Web framework and web service development in Go language
Go language has become increasingly popular in the field of web development in recent years. On the one hand, its performance and concurrency characteristics are excellent, and it is very suitable for handling highly concurrent Web requests; on the other hand, its development efficiency has gradually improved, and more and more Web frameworks and development tools have been launched.
This article will mainly introduce the related content of developing Web framework and Web services in Go language. Whether you are a beginner in web development or a developer with some experience, you can learn about the relevant knowledge and technologies of web development in Go language through this article.
1. What is a Web framework?
Web framework is a software tool designed to simplify Web application development. It usually provides functions such as processing Web requests, routing, template rendering, and database management. By using web frameworks, developers can quickly build web applications without reinventing the wheel.
In the Go language, commonly used web frameworks include Gin, Echo, Beego, etc. These frameworks provide a variety of functions that allow developers to quickly build web applications.
2. How to choose a web framework that suits you?
When choosing a Web framework, you can consider the following aspects:
(1) Performance: The performance of the framework has a crucial impact on the response speed and concurrent processing of Web applications.
(2) Coding style: Each framework has its own coding style and design ideas. Choosing a framework that suits your development style can make development smoother.
(3) Supported functions: Different frameworks usually provide different functions, such as routing, rendering templates, processing requests, database management, etc. Choosing a framework that meets your own needs can improve development efficiency.
(4) Community support: Choosing a framework with active community support can provide you with a better development experience. For example, you can get better solutions when there are problems, and you can also contribute your own code to the community to increase your influence.
3. How to use Web framework?
Using the Web framework can be divided into the following steps:
(1) Download the framework: Different frameworks have different installation methods, which can be obtained through official documents.
(2) Import the framework: Import the framework in the code, for example:
import "github.com/gin-gonic/gin"
(3) Define the route: Routing is the core part of web applications, which binds different requests with corresponding processing functions. In the Gin framework, routes can be defined in the following way:
func main() {
r := gin.Default() r.GET("/ping", func(c *gin.Context) { c.JSON(200, gin.H{ "message": "pong", }) }) r.Run() // listen and serve on 0.0.0.0:8080
}
This example defines a GET request route, requesting "/ping " will return "pong".
(4) Write a processing function: The route needs to be bound to the processing function, which will receive the request and return the response. In the Gin framework, you can write a processing function in the following way:
func main() {
r := gin.Default() r.GET("/hello/:name", func(c *gin.Context) { name := c.Param("name") c.String(http.StatusOK, "Hello %v", name) }) r.Run() // listen and serve on 0.0.0.0:8080
}
This example defines a processing function that when requesting "/ hello/xxx", returns "Hello xxx".
(5) Run the Web application: Finally, run the Web application. In the Gin framework, you can use r.Run() to start the web application, which listens to port 8080 by default.
4. What is a Web service?
Web service is a network-oriented application that provides standardized APIs, service interfaces and related protocols for data interaction through the network. With the continuous development of Internet technology, more and more Web services have emerged, such as WeChat public accounts, Alipay open platform, Baidu Maps, etc.
In the Go language, Web services can be created through the standard library net/http. This is a lightweight HTTP server that supports routing, cookies, sessions, middleware and other functions.
5. How to use net/http to create a Web service?
Creating a Web service using the standard library net/http is mainly divided into the following steps:
(1) Define the processing function: The processing function is the core part of the Web service. It will receive the request and Return response. The format of the processing function definition is as follows:
func handler(w http.ResponseWriter, r *http.Request) {
// ...
}
where, w represents a ResponseWriter interface, Can be used to write responses; r is a pointer to the http.Request structure, representing the received request.
(2) Create route: Creating route is another core part of web service, which binds requests and processing functions together. You can use the http.HandleFunc() or http.Handle() function to create a route.
(3) Create Web service: To create a Web service, you need to use the http.ListenAndServe(addr string, handler http.Handler) function. Among them, the addr parameter represents the address and port that the server monitors, and the handler parameter represents the processor of the http.Handler interface type.
The following is a simple web service example:
package main
import (
"fmt" "net/http"
)
func handler(w http .ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "Hello World!")
}
func main() {
http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil)
}
This example defines a route" /", which binds all requests to the handler() function. The http.ListenAndServe() function uses the parameter ":8080" to specify the port that the web service listens on.
6. Summary
This article introduces the relevant content of developing Web framework and Web services in Go language. By understanding the relevant knowledge and technologies of Web frameworks and Web services, you can better understand the working principles of Web development, improve your development efficiency, and lay the foundation for building high-performance concurrent Web applications.
