1. Basic concepts
A trigger is a special type of stored procedure. A trigger is triggered by an event. Execution of
trigger trigger is similar to js events
1. Function
Forcibly check or convert data before writing to the data table (to ensure data security )
When a trigger error occurs, the result of the change will be revoked (transaction safety)
Some database management systems can define language for data DDL uses triggers, called DDL triggers
You can replace the change instructions instead of according to specific situations (mysql does not support)
2. Advantages and Disadvantages of Triggers
2.1. Advantages
Triggers can achieve cascading changes through related tables in the database (if the data of a table changes , you can use triggers to implement operations on other tables, the user does not know)
Ensure data security and perform security verification
- Excessive reliance on triggers will inevitably affect the structure of the database and increase the complexity of maintenance
- Making the data unavailable at the program level Control
create trigger 触发器名字 触发时机 触发事件 on 表 for each row
begin
end
2. Trigger object
on table for each row The trigger binds all rows in the table. When no specified change occurs in a row, the trigger will be triggered
- before : The state before the data changes
- after: The state after the data has changed
- inert insert operations
- update Update operation
- delete operation
before insert after insert before update after update before delete after delete
Requirements:
Place an order to reduce inventoryThere are two tables, one is the product table and the other is the order table (retaining the product ID) for each order generated, the corresponding inventory in the product table should changeCreate two tables:
create table my_item( id int primary key auto_increment, name varchar(20) not null, count int not null default 0 ) comment '商品表'; create table my_order( id int primary key auto_increment, item_id int not null, count int not null default 1 ) comment '订单表'; insert my_item (name, count) values ('手机', 100),('电脑', 100), ('包包', 100); mysql> select * from my_item; +----+--------+-------+ | id | name | count | +----+--------+-------+ | 1 | 手机 | 100 | | 2 | 电脑 | 100 | | 3 | 包包 | 100 | +----+--------+-------+ 3 rows in set (0.00 sec) mysql> select * from my_order; Empty set (0.02 sec)
Create trigger:
If data insertion occurs in the order table, the corresponding product should be reduced in inventorydelimiter $$ create trigger after_insert_order_trigger after insert on my_order for each row begin -- 更新商品库存 update my_item set count = count - 1 where id = 1; end $$ delimiter ;3. View the trigger
-- 查看所有触发器
show triggers\G
*************************** 1. row ***************************
Trigger: after_insert_order_trigger
Event: INSERT
Table: my_order
Statement: begin
update my_item set count = count - 1 where id = 1;
end
Timing: AFTER
Created: 2022-04-16 10:00:19.09
sql_mode: ONLY_FULL_GROUP_BY,STRICT_TRANS_TABLES,NO_ZERO_IN_DATE,NO_ZERO_DATE,ERROR_FOR_DIVISION_BY_ZERO,NO_ENGINE_SUBSTITUTION
Definer: root@localhost
character_set_client: utf8mb4
collation_connection: utf8mb4_general_ci
Database Collation: utf8mb4_general_ci
1 row in set (0.00 sec)
-- 查看创建语句
show crate trigger 触发器名字;
-- eg:
show create trigger after_insert_order_trigger;
