In web development, JavaScript is a powerful scripting language that can make web pages more dynamic and interactive. In many cases, developers need to check whether a piece of text contains repeated characters. This article will explain how to use JavaScript to achieve this goal and avoid repeated characters.
- Using the Set object
Set is a new data structure in ES6. It is similar to an array, but the elements in the Set are not repeated. You can convert the text into a character array, then convert the character array into a Set object, and find whether the elements are repeated in the Set object.
The following is an example of using a Set object to check whether there are repeated characters in the text:
function checkDuplicates(text) { var charArray = text.split(''); var charSet = new Set(charArray); return charSet.size !== charArray.length; } var textWithDuplicates = "aabbccdd"; var textWithoutDuplicates = "abcd"; console.log(checkDuplicates(textWithDuplicates)); // true console.log(checkDuplicates(textWithoutDuplicates)); // false
First use the split
method to convert the text into a character array, and then create a Set Object, the Set object consists of elements in the character array. Finally, use the size
property of the Set object to check whether the number of elements in the Set object is equal to the length of the character array. If this condition is not true, it means that there are repeated characters in the text.
- Using Object Properties
Another way is to check if there are duplicate characters in the text by using an object. You can create an empty object named charMap
and iterate over the character array, store the characters as property names of the object, and set their values to true. Before adding the next character, you need to check if the object property already exists. If present, it means that the character already exists in the text and is therefore a duplicate.
The following is an example of using object properties to check whether there are repeated characters in the text:
function checkDuplicates(text) { var charArray = text.split(''); var charMap = {}; for (var i = 0; i < charArray.length; i++) { var currentChar = charArray[i]; if (charMap[currentChar]) { return true; } charMap[currentChar] = true; } return false; } var textWithDuplicates = "aabbccdd"; var textWithoutDuplicates = "abcd"; console.log(checkDuplicates(textWithDuplicates)); // true console.log(checkDuplicates(textWithoutDuplicates)); // false
First use the split
method to convert the text into a character array, and then create an empty ObjectcharMap
. Next, use for
to loop through the character array and check whether the current character already exists in the charMap
object. If it exists, the character is repeated. If the character is not present, it is used as a property name of the object and its value is set to true
. Finally, if no repeated characters are found after the loop ends, false
is returned.
It should be noted that when using object properties as property names, the properties will be automatically converted into strings. Therefore, if you do not use the split
method to convert to a character array, but directly use text as input, it may cause some unpredictable problems.
To sum up, this article introduces two methods to use JavaScript to check whether there are repeated characters in the text. By using Set objects or object properties, developers can avoid duplicate characters and improve the performance and reliability of web applications.
The above is the detailed content of javascript does not repeat characters. For more information, please follow other related articles on the PHP Chinese website!

The use of class selectors and ID selectors depends on the specific use case: 1) Class selectors are suitable for multi-element, reusable styles, and 2) ID selectors are suitable for unique elements and specific styles. Class selectors are more flexible, ID selectors are faster to process but may affect code maintenance.

ThekeygoalsandmotivationsbehindHTML5weretoenhancesemanticstructure,improvemultimediasupport,andensurebetterperformanceandcompatibilityacrossdevices,drivenbytheneedtoaddressHTML4'slimitationsandmeetmodernwebdemands.1)HTML5aimedtoimprovesemanticstructu

IDsareuniqueandusedforsingleelements,whileclassesarereusableformultipleelements.1)UseIDsforuniqueelementslikeaspecificheader.2)Useclassesforconsistentstylingacrossmultipleelementslikebuttons.3)BecautiouswithspecificityasIDsoverrideclasses.4)Useclasse

HTML5aimstoenhancewebaccessibility,interactivity,andefficiency.1)Itsupportsmultimediawithoutplugins,simplifyinguserexperience.2)Semanticmarkupimprovesstructureandaccessibility.3)Enhancedformhandlingincreasesusability.4)Thecanvaselementenablesdynamicg

HTML5isnotparticularlydifficulttousebutrequiresunderstandingitsfeatures.1)Semanticelementslike,,,andimprovestructure,readability,SEO,andaccessibility.2)Multimediasupportviaandelementsenhancesuserexperiencewithoutplugins.3)Theelementenablesdynamic2Dgr

No,youshouldn'tusemultipleIDsinthesameDOM.1)IDsmustbeuniqueperHTMLspecification,andusingduplicatescancauseinconsistentbrowserbehavior.2)Useclassesforstylingmultipleelements,attributeselectorsfortargetingbyattributes,anddescendantselectorsforstructure

HTML5aimstoenhancewebcapabilities,makingitmoredynamic,interactive,andaccessible.1)Itsupportsmultimediaelementslikeand,eliminatingtheneedforplugins.2)Semanticelementsimproveaccessibilityandcodereadability.3)Featureslikeenablepowerful,responsivewebappl

HTML5aimstoenhancewebdevelopmentanduserexperiencethroughsemanticstructure,multimediaintegration,andperformanceimprovements.1)Semanticelementslike,,,andimprovereadabilityandaccessibility.2)andtagsallowseamlessmultimediaembeddingwithoutplugins.3)Featur


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
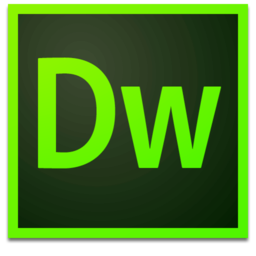
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!
