With the rise of the Go language, the ORM (Object Relational Mapping) framework has become more and more important. ORM frameworks can be used to store and retrieve data in databases, which in turn provides applications with an efficient way to access this data. In this article, we will learn about the ORM framework in the Go language and master how to use the ORM framework to improve the performance of your application.
ORM framework in Go language
In Go language, there are many ORM frameworks to choose from. One of the most popular frameworks is GORM. GORM is an open source framework that provides a simple way to interact with databases and supports a variety of relational databases, such as MySQL, PostgreSQL, SQLite, etc. GORM also supports many common ORM functions, such as model association, transactions, preloading and migration, etc.
Another ORM framework worth mentioning is Xorm, which is also an open source framework. Similar to GORM, it supports a variety of relational databases and provides common ORM functions. There are other ORM frameworks, such as QBS and Beego ORM, which all provide ORM functionality to a certain extent.
However, compared with other programming languages, Go language has relatively few ORM frameworks. Therefore, when choosing an ORM framework, you need to carefully consider the availability, maintenance, documentation, and support of the framework.
Using the ORM framework for database operations
Below, we will see how to use the GORM framework for common database operations.
First, we need to install the GORM framework. You can use the following command to install:
go get -u gorm.io/gorm
After installation, we can start using the GORM framework. The following are some common operations:
Establish a connection
import ( "gorm.io/driver/mysql" "gorm.io/gorm" ) func main() { dsn := "user:password@tcp(host:port)/database?charset=utf8mb4&parseTime=True&loc=Local" db, err := gorm.Open(mysql.Open(dsn), &gorm.Config{}) if err != nil { panic(err) } defer db.Close() }
Create table
db.AutoMigrate(&User{})
Add data
user := User{Name: "Jinzhu", Age: 18, Birthday: time.Now()} db.Create(&user)
Get data
var user User db.First(&user, 1) // 根据整形主键查找 db.First(&user, "name = ?", "Jinzhu") // 根据查询条件查找
Update Data
db.Model(&user).Update("name", "Gopher")
Delete data
db.Delete(&user)
Conclusion
The ORM framework provides a simple and convenient way for database operations in the Go language. However, there are some disadvantages to using an ORM framework. ORM frameworks may incur a certain performance loss and may abstract the underlying details of the database, making it difficult for developers to maintain efficient queries. Additionally, some advanced operations may require manually writing raw SQL queries.