4. Trigger the triggerLet the trigger execute , let the corresponding operation occur at the corresponding time in the table specified by the triggerinsert into my_order (item_id, count) values(1, 1); mysql> select * from my_order; +----+---------+-------+ | id | item_id | count | +----+---------+-------+ | 1 | 1 | 1 | +----+---------+-------+ 1 row in set (0.00 sec) mysql> select * from my_item; +----+--------+-------+ | id | name | count | +----+--------+-------+ | 1 | 手机 | 99 | | 2 | 电脑 | 100 | | 3 | 包包 | 100 | +----+--------+-------+ 3 rows in set (0.00 sec)5. Deleting the trigger
drop trigger 触发器名字;
-- eg
drop trigger after_insert_order_trigger;
6. Application of the triggerRecord keywords new old6. ImproveAutomatic inventory deduction for productsThe trigger targets each record in the data table, and each row of data has a corresponding one before and after the operation. The status
The trigger obtains the corresponding data status before execution:
- Save the data status before there is any operation to The status after the
old
keyword in the
- operation is placed in
new
Basic syntax:
Keyword.Field nameNot all triggers of old and new have
- insert is empty before inserting, and there is no old
- delete clear Data, no new
Item automatically deducts inventory:
delimiter $$ create trigger after_insert_order_trigger after insert on my_order for each row begin -- 通过new关键字获取新数据的id 和数量 update my_item set count = count - new.count where id = new.item_id; end $$ delimiter ;
Trigger trigger:
mysql> select * from my_order; +----+---------+-------+ | id | item_id | count | +----+---------+-------+ | 1 | 1 | 1 | +----+---------+-------+ mysql> select * from my_item; +----+--------+-------+ | id | name | count | +----+--------+-------+ | 1 | 手机 | 99 | | 2 | 电脑 | 100 | | 3 | 包包 | 100 | +----+--------+-------+ insert into my_order (item_id, count) values(2, 3); mysql> select * from my_order; +----+---------+-------+ | id | item_id | count | +----+---------+-------+ | 1 | 1 | 1 | | 2 | 2 | 3 | +----+---------+-------+ mysql> select * from my_item; +----+--------+-------+ | id | name | count | +----+--------+-------+ | 1 | 手机 | 99 | | 2 | 电脑 | 97 | | 3 | 包包 | 100 | +----+--------+-------+2.Optimization
What should I do if the inventory quantity is not as large as the product order?
-- 删除原有触发器 drop trigger after_insert_order_trigger; -- 新增判断库存触发器 delimiter $$ create trigger after_insert_order_trigger after insert on my_order for each row begin -- 查询库存 select count from my_item where id = new.item_id into @count; -- 判断 if new.count > @count then -- 中断操作,暴力抛出异常 insert into xxx values ('xxx'); end if; -- 通过new关键字获取新数据的id 和数量 update my_item set count = count - new.count where id = new.item_id; end $$ delimiter ;
Result verification:
mysql> insert into my_order (item_id, count) values(3, 101); ERROR 1146 (42S02): Table 'mydatabase2.xxx' doesn't exist mysql> select * from my_order; +----+---------+-------+ | id | item_id | count | +----+---------+-------+ | 1 | 1 | 1 | | 2 | 2 | 3 | +----+---------+-------+ 2 rows in set (0.00 sec) mysql> select * from my_item; +----+--------+-------+ | id | name | count | +----+--------+-------+ | 1 | 手机 | 99 | | 2 | 电脑 | 97 | | 3 | 包包 | 100 | +----+--------+-------+ 3 rows in set (0.00 sec)
The above is the detailed content of How to use trigger in MySQL database. For more information, please follow other related articles on the PHP Chinese website!
