplify the above code:
$square = fn($x) => $x * $x; $result = $square(3); var_dump($result); // 输出 9
- Curi Currying is a technique that converts a function with multiple parameters into a function with a single parameter. In functional programming, currying can be used to reuse and simplify functions.
- In PHP, you can use closures and higher-order functions to implement currying. For example, we have a function add(x,y,z) and hope to implement a curried version:
function add($x, $y, $z) { return $x + $y + $z; } $curried_add = function($x) use ($add) { return function($y) use ($x, $add) { return function($z) use ($x, $y, $add) { return $add($x, $y, $z); }; }; }; $result = $curried_add(1)(2)(3); var_dump($result); // 输出 6Combined function
- Combined function refers to combining multiple Functions are combined together to form new functions, which can be used to simplify the code and enhance the readability and maintainability of the code.
- In PHP, you can use closures and higher-order functions to achieve function combination. For example, we have two functions $f(x)$ and $g(x)$, and want to implement a combined function $h(x)$ such that $h(x) = f(g(x))$:
function f($x) { return $x + 1; } function g($x) { return $x * 2; } $compose = function($f, $g) { return function($x) use ($f, $g) { return $f($g($x)); }; }; $h = $compose('f', 'g'); $result = $h(2); var_dump($result); // 输出 5Summary
- This article introduces how to use PHP for functional programming, including common techniques such as higher-order functions, anonymous functions, currying, and function composition. Functional programming can make code more modular, easier to test and maintain. For web developers, understanding functional programming is very valuable.
The above is the detailed content of How to do functional programming with PHP. For more information, please follow other related articles on the PHP Chinese website!
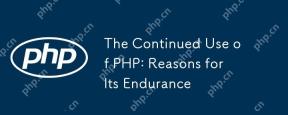
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
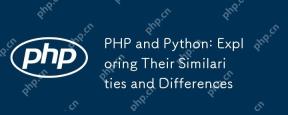
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
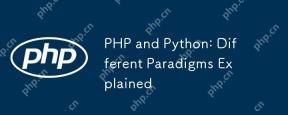
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
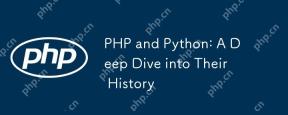
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
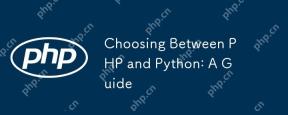
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
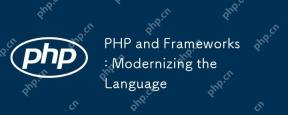
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
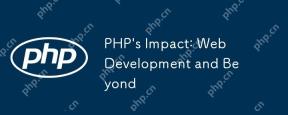
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
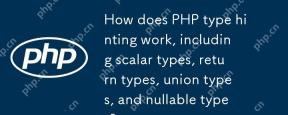
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
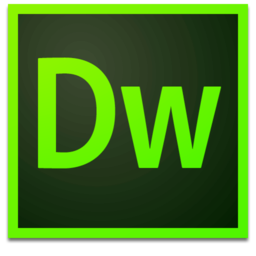
Dreamweaver Mac version
Visual web development tools
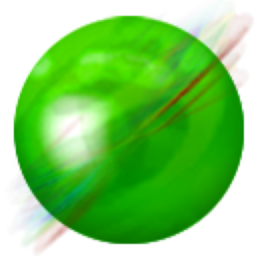
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
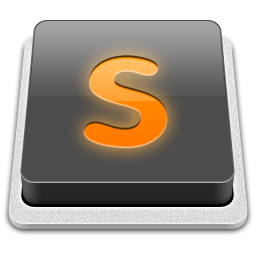
SublimeText3 Mac version
God-level code editing software (SublimeText3)