Therefore, you should carefully consider your own needs and the performance requirements of your application before choosing an ORM framework. At the same time, you should also learn how to optimize database operations through raw SQL queries so that you can better use the ORM framework when needed.
The above is the detailed content of Tips for using the ORM framework in Go language. For more information, please follow other related articles on the PHP Chinese website!
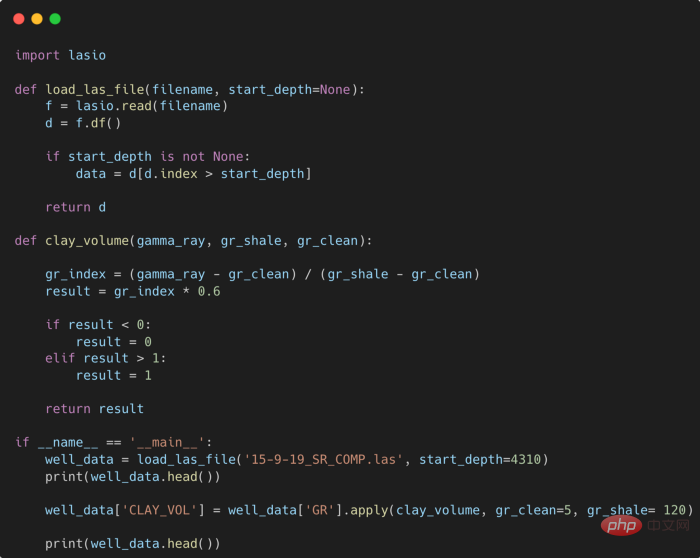
Python 中有许多方法可以帮助我们理解代码的内部工作原理,良好的编程习惯,可以使我们的工作事半功倍!例如,我们最终可能会得到看起来很像下图中的代码。虽然不是最糟糕的,但是,我们需要扩展一些事情,例如:load_las_file 函数中的 f 和 d 代表什么?为什么我们要在 clay 函数中检查结果?这些函数需要什么类型?Floats? DataFrames?在本文中,我们将着重讨论如何通过文档、提示输入和正确的变量名称来提高应用程序/脚本的可读性的五个基本技巧。1. Comments我们可
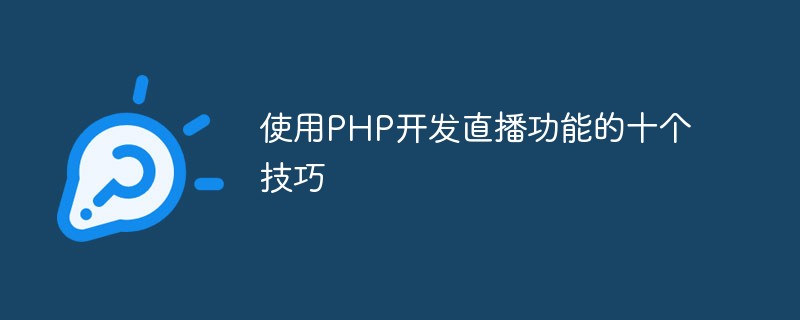
随着直播业务的火爆,越来越多的网站和应用开始加入直播这项功能。PHP作为一种流行的服务器端语言,也可以用来开发高效的直播功能。当然,要实现一个稳定、高效的直播功能需要考虑很多问题。下面列出了使用PHP开发直播功能的十个技巧,帮助你更好地实现直播。选择合适的流媒体服务器PHP开发直播功能,首先需要考虑的就是流媒体服务器的选择。有很多流媒体服务器可以选择,比如常
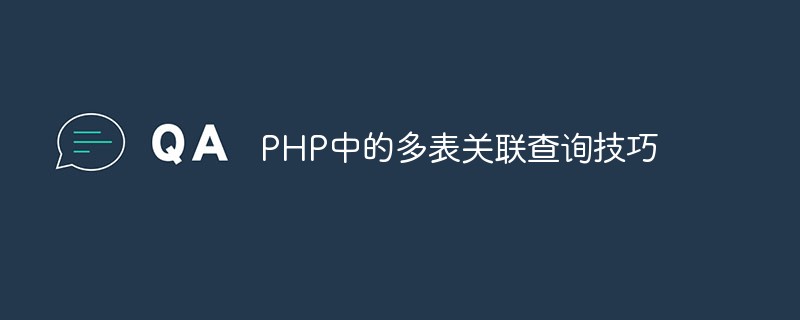
PHP中的多表关联查询技巧关联查询是数据库查询的重要部分,特别是当你需要展示多个相关数据库表内的数据时。在PHP应用程序中,在使用MySQL等数据库时,多表关联查询经常会用到。多表关联的含义是,将一个表中的数据与另一个或多个表中的数据进行比较,在结果中将那些满足要求的行连接起来。在进行多表关联查询时,需要考虑表之间的关系,并使用合适的关联方法。下面介绍几种多
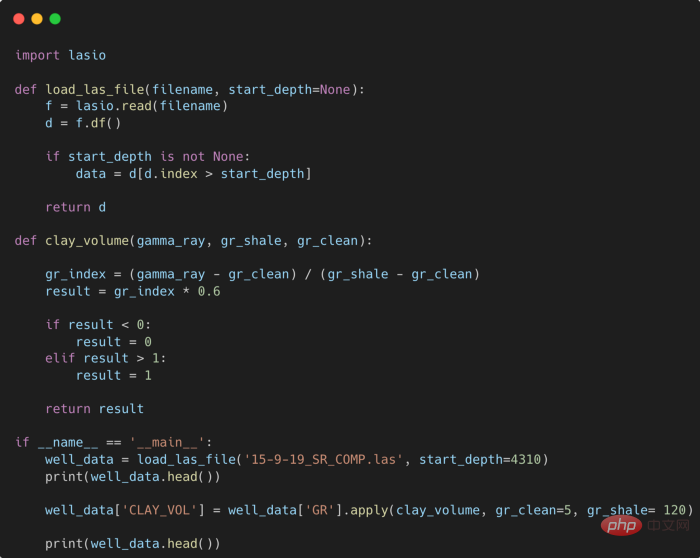
译者 | 赵青窕审校 | 孙淑娟你是否经常回头看看6个月前写的代码,想知道这段代码底是怎么回事?或者从别人手上接手项目,并且不知道从哪里开始?这样的情况对开发者来说是比较常见的。Python中有许多方法可以帮助我们理解代码的内部工作方式,因此当您从头来看代码或者写代码时,应该会更容易地从停止的地方继续下去。在此我给大家举个例子,我们可能会得到如下图所示的代码。这还不是最糟糕的,但有一些事情需要我们去确认,例如:在load_las_file函数中f和d代表什么?为什么我们要在clay函数中检查结果
