With the rapid development of the Internet, computer programming is becoming more and more important. When writing code, many times we encounter various problems. Debugging is a necessary process for writing code. Through debugging, we can find errors in the code and solve them. Go language is an emerging programming language that has the advantages of simplicity, speed and efficiency, and also has many powerful debugging techniques and tools. In this article, we will introduce debugging techniques and tool applications in the Go language to help you debug faster and better.
1. Print debugging information
When debugging code, the simplest way is to print debugging information. The Go language has a fmt package that can be used to print strings in various formats. By using the fmt package, you can print variables, constants, expressions and other information at runtime to understand the execution of the program. The following is an example:
package main import "fmt" func main() { fmt.Println("Start of the program") x := 2 fmt.Printf("x = %d ", x) y := 3 fmt.Printf("y = %d ", y) z := x + y fmt.Printf("z = %d ", z) fmt.Println("End of the program") }
Through the above code, we can see the running process of the program on the console to better understand the code execution process. During the actual debugging process, you can use more functions provided by the fmt package, such as Sprintf, Printf, etc., to print debugging information more flexibly. It should be noted that after debugging is completed, all printed debugging information should be deleted to avoid leaking sensitive information.
2. Debugging breakpoints
In addition to printing debugging information, debugging breakpoints are also a very important debugging tool. Debugging breakpoints pause the program when it reaches a specific line of code so that you can examine program status and variable values. In Go language, you can use debuggers such as delve and GDB to implement debugging breakpoints. delve is an open source command line debugger that supports syntax and data types in the Go language and is very easy to use. The following is a simple example:
package main func main() { x := 2 y := 3 z := x + y println(z) }
In the above code, we define three variables x, y and z, and add them and output. If you want to pause program execution when it reaches the addition statement, you can use delve to set a debugging breakpoint. First, you need to enter the following command on the command line to install delve:
go get -u github.com/go-delve/delve/cmd/dlv
After the installation is complete, you can add breakpoints in the program. Run the following command in the console:
dlv debug main.go
When the program executes the addition statement, delve will automatically pause the program. You can use the command-line input interface to inspect variables, modify variable values, and even step into programs. This tool is very powerful and it is recommended that you learn more about its usage.
3. Performance Analysis
Performance is an important indicator. Through performance analysis and optimization of the program, we can make the program run faster. The Go language provides many tools to help you analyze the performance of your program. Among them, the most commonly used is pprof.
pprof is an open source performance analysis tool that supports multiple performance analysis methods, such as CPU analysis, memory analysis, etc., and can output the analysis results to files to facilitate subsequent analysis and optimization. Here is an example:
package main import ( "fmt" "math/rand" "time" ) func main() { rand.Seed(time.Now().UnixNano()) work := make([]int, 1000000) l := len(work) for i := 0; i < l; i++ { work[i] = rand.Intn(10) } sum := int64(0) for _, v := range work { sum += int64(v) } fmt.Println("Sum:", sum) }
In the above code, we generated 1,000,000 random numbers and found their sum. If you want to analyze the CPU performance of the program, you can use the following command:
go run -cpuprofile=cpu.out main.go go tool pprof cpu.out (pprof) web
After executing the above command, pprof will automatically open a WEB interface through which you can view the performance bottleneck and call stack of the program. and other information, and optimize based on this information. It should be noted that you need to install the Graphviz tool to use pprof's web functionality. You can install Graphviz through the following command:
sudo apt-get install graphviz
In addition to pprof, the Go language also provides many other tools, such as trace, traceview, etc., that can help you better conduct performance analysis.
Summary
Debugging is a necessary process of writing code, which can help us find errors in the code and solve them. In the Go language, there are many powerful debugging techniques and tools, such as printing debugging information, debugging breakpoints, performance analysis, etc. By learning these tips and tools, you can debug faster and better, making your programs more robust and efficient. It is recommended that you include the debugging process when writing code and use appropriate debugging tools to ensure the quality and stability of the code.
The above is the detailed content of Debugging skills and tool applications in Go language. For more information, please follow other related articles on the PHP Chinese website!