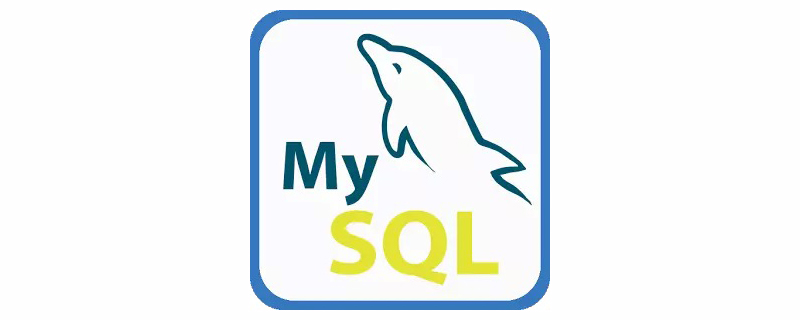
本篇文章给大家带来了关于mysql的相关知识,其中主要介绍了关于架构原理的相关内容,MySQL Server架构自顶向下大致可以分网络连接层、服务层、存储引擎层和系统文件层,下面一起来看一下,希望对大家有帮助。
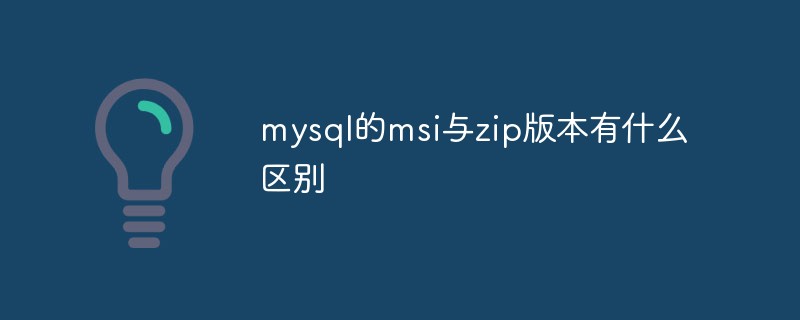
mysql的msi与zip版本的区别:1、zip包含的安装程序是一种主动安装,而msi包含的是被installer所用的安装文件以提交请求的方式安装;2、zip是一种数据压缩和文档存储的文件格式,msi是微软格式的安装包。
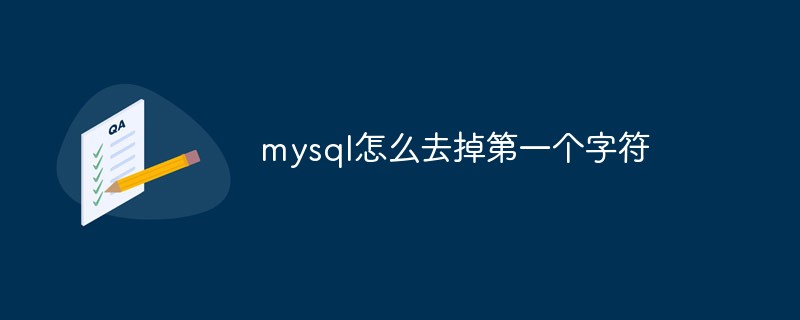
方法:1、利用right函数,语法为“update 表名 set 指定字段 = right(指定字段, length(指定字段)-1)...”;2、利用substring函数,语法为“select substring(指定字段,2)..”。
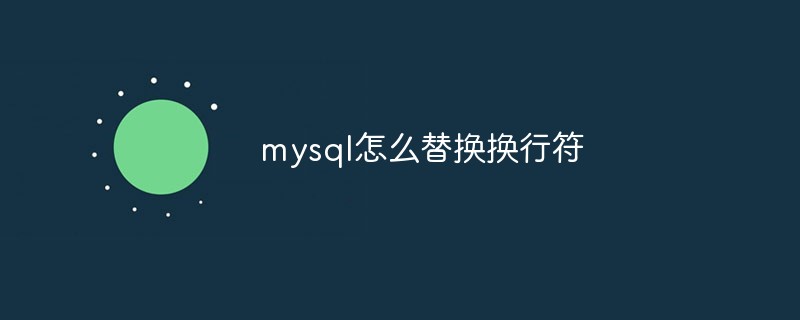
在mysql中,可以利用char()和REPLACE()函数来替换换行符;REPLACE()函数可以用新字符串替换列中的换行符,而换行符可使用“char(13)”来表示,语法为“replace(字段名,char(13),'新字符串') ”。
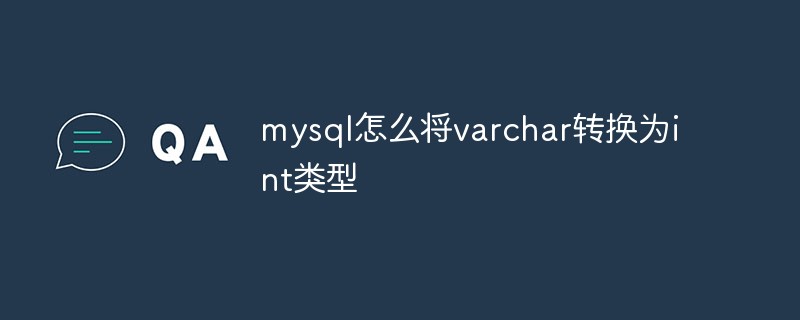
