golang Linked List Flip
In computer science, linked list (Linked List) is a basic data structure. A linked list is composed of a series of nodes, each node contains a data item and a reference to the next node. Linked lists are often used to implement data structures such as stacks, queues, and hash tables in programs.
In a linked list, each node has a reference to the next node. This makes linked lists ideal for insertion and deletion operations. But one disadvantage of the linked list is that when accessing any element of the linked list, you need to traverse the entire linked list from the beginning, which makes accessing the linked list very complicated. To avoid this problem, we need to reorganize the linked list so that each node points to its previous node. In this way, we can access the linked list from the end without traversing the entire linked list.
Linked list flipping is a common linked list operation. This article will introduce the method of using golang language to implement linked list flipping.
- Define the linked list node structure
First, we need to define a linked list node structure. Each node contains two properties: Value and Next.
type ListNode struct { Value int Next *ListNode }
Among them, Value is used to store the value of the current node, and Next is used to point to the address of the next node.
- Implementing the linked list flip function
Next, we need to implement the linked list flip function. The linked list flip function needs to receive the head node of a linked list as a parameter and return a flipped head node of the linked list. The code is as follows:
func reverseList(head *ListNode) *ListNode { // 定义空节点和当前节点 var prev *ListNode curr := head // 遍历整个链表 for curr != nil { // 保存当前节点的下一个节点 next := curr.Next // 将当前节点的Next指向前一个节点 curr.Next = prev // 更新prev和curr prev = curr curr = next } // 返回翻转后的链表头节点 return prev }
In this function, we use three pointers: prev, curr and next. prev points to the node that has been flipped, curr points to the node that currently needs to be flipped, and next points to the next node of curr.
We traverse the entire linked list, pointing curr's Next to prev each time, and update prev and curr. Finally, return the flipped head node of the linked list (ie, prev node).
- Complete code
The following is the complete golang code:
type ListNode struct { Value int Next *ListNode } func reverseList(head *ListNode) *ListNode { // 定义空节点和当前节点 var prev *ListNode curr := head // 遍历整个链表 for curr != nil { // 保存当前节点的下一个节点 next := curr.Next // 将当前节点的Next指向前一个节点 curr.Next = prev // 更新prev和curr prev = curr curr = next } // 返回翻转后的链表头节点 return prev }
Through the above code, we have successfully implemented the linked list flip function. In practical applications, linked list flipping is usually used to solve some problems, such as reversing strings, reversing arrays, etc. Mastering linked list operation skills is very important for writing efficient and stable programs.
The above is the detailed content of golang linked list flipping. For more information, please follow other related articles on the PHP Chinese website!
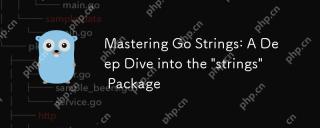
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
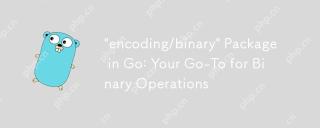
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
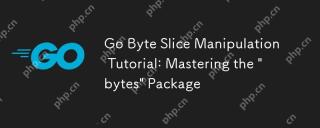
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.
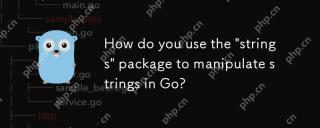
You can use the "strings" package in Go to manipulate strings. 1) Use strings.TrimSpace to remove whitespace characters at both ends of the string. 2) Use strings.Split to split the string into slices according to the specified delimiter. 3) Merge string slices into one string through strings.Join. 4) Use strings.Contains to check whether the string contains a specific substring. 5) Use strings.ReplaceAll to perform global replacement. Pay attention to performance and potential pitfalls when using it.
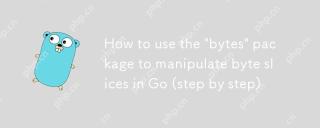
ThebytespackageinGoishighlyeffectiveforbyteslicemanipulation,offeringfunctionsforsearching,splitting,joining,andbuffering.1)Usebytes.Containstosearchforbytesequences.2)bytes.Splithelpsbreakdownbyteslicesusingdelimiters.3)bytes.Joinreconstructsbytesli
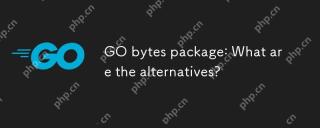
ThealternativestoGo'sbytespackageincludethestringspackage,bufiopackage,andcustomstructs.1)Thestringspackagecanbeusedforbytemanipulationbyconvertingbytestostringsandback.2)Thebufiopackageisidealforhandlinglargestreamsofbytedataefficiently.3)Customstru
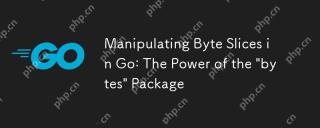
The"bytes"packageinGoisessentialforefficientlymanipulatingbyteslices,crucialforbinarydata,networkprotocols,andfileI/O.ItoffersfunctionslikeIndexforsearching,Bufferforhandlinglargedatasets,Readerforsimulatingstreamreading,andJoinforefficient
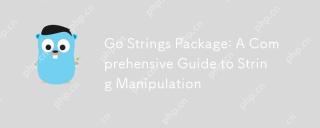
Go'sstringspackageiscrucialforefficientstringmanipulation,offeringtoolslikestrings.Split(),strings.Join(),strings.ReplaceAll(),andstrings.Contains().1)strings.Split()dividesastringintosubstrings;2)strings.Join()combinesslicesintoastring;3)strings.Rep


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