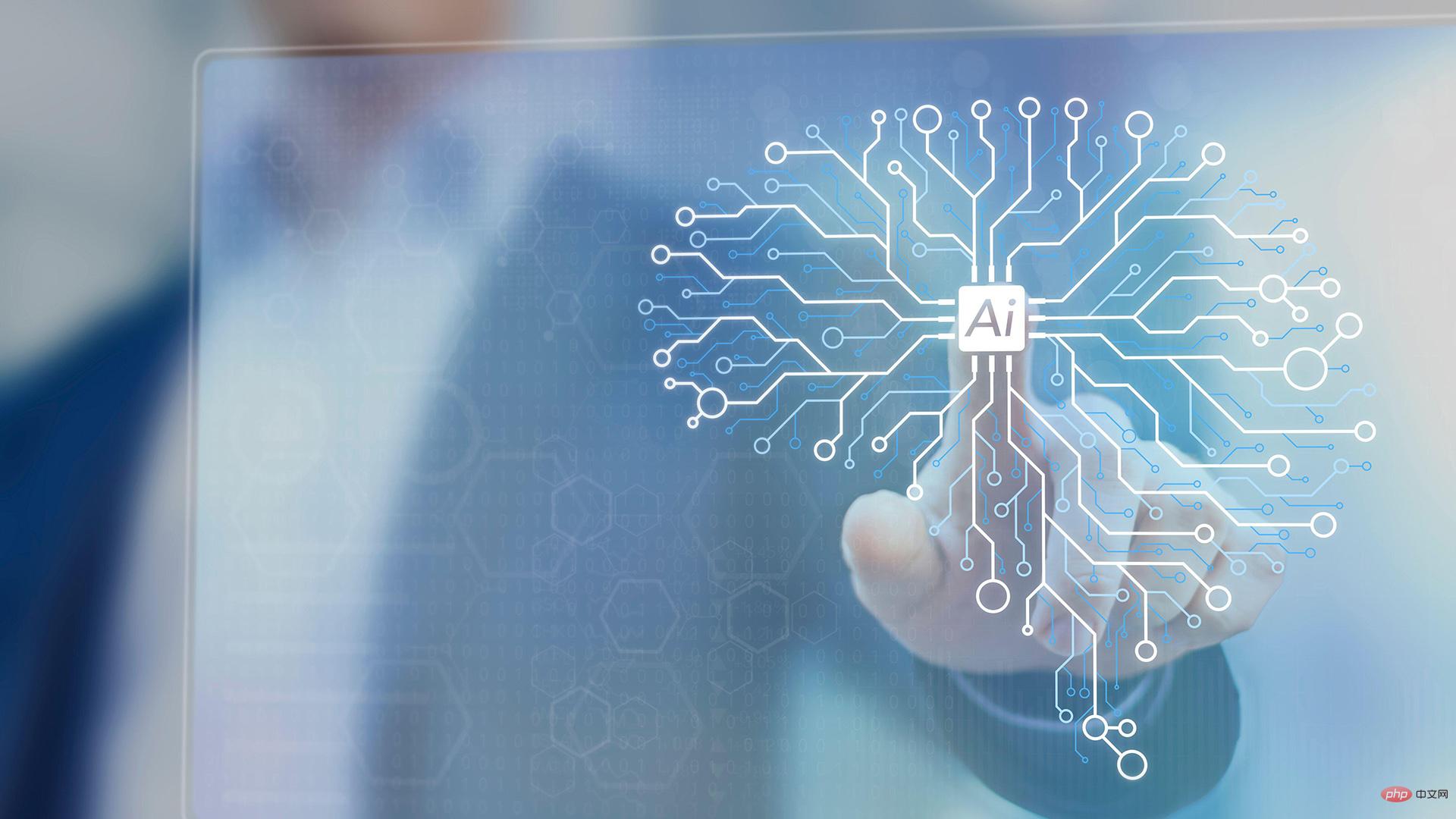
随着互联网技术和人工智能的发展,越来越多的内容创作者开始采用各种AI工具来提高创作效率和质量。本文将介绍8个最流行的AI工具,它们可以帮助你轻松实现10倍的效率提升,让你更快地完成内容创作任务,同时保证内容的高质量和创意。Chatsonic一个类似chatgpt的聊天机器人,具有实时数据、图像、语音搜索等功能。专门为内容创作者设计的AI聊天机器人来提升你的生产力。网址:https://writesonic.com/chatMidjourney一个由人工智能驱动的系统,根据用户输入的提示创建图像。
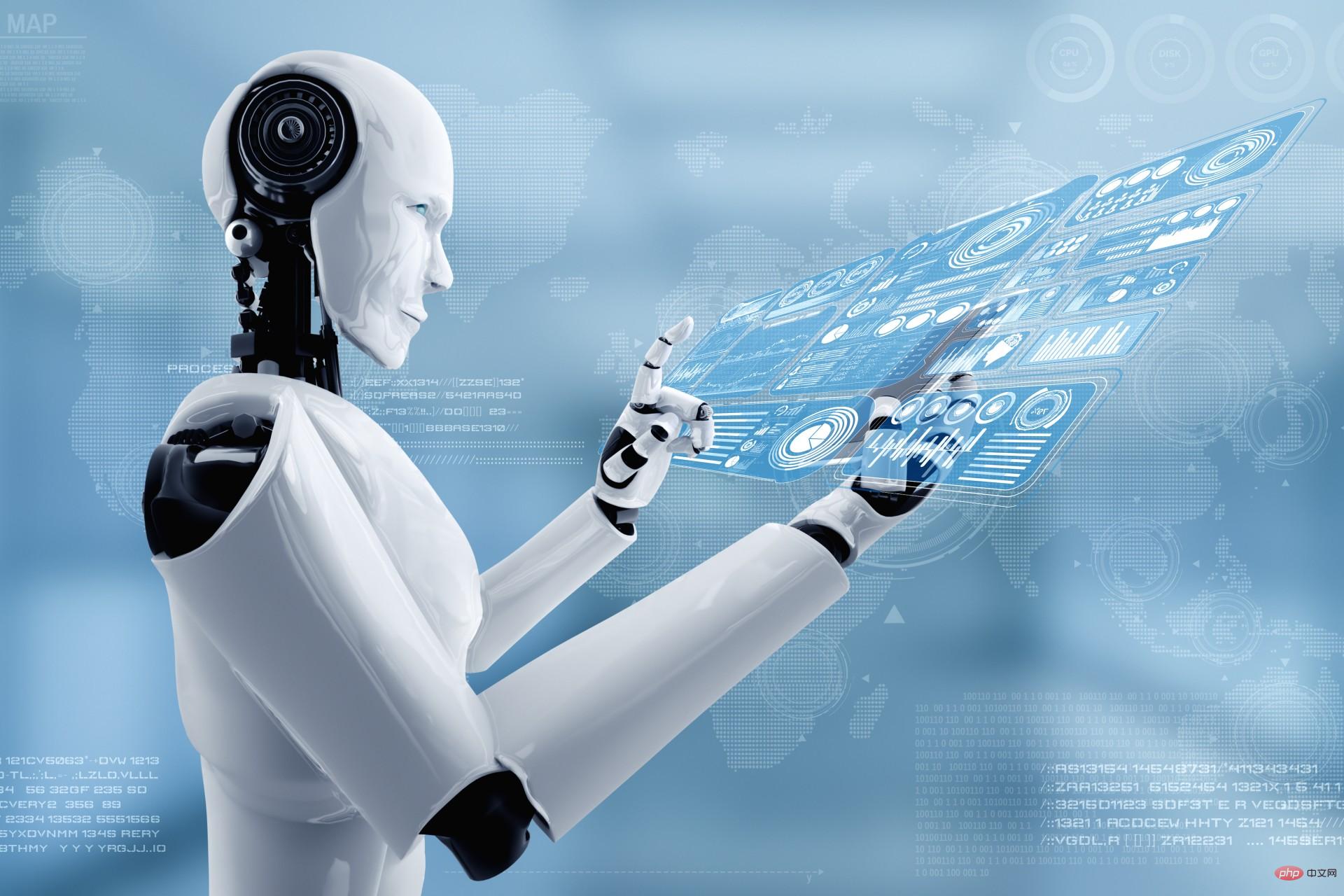
ChatGPT 之前几十年来,人工智能 (AI) 一直在改变我们的生活和工作方式。从使用 AI 驱动的虚拟助手改善我们的个人生活,到通过智能自动化彻底改变整个行业,AI 一次又一次地证明了它的价值。但在 ChatGPT 之前,AI 过去常常执行特定的小任务,很少有人认真对待它。ChatGPT 之后有了 ChatGPT,世界变得疯狂了。就那么几天之内,人们都在谈论这种令人兴奋的语言模型的强大功能。重点突然转移到基于人工智能的工具上,越来越多的人开始使用这些基于人工智能的工具,从那时起,更多工具应运