转换方法:1、利用cast函数,语法“select * from 表名 order by cast(字段名 as SIGNED)”;2、利用“select * from 表名 order by CONVERT(字段名,SIGNED)”语句。
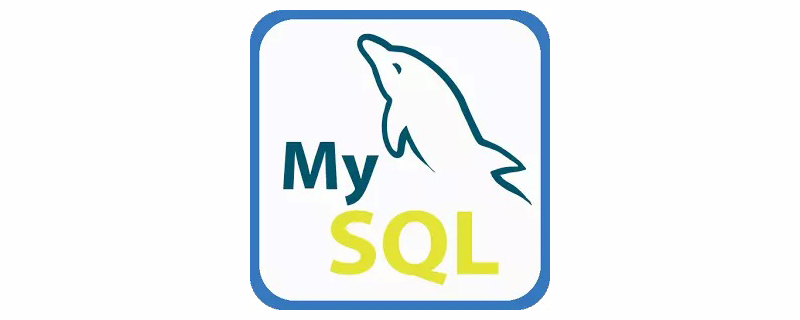
本篇文章给大家带来了关于mysql的相关知识,其中主要介绍了关于MySQL复制技术的相关问题,包括了异步复制、半同步复制等等内容,下面一起来看一下,希望对大家有帮助。
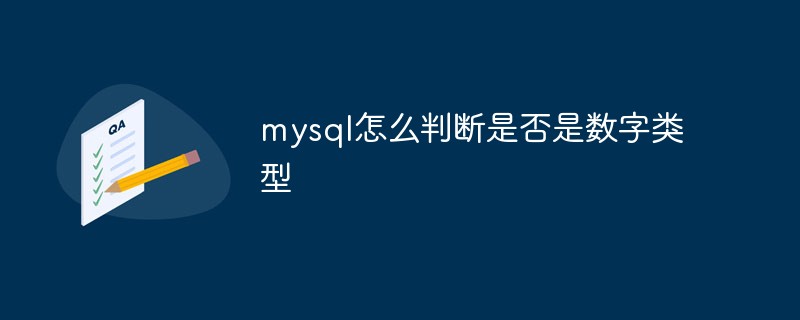
在mysql中,可以利用REGEXP运算符判断数据是否是数字类型,语法为“String REGEXP '[^0-9.]'”;该运算符是正则表达式的缩写,若数据字符中含有数字时,返回的结果是true,反之返回的结果是false。
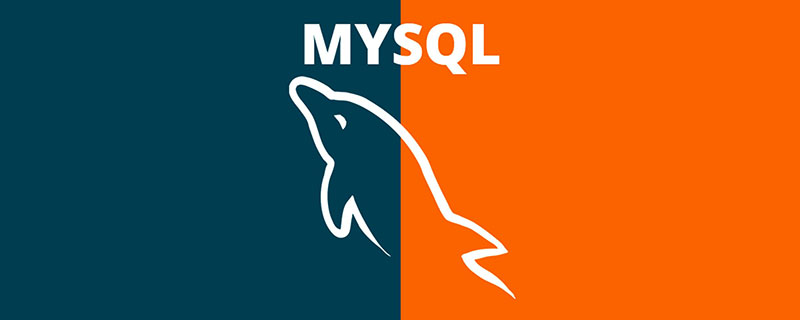
本篇文章给大家带来了关于mysql的相关知识,其中主要介绍了mysql高级篇的一些问题,包括了索引是什么、索引底层实现等等问题,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
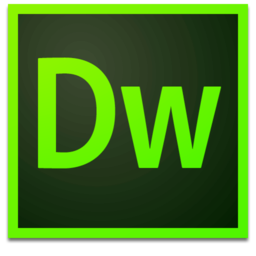
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),