Usage scenarios
In my project, there is a function provided for Autocomplete, and the amount of data is probably tens of thousands. In this article, I use the example of name retrieval to illustrate. For the list, please click on the Demo from the author of Redis.
Such a list is full of user names. For example, there is a user object in our system:
public Class User { public string Id{get; set;} public string Name {get; set;} .... public string UserHead {get; set;} }
The system needs a drop-down list of users, which cannot be done due to the large amount of data. It is displayed once, so an AutoComplete function is added. The cache can be stored directly in local memory. There is no need to use a centralized cache like Redis, so the cache structure will be simpler.
var users = new List<user>{...};//读到一个用户列表MemoryCache.Set("capqueen:users", users);//放入内存//读取var users = MemoryCache.Get<list>>("capqueen:users");</list></user>
Because they are all in memory, it is enough to store the List directly. When searching It can also be directly as follows:
var findUsers = users.Where(user => user.Name.StartWith("A")).ToList();例如输入的字符是 “A“
It is quite simple. There is no need to consider how to store and the stored data structure. However, once we move to a centralized caching service like Redis, we need to rethink how we store it.
Option 1: Similar memory-based cache implementation.
The Redis link library used in this article is StactkExchange.Redis, an open source product from StackOverFlow.
var db = redis.GetDataBase();//获取0数据库var usersJson = JsonConvert.SerializeObject(users)//序列化db.StringSet("capqueen:users", usersJson);//存储var usersString = db.StringGet("capqueen:users"); var userList = JsonConvert.DeserializeObject<list>>(users);//反序列化 </list>
There is no logical problem with the above method, and the compilation can pass. But if you think about it carefully, Redis is an independent cache service and is separated from appSever. This kind of reading method is a burden on the IO of the redis server, and even such reading is much slower than the local memory cache.
How to solve it? Just imagine that the essence of key-value lies in Key, so for List, items should be stored separately.
Option 2: Keys fuzzy matching.
After browsing the Redis command documentation (see Reference 4), he was surprised to find the Keys command, which made him immediately modify his plan. First, we need to establish the keyword to be searched as a key. Here I define the key as "capqueen:user:{id}:{name}", where the items in {} need to be replaced with the corresponding attributes of the item. The code is as follows:
var redis = ConnectionMultiplexer.Connect("localhost");var db = redis.GetDatabase(); var users = new List<user> { new User{Id = 6, Name = "aaren", Age=10}, new User{Id = 7, Name = "issy", Age=11}, new User{Id = 8, Name = "janina", Age=13}, new User{Id = 9, Name = "karena", Age=14} }; users.ForEach(item => { var key = string.Format("capqueen:user:{0}:{1}", item.Id, item.Name); var value = JsonConvert.SerializeObject(item); db.StringSet(key, value); });</user>
All users are stored in separate Key-Value methods, so how to use Keys to search? Let’s take a look at the Keys command of Redis:
KEYS pattern 查找所有符合给定模式 pattern 的 key 。 KEYS * 匹配数据库中所有 key 。 KEYS h?llo 匹配 hello , hallo 和 hxllo 等。 KEYS h*llo 匹配 hllo 和 heeeeello 等。 KEYS h[ae]llo 匹配 hello 和 hallo ,但不匹配 hillo 。 特殊符号用 \ 隔开
In other words, Keys can perform simple fuzzy matching, so our search here can be changed to the following method:
var redis = ConnectionMultiplexer.Connect("192.168.10.178");var db = redis.GetDatabase();var server = redis.GetServer("192.168.10.178", 6379);var keys = server.Keys(pattern: "capqueen:user:*:a*");var values = db.StringGet(keys.ToArray());//反序列化var jsonValues = new StringBuilder("["); values.ToList().ForEach(item => jsonValues.Append(item).Append(",")); jsonValues.Append("]");var userList = JsonConvert.DeserializeObject<list>>(jsonValues.ToString());</list>
Note the above In the code, because each value is a json, in order to increase the efficiency of conversion, I first process it into json arry and then deserialize it.
This solution indeed solved my current problem. However, I noticed a passage in the Redis document:
KEYS is very fast, but when used in a large database It may still cause performance problems. If you need to find a specific key
from a data set, you are better off using Redis's set structure (set) instead.
