Laravel is a popular PHP framework that provides convenient tools and features to help developers quickly build web applications. One of the basic functions is to use tables to add, delete, modify and query data. This article will introduce how to implement these functions in Laravel.
- Create database and table
First, we need to create a database and a data table to store data. In this article, we will create a table called "users" that contains the following fields: id, name, email, and password.
We can use migrations in Laravel to create tables. Run the following command in the command line:
php artisan make:migration create_users_table --create=users
After running this command, Laravel will create a new migration file in the "database/migrations" directory. We can use the "Schema" class in the migration file to define the table structure. The code is as follows:
use IlluminateDatabaseMigrationsMigration; use IlluminateDatabaseSchemaBlueprint; use IlluminateSupportFacadesSchema; class CreateUsersTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('users', function (Blueprint $table) { $table->increments('id'); $table->string('name'); $table->string('email')->unique(); $table->string('password'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('users'); } }
The above code creates a table named "users", which contains the id, name, email, password and timestamps fields.
When we run the migration, Laravel will create the table in the database. Run the following command to migrate:
php artisan migrate
- Add records
To add records to the table, we need to create a controller and use a model to save the data to the database . Run the following command in the command line:
php artisan make:controller UserController
This command will create a new "UserController" controller in the "app/Http/Controllers" directory.
In the controller file, we can use the "create" method to save new records. The following is the sample code:
use AppUser; use IlluminateHttpRequest; class UserController extends Controller { public function store(Request $request) { $user = User::create([ 'name' => $request->input('name'), 'email' => $request->input('email'), 'password' => bcrypt($request->input('password')), ]); return response()->json([ 'message' => 'User created successfully', 'user' => $user, ]); } }
In the above code, we first import the "User" model and then use the "create" method in the "store" method to create a new record. We use the "name", "email" and "password" fields from the request as parameters and encrypt the password using the "bcrypt" function. Finally, we return a JSON response containing the created user record.
- List Records
We can use a controller to get all the records in the table and return them to the user. Add the following code in the controller:
public function index() { $users = User::all(); return view('users.index', ['users' => $users]); }
The above code gets all the records in the user table by using the "all" method and passes the results to the view. We can use this data in the view to render the HTML table.
- Update records
Similar to when creating records, we can use models and controllers to update records. Here is an example "update" method:
public function update(Request $request, $id) { $user = User::find($id); $user->name = $request->input('name'); $user->email = $request->input('email'); if ($request->input('password')) { $user->password = bcrypt($request->input('password')); } $user->save(); return response()->json([ 'message' => 'User updated successfully', 'user' => $user, ]); }
In the above code, we first use the "find" method to get the user record with the specified ID. We then use the "name" and "email" fields from the request as attribute values to update the record. If the request contains a "password" field, it is encrypted using the "bcrypt" function and the user record is updated. Finally, we save the record using the "save" method and return the updated user record as a JSON response.
- Delete records
We can use controllers and models to delete user records. Here is an example of the "destroy" method:
public function destroy($id) { $user = User::find($id); $user->delete(); return response()->json([ 'message' => 'User deleted successfully', ]); }
In the above code, we first use the "find" method to get the user record with the specified ID. We can then perform other operations on the record before deleting it using the "delete" method. Finally, we return a JSON response containing information about the deleted user.
- Table View
For the case of listing all user records, we can use a view to render an HTML table. Here is a sample view code:
<table> <thead> <tr> <th>ID</th> <th>Name</th> <th>Email</th> <th>Action</th> </tr> </thead> <tbody> @foreach ($users as $user) <tr> <td>{{ $user->id }}</td> <td>{{ $user->name }}</td> <td>{{ $user->email }}</td> <td> <a href="{{ route('users.edit', $user->id) }}">Edit</a> <form action="{{ route('users.destroy', $user->id) }}" method="POST"> {{ csrf_field() }} {{ method_field('DELETE') }} <button type="submit">Delete</button> </form> </td> </tr> @endforeach </tbody> </table>
In the above code, we are using "@foreach" directive to loop through all the user records and display their ID, name and email in an HTML table. Additionally, we added two action columns: Edit and Delete. For deletion, we use a form to submit a DELETE request and add the CSRF token using the "csrf_field" directive. For editing, we define separate views and controllers in routes.
- Conclusion
In this article, we introduced how to use models and controllers in Laravel to perform basic table add, delete, modify, and query operations. While there may be more functionality and details involved in a real project, this article provides a starting point to help you get started using Laravel to handle table operations for your web applications.
The above is the detailed content of laravel table add, delete, modify. For more information, please follow other related articles on the PHP Chinese website!
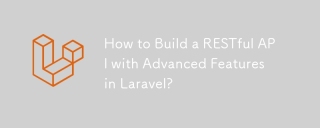
This article guides building robust Laravel RESTful APIs. It covers project setup, resource management, database interactions, serialization, authentication, authorization, testing, and crucial security best practices. Addressing scalability chall
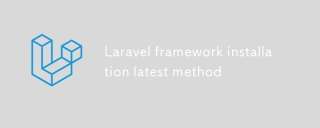
This article provides a comprehensive guide to installing the latest Laravel framework using Composer. It details prerequisites, step-by-step instructions, troubleshooting common installation issues (PHP version, extensions, permissions), and minimu
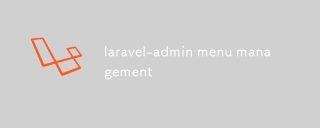
This article guides Laravel-Admin users on menu management. It covers menu customization, best practices for large menus (categorization, modularization, search), and dynamic menu generation based on user roles and permissions using Laravel's author
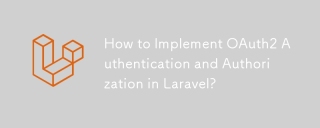
This article details implementing OAuth 2.0 authentication and authorization in Laravel. It covers using packages like league/oauth2-server or provider-specific solutions, emphasizing database setup, client registration, authorization server configu
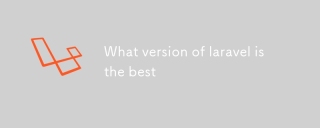
This article guides Laravel developers in choosing the right version. It emphasizes the importance of selecting the latest Long Term Support (LTS) release for stability and security, while acknowledging that newer versions offer advanced features.
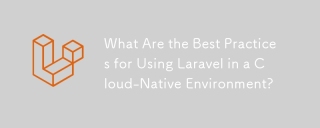
The article discusses best practices for deploying Laravel in cloud-native environments, focusing on scalability, reliability, and security. Key issues include containerization, microservices, stateless design, and optimization strategies.
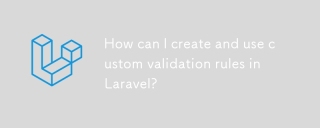
The article discusses creating and using custom validation rules in Laravel, offering steps to define and implement them. It highlights benefits like reusability and specificity, and provides methods to extend Laravel's validation system.
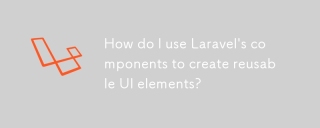
The article discusses creating and customizing reusable UI elements in Laravel using components, offering best practices for organization and suggesting enhancing packages.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
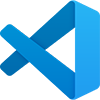
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