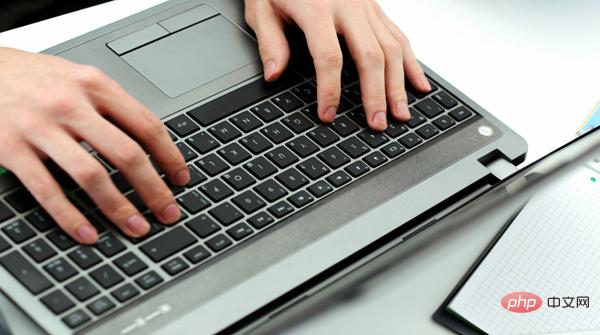
1.简介我们在日常使用Python进行各种数据计算处理任务时,若想要获得明显的计算加速效果,最简单明了的方式就是想办法将默认运行在单个进程上的任务,扩展到使用多进程或多线程的方式执行。而对于我们这些从事数据分析工作的人员而言,以最简单的方式实现等价的加速运算的效果尤为重要,从而避免将时间过多花费在编写程序上。而今天的文章费老师我就来带大家学习如何利用joblib这个非常简单易用的库中的相关功能,来快速实现并行计算加速效果。2.使用joblib进行并行计算作为一个被广泛使用的第三方Python库(
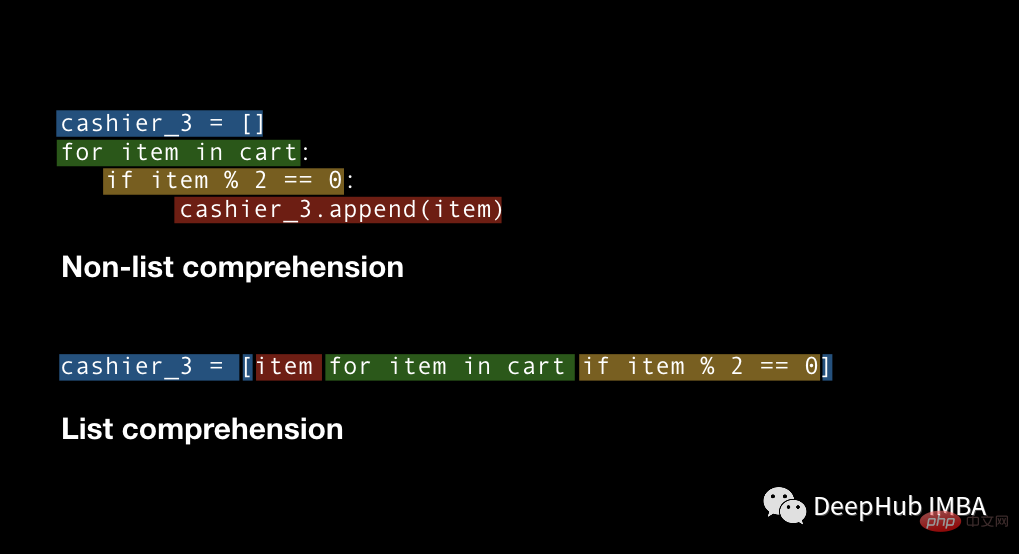
对于数据科学,Python通常被广泛地用于进行数据的处理和转换,它提供了强大的数据结构处理的函数,使数据处理更加灵活,这里说的“灵活性”是什么意思?这意味着在Python中总是有多种方法来实现相同的结果,我们总是有不同的方法并且需要从中选择易于使用、省时并能更好控制的方法。要掌握所有的这些方法是不可能的。所以这里列出了在处理任何类型的数据时应该知道的4个Python技巧。列表推导式ListComprehension是创建列表的一种优雅且最符合python语言的方法。与for循环和if语句相比,列
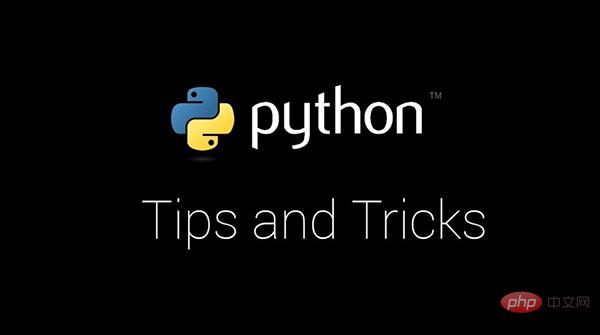
整理字符串输入整理用户输入的问题在编程过程中极为常见。通常情况下,将字符转换为小写或大写就够了,有时你可以使用正则表达式模块「Regex」完成这项工作。但是如果问题很复杂,可能有更好的方法来解决:user_input="Thisnstringhastsomewhitespaces...rn"character_map={ord('n'):'',ord('t'):'',ord('r'):None}user_input.translate(charact
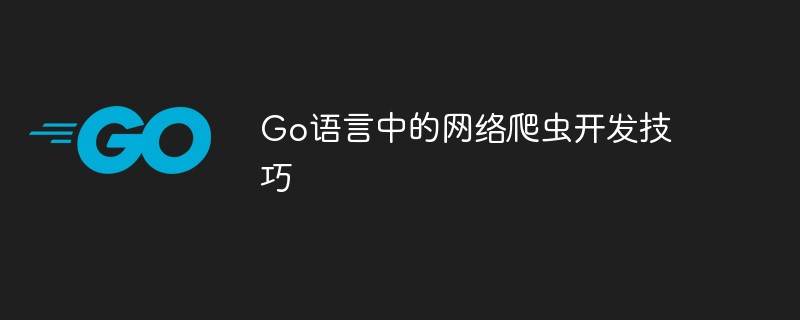
近年来,随着网络信息的急剧增长,网络爬虫技术在互联网行业中扮演着越来越重要的角色。其中,Go语言的出现为网络爬虫的开发带来了诸多优势,如高速度、高并发、低内存占用等。本文将介绍一些Go语言中的网络爬虫开发技巧,帮助开发者更快更好地进行网络爬虫项目开发。一、如何选择合适的HTTP客户端在Go语言中,有多种HTTP请求库可供选择,如net/http、GoRequ


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
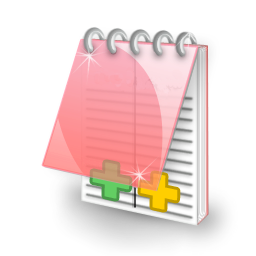
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
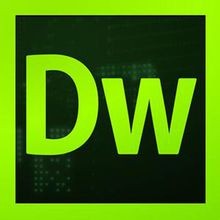
Dreamweaver CS6
Visual web development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