The above is the detailed content of How to use Redis. For more information, please follow other related articles on the PHP Chinese website!
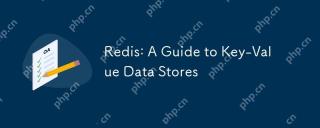
Redis is an open source memory data structure storage used as a database, cache and message broker, suitable for scenarios where fast response and high concurrency are required. 1.Redis uses memory to store data and provides microsecond read and write speed. 2. It supports a variety of data structures, such as strings, lists, collections, etc. 3. Redis realizes data persistence through RDB and AOF mechanisms. 4. Use single-threaded model and multiplexing technology to handle requests efficiently. 5. Performance optimization strategies include LRU algorithm and cluster mode.
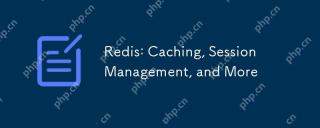
Redis's functions mainly include cache, session management and other functions: 1) The cache function stores data through memory to improve reading speed, and is suitable for high-frequency access scenarios such as e-commerce websites; 2) The session management function shares session data in a distributed system and automatically cleans it through an expiration time mechanism; 3) Other functions such as publish-subscribe mode, distributed locks and counters, suitable for real-time message push and multi-threaded systems and other scenarios.
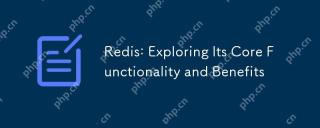
Redis's core functions include memory storage and persistence mechanisms. 1) Memory storage provides extremely fast read and write speeds, suitable for high-performance applications. 2) Persistence ensures that data is not lost through RDB and AOF, and the choice is based on application needs.
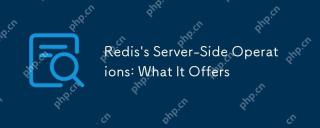
Redis'sServer-SideOperationsofferFunctionsandTriggersforexecutingcomplexoperationsontheserver.1)FunctionsallowcustomoperationsinLua,JavaScript,orRedis'sscriptinglanguage,enhancingscalabilityandmaintenance.2)Triggersenableautomaticfunctionexecutionone
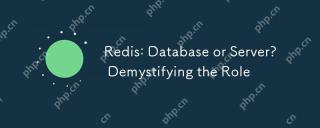
Redisisbothadatabaseandaserver.1)Asadatabase,itusesin-memorystorageforfastaccess,idealforreal-timeapplicationsandcaching.2)Asaserver,itsupportspub/submessagingandLuascriptingforreal-timecommunicationandserver-sideoperations.
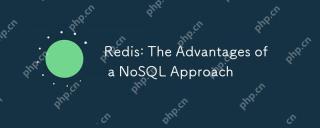
Redis is a NoSQL database that provides high performance and flexibility. 1) Store data through key-value pairs, suitable for processing large-scale data and high concurrency. 2) Memory storage and single-threaded models ensure fast read and write and atomicity. 3) Use RDB and AOF mechanisms to persist data, supporting high availability and scale-out.
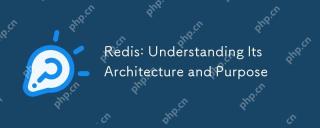
Redis is a memory data structure storage system, mainly used as a database, cache and message broker. Its core features include single-threaded model, I/O multiplexing, persistence mechanism, replication and clustering functions. Redis is commonly used in practical applications for caching, session storage, and message queues. It can significantly improve its performance by selecting the right data structure, using pipelines and transactions, and monitoring and tuning.
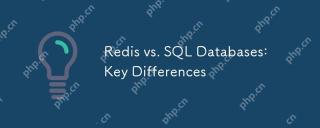
The main difference between Redis and SQL databases is that Redis is an in-memory database, suitable for high performance and flexibility requirements; SQL database is a relational database, suitable for complex queries and data consistency requirements. Specifically, 1) Redis provides high-speed data access and caching services, supports multiple data types, suitable for caching and real-time data processing; 2) SQL database manages data through a table structure, supports complex queries and transaction processing, and is suitable for scenarios such as e-commerce and financial systems that require data consistency.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version
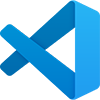
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