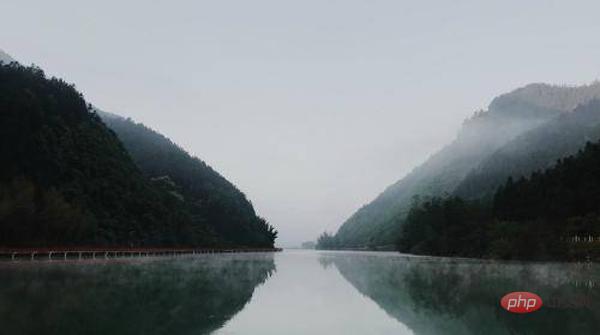
糟透了我承认我不是一个爱整理桌面的人,因为我觉得乱糟糟的桌面,反而容易找到文件。哈哈,可是最近桌面实在是太乱了,自己都看不下去了,几乎占满了整个屏幕。虽然一键整理桌面的软件很多,但是对于其他路径下的文件,我同样需要整理,于是我想到使用Python,完成这个需求。效果展示我一共为将文件分为9个大类,分别是图片、视频、音频、文档、压缩文件、常用格式、程序脚本、可执行程序和字体文件。# 不同文件组成的嵌套字典 file_dict = { '图片': ['jpg','png','gif','webp
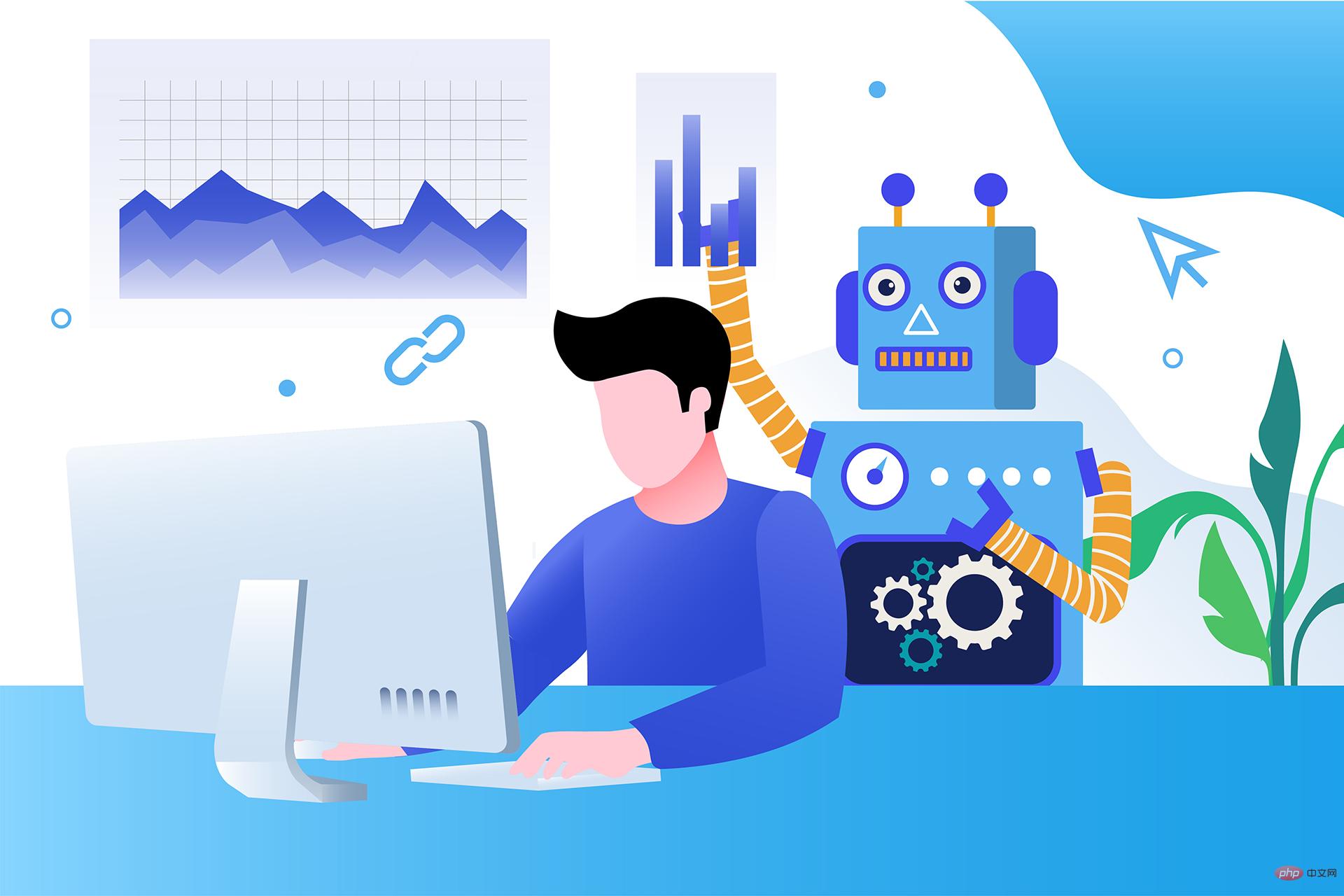
自 2020 年以来,内容开发领域已经感受到人工智能工具的存在。1.Jasper AI网址:https://www.jasper.ai在可用的 AI 文案写作工具中,Jasper 作为那些寻求通过内容生成赚钱的人来讲,它是经济实惠且高效的选择之一。