In Node.js, Buffer is a class used to process binary data. When dealing with binary data, we often need to convert the data to hexadecimal format. This article will introduce how to use the built-in method of Node.js to convert a Buffer into a hexadecimal string.
- Using the toString method
The Buffer class provides the toString method, which can convert the Buffer object into a string in the specified encoding format. If the specified encoding format is hex, the toString method will return a string in hexadecimal format.
The following is a sample code:
const buffer = Buffer.from('hello', 'utf8'); const hexString = buffer.toString('hex'); console.log(hexString); // 68656c6c6f
First, we create a Buffer object containing the string "hello". Then, we call the toString method and pass in "hex" as the parameter. Finally, we print the converted hex string.
- Use the readUInt8 method
readUInt8 is an instance method in the Buffer class that can read the 8-bit unsigned integer at the specified index and convert it to ten A string in hexadecimal format.
The following is a sample code:
const buffer = Buffer.from('hello', 'utf8'); let hexString = ''; for (let i = 0; i < buffer.length; i++) { const hex = buffer.readUInt8(i).toString(16); hexString += (hex.length === 1 ? '0' + hex : hex); } console.log(hexString); // 68656c6c6f
First, we create a Buffer object containing the string "hello". We then use a for loop to iterate through each byte in the Buffer object. In the loop body, we use the readUInt8 method to read the 8-bit unsigned integer at the current index and convert it to a string in hexadecimal format. Finally, we concatenate each byte of the hex string into a complete string and print it out.
- Use the toString method and the Buffer.slice method
In addition to using the toString method, we can also use the Buffer.slice method to obtain a new Buffer object and convert it A string in hexadecimal format.
The following is a sample code:
const buffer = Buffer.from('hello', 'utf8'); const hexString = buffer.slice(0, buffer.length).toString('hex'); console.log(hexString); // 68656c6c6f
First, we create a Buffer object containing the string "hello". We then use the slice method to get a new Buffer object that contains all the bytes in the original Buffer object. Finally, we convert the new Buffer object into a string in hexadecimal format and print it out.
Summary
This article introduces three methods of converting Node.js Buffer objects to hexadecimal format strings. Using the toString method is the simplest method and the most commonly used method. Using the readUInt8 method and the Buffer.slice method requires more code, but can handle binary data more flexibly. In actual development, we can choose appropriate methods to process binary data according to different needs.
The above is the detailed content of Convert nodejs buffer to hexadecimal. For more information, please follow other related articles on the PHP Chinese website!
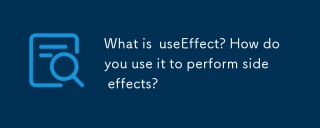
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
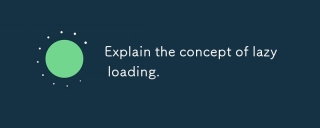
Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
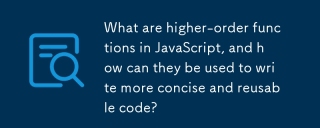
Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
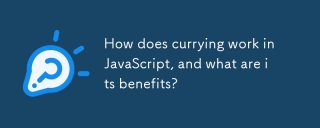
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
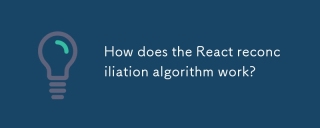
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
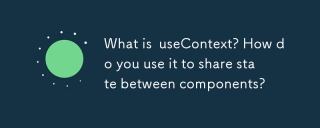
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
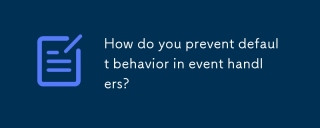
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
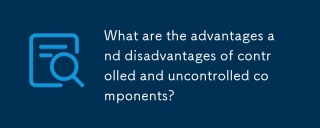
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
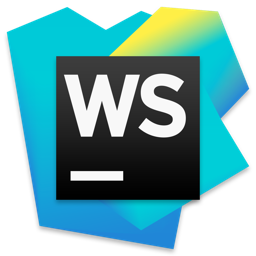
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