The above is the detailed content of Web framework and web service development in Go language. For more information, please follow other related articles on the PHP Chinese website!
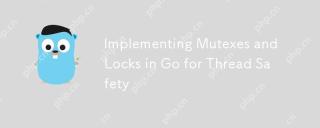
In Go, using mutexes and locks is the key to ensuring thread safety. 1) Use sync.Mutex for mutually exclusive access, 2) Use sync.RWMutex for read and write operations, 3) Use atomic operations for performance optimization. Mastering these tools and their usage skills is essential to writing efficient and reliable concurrent programs.
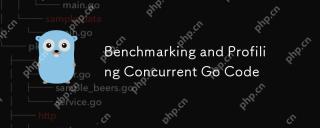
How to optimize the performance of concurrent Go code? Use Go's built-in tools such as getest, gobench, and pprof for benchmarking and performance analysis. 1) Use the testing package to write benchmarks to evaluate the execution speed of concurrent functions. 2) Use the pprof tool to perform performance analysis and identify bottlenecks in the program. 3) Adjust the garbage collection settings to reduce its impact on performance. 4) Optimize channel operation and limit the number of goroutines to improve efficiency. Through continuous benchmarking and performance analysis, the performance of concurrent Go code can be effectively improved.
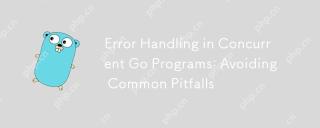
The common pitfalls of error handling in concurrent Go programs include: 1. Ensure error propagation, 2. Processing timeout, 3. Aggregation errors, 4. Use context management, 5. Error wrapping, 6. Logging, 7. Testing. These strategies help to effectively handle errors in concurrent environments.
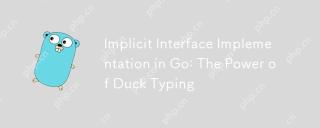
ImplicitinterfaceimplementationinGoembodiesducktypingbyallowingtypestosatisfyinterfaceswithoutexplicitdeclaration.1)Itpromotesflexibilityandmodularitybyfocusingonbehavior.2)Challengesincludeupdatingmethodsignaturesandtrackingimplementations.3)Toolsli
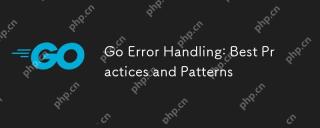
In Go programming, ways to effectively manage errors include: 1) using error values instead of exceptions, 2) using error wrapping techniques, 3) defining custom error types, 4) reusing error values for performance, 5) using panic and recovery with caution, 6) ensuring that error messages are clear and consistent, 7) recording error handling strategies, 8) treating errors as first-class citizens, 9) using error channels to handle asynchronous errors. These practices and patterns help write more robust, maintainable and efficient code.
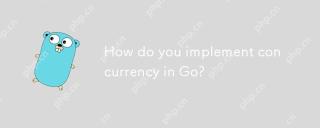
Implementing concurrency in Go can be achieved by using goroutines and channels. 1) Use goroutines to perform tasks in parallel, such as enjoying music and observing friends at the same time in the example. 2) Securely transfer data between goroutines through channels, such as producer and consumer models. 3) Avoid excessive use of goroutines and deadlocks, and design the system reasonably to optimize concurrent programs.
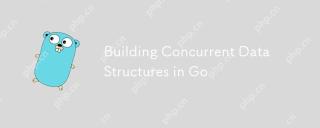
Gooffersmultipleapproachesforbuildingconcurrentdatastructures,includingmutexes,channels,andatomicoperations.1)Mutexesprovidesimplethreadsafetybutcancauseperformancebottlenecks.2)Channelsofferscalabilitybutmayblockiffullorempty.3)Atomicoperationsareef
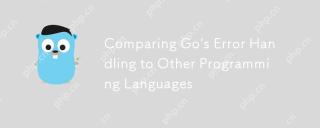
Go'serrorhandlingisexplicit,treatingerrorsasreturnedvaluesratherthanexceptions,unlikePythonandJava.1)Go'sapproachensureserrorawarenessbutcanleadtoverbosecode.2)PythonandJavauseexceptionsforcleanercodebutmaymisserrors.3)Go'smethodpromotesrobustnessand


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Zend Studio 13.0.1
Powerful PHP integrated development environment
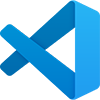
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