该工具精通短格式和长格式内容均能完成。Jasper 拥有一系列功能,包括无需切换到模板即可快速生成内容的命令、用于创建文章的高效长格式编辑器,以及包含有助于创建各种类型内容的向导的内容工作流,例如,博客文章、销售文案和重写。Jasper Chat 是该
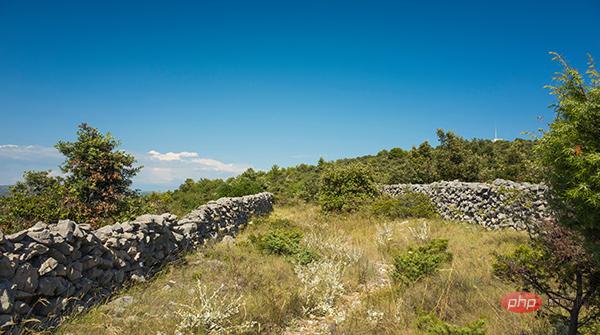
大家好,我是Python人工智能技术喜欢用 Python 做项目的小伙伴不免会遇到这种情况:做图表时,用哪种好看又实用的可视化工具包呢?之前文章里出现过漂亮的图表时,也总有读者在后台留言问该图表时用什么工具做的。下面,作者介绍了八种在 Python 中实现的可视化工具包,其中有些包还能用在其它语言中。快来试试你喜欢哪个?用 Python 创建图形的方法有很多,但是哪种方法是最好的呢?当我们做可视化之前,要先明确一些关于图像目标的问题:你是想初步了解数据的分布情况?想展示时给人们留下深刻印象?也许
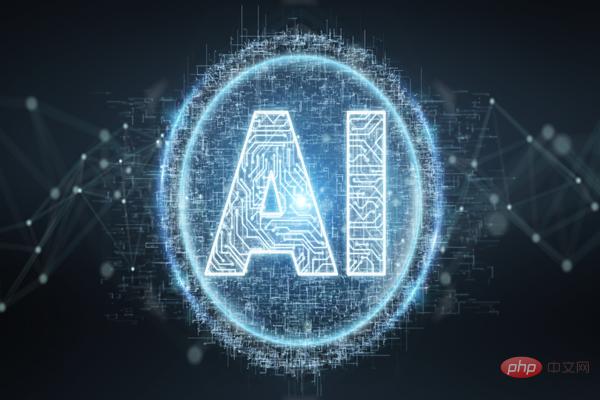
