How to use PHP's socket programming function?
PHP is a programming language widely used in web development and supports many network programming applications. Among them, Socket programming is a commonly used way to implement network communication. It allows programs to implement inter-process communication and transmit data through the network.
This article will introduce how to use Socket programming function in PHP.
1. Introduction to Socket Programming
Socket (socket) is an abstract concept. It represents an open port in network communication. A process needs to connect to the port in order to Exchange data with other processes.
Sockets are generally divided into two types: TCP Socket and UDP Socket. TCP Socket is suitable for reliable connection-oriented communication, and UDP Socket is suitable for connectionless communication, applications with relatively small data packets and sensitive to delay.
2. Socket programming using PHP
In PHP, using Socket programming requires calling the socket_create() function to create a Socket connection, then setting the Socket options as needed, and finally calling socket_connect() function or socket_bind() function to further connect or bind the address and port. Finally, call the socket_close() function to disconnect.
1. Create a Socket connection
The socket_create() function can create a Socket connection.
$socket = socket_create(AF_INET, SOCK_STREAM, 0);
The first parameter specifies the address family ipv4, and the second parameter specifies the Socket type as a streaming socket (Socket stream). The third parameter is generally 0, which means using the default protocol (TCP/IP).
2. Set Socket options
You can use the socket_set_option() function to set Socket options, such as setting reuse addresses.
socket_set_option($socket, SOL_SOCKET, SO_REUSEADDR, 1);
Among them, the first parameter is the Socket connection resource, and the second parameter represents the Socket level. For example, setting the Socket option requires using SOL_SOCKET level. The third parameter specifies which option to set. Here, the SO_REUSEADDR option is set to 1Enable address reuse.
3. Connect or bind address and port
The socket_connect() function is used to connect to the remote Socket server, and the socket_bind() function is used to bind the local Socket server address and port.
For example, use the socket_bind() function to bind the local address 127.0.0.1 and port 8888.
socket_bind($socket, '127.0.0.1', 8888);
4. Listen for connection requests
Use the socket_listen() function to start listening for connection requests.
socket_listen($socket);
5. Receiving and sending data
Use the socket_accept() function to receive a connection request, and then perform data transfer operations, such as reading or writing data.
$client = socket_accept($socket); $bytes = socket_recv($client, $data, 1024, 0); socket_send($client, $data, $bytes, 0);
Among them, the socket_recv() function can be used to receive data. The first parameter is the Socket connection resource, the second parameter is used to store the received data, and the third parameter represents the maximum reading of data. Length, the fourth parameter is generally 0, which means the socket flag is not used.
The socket_send() function can be used to send data to the client.
6. Close the connection
Use the socket_close() function to close the connection.
socket_close($socket);
3. Sample code
The following is a simple complete sample code of Socket Server and Client for Socket communication.
Server side:
<?php $host = "127.0.0.1"; $port = 8888; // 创建Socket连接 $socket = socket_create(AF_INET, SOCK_STREAM, 0); // 设置Socket选项 socket_set_option($socket, SOL_SOCKET, SO_REUSEADDR, 1); // 绑定本地地址和端口 if (!socket_bind($socket, $host, $port)) { echo "Socket bind failed!"; } // 监听连接请求 socket_listen($socket); // 接受连接请求 $client = socket_accept($socket); // 读取数据 $msg = socket_read($client, 1024); // 输出接收到的数据 echo "Received message: {$msg}"; // 向Client发送数据 $response = "Message from Server: Hi Client!"; socket_write($client, $response, strlen($response)); // 关闭连接 socket_close($client); socket_close($socket); ?>
Client side:
<?php $host = "127.0.0.1"; $port = 8888; // 创建Socket连接 $socket = socket_create(AF_INET, SOCK_STREAM, 0); // 连接到Server if (!socket_connect($socket, $host, $port)) { echo "Socket connect failed!"; } // 向Server发送数据 $message = "Hello Server!"; socket_write($socket, $message, strlen($message)); // 接收Server的响应 $response = socket_read($socket, 1024); // 输出Server响应 echo "Received message: {$response}"; // 关闭连接 socket_close($socket); ?>
IV. Summary
This article introduces how to use Socket programming in PHP to achieve network communication, including Create a Socket connection, set Socket options, connect/bind Socket address and port, send/receive data, close Socket connection and other steps.