我们非常接近 2023 年,我们都希望在新的一年里基于 AI 的工具会出现爆炸式增长,这是有充分理由的。如果像我一样,你是这些技术的忠实粉丝,以及它们如何将我们的生产力提高 10 倍,你可以在这篇文章中找到该领域的 7 种工具列表。您知道吗,您可以在DoTenX上免费实施带有或不带有编码的网络应用程序、API、网站或登录页面?请务必检查一下,甚至提名您的作品进行展示。DoTenX 是开源的,您可以在此处找到存储库:github.com/dotenx/dotenx。现在,让我们来看看我们的列表。
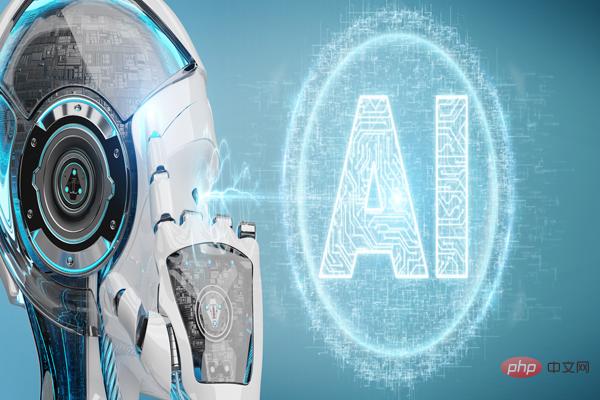
Instagram正在测试用户验证年龄的新方法,包括由第三方公司Yoti开发的一款人工智能工具,它可以通过扫描你的脸来估计你的年龄。按照官方规定,必须年满13岁才能注册Instagram账户。但多年来,该公司几乎没有努力执行这一规定。它甚至都懒得问新用户的生日,更不用说核实这些信息了。然而,直到2019年遭到隐私和儿童安全专家的猛烈抨击之后,Instagram推出了越来越多的年龄验证功能,以及将年轻用户与成年用户区分开来的方法。目前,在青少年试图修改自己的出生日期,显示自己年满18岁时Inst
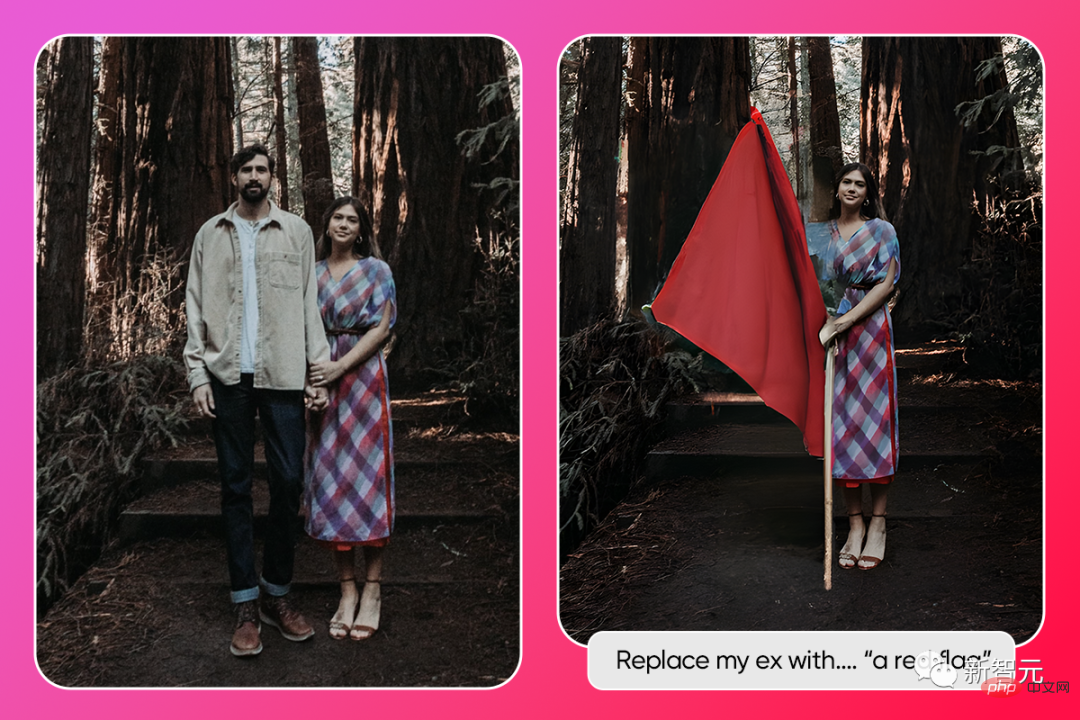
我们都有过这样的经历:你有一张看起来超级棒的照片,但它被一个已经不在你生活中的人的存在所玷污。你不想看到或想到他们,但又不一定想删除你们在一起的数百(甚至数千)张照片。现在,一家名叫Picsart的照片和视频编辑公司,就推出了一个由AI驱动的全新功能——「AI帮我换前任」(AI Replace My Ex)。官方表示,无论是你的前男友、前女友还是前朋友,AI Replace都可以让你用几乎任何你能想到的东西替换照片中的人。操作超级简单,不需要任何技巧,只需几秒钟就能完成。(如此神乎其技?多半是吹


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
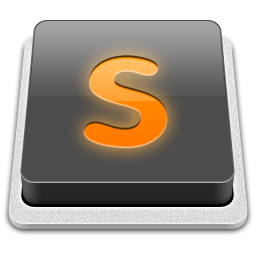
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
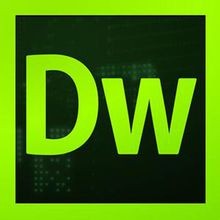
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