Socket programming is a very important and commonly used network programming technology, which enables processes to communicate on the network and realize data interoperability and exchange. I believe that through the introduction of this article, readers have a certain understanding and mastery of how to use Socket programming in PHP.
The above is the detailed content of How to use PHP's socket programming function?. For more information, please follow other related articles on the PHP Chinese website!
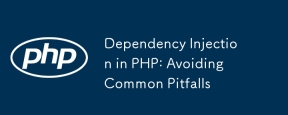
DependencyInjection(DI)inPHPenhancescodeflexibilityandtestabilitybydecouplingdependencycreationfromusage.ToimplementDIeffectively:1)UseDIcontainersjudiciouslytoavoidover-engineering.2)Avoidconstructoroverloadbylimitingdependenciestothreeorfour.3)Adhe
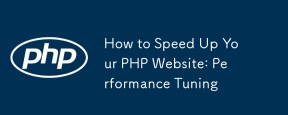
ToimproveyourPHPwebsite'sperformance,usethesestrategies:1)ImplementopcodecachingwithOPcachetospeedupscriptinterpretation.2)Optimizedatabasequeriesbyselectingonlynecessaryfields.3)UsecachingsystemslikeRedisorMemcachedtoreducedatabaseload.4)Applyasynch
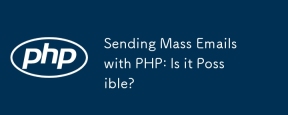
Yes,itispossibletosendmassemailswithPHP.1)UselibrarieslikePHPMailerorSwiftMailerforefficientemailsending.2)Implementdelaysbetweenemailstoavoidspamflags.3)Personalizeemailsusingdynamiccontenttoimproveengagement.4)UsequeuesystemslikeRabbitMQorRedisforb
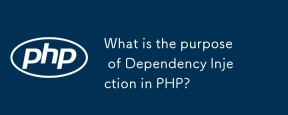
DependencyInjection(DI)inPHPisadesignpatternthatachievesInversionofControl(IoC)byallowingdependenciestobeinjectedintoclasses,enhancingmodularity,testability,andflexibility.DIdecouplesclassesfromspecificimplementations,makingcodemoremanageableandadapt
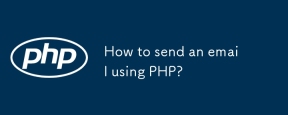
The best ways to send emails using PHP include: 1. Use PHP's mail() function to basic sending; 2. Use PHPMailer library to send more complex HTML mail; 3. Use transactional mail services such as SendGrid to improve reliability and analysis capabilities. With these methods, you can ensure that emails not only reach the inbox, but also attract recipients.
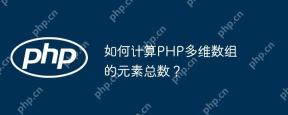
Calculating the total number of elements in a PHP multidimensional array can be done using recursive or iterative methods. 1. The recursive method counts by traversing the array and recursively processing nested arrays. 2. The iterative method uses the stack to simulate recursion to avoid depth problems. 3. The array_walk_recursive function can also be implemented, but it requires manual counting.
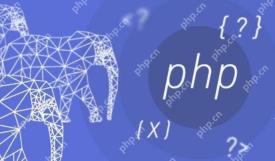
In PHP, the characteristic of a do-while loop is to ensure that the loop body is executed at least once, and then decide whether to continue the loop based on the conditions. 1) It executes the loop body before conditional checking, suitable for scenarios where operations need to be performed at least once, such as user input verification and menu systems. 2) However, the syntax of the do-while loop can cause confusion among newbies and may add unnecessary performance overhead.
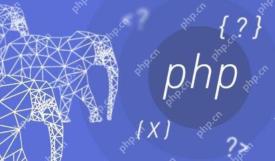
Efficient hashing strings in PHP can use the following methods: 1. Use the md5 function for fast hashing, but is not suitable for password storage. 2. Use the sha256 function to improve security. 3. Use the password_hash function to process passwords to provide the highest security and convenience.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
