As web applications become more and more complex, exception handling in PHP development becomes more and more important. Exceptions refer to errors that occur during program execution. When an exception occurs in the program, the execution flow of the code will be interrupted, and the program will jump to the exception handler and handle it accordingly. In PHP, the exception handling mechanism can help developers diagnose and solve various problems in the program more easily.
The exception handling mechanism in PHP is based on try-catch blocks. The try block contains code that may throw exceptions. When an exception is thrown in the try block, the catch block will catch the exception and handle it accordingly.
The following is a simple PHP exception handling code example:
try { $file = fopen("example.txt", "r"); if (!$file) { throw new Exception("无法打开文件!"); } } catch (Exception $e) { echo "捕获到异常:" . $e->getMessage(); }
In the above code, we try to open a file named example.txt. If the file cannot be opened, an exception is thrown and handled in the catch block.
In PHP, different types of exceptions can be used to represent different error types. For example, you can use PHP's built-in Exception class to represent generic exception conditions, or you can create custom exception types to better suit the needs of a specific application.
When handling exceptions, PHP allows developers to perform complex nesting and multiple catch blocks. This means that multiple different types of exceptions can be caught and handled, with appropriate handlers specified for each type. For example:
try { // some code } catch (Exception $e) { // 处理通用异常 } catch (InvalidArgumentException $e) { // 处理无效参数异常 } catch (OutOfBoundsException $e) { // 处理溢出异常 }
PHP also provides the keyword finally, which can be added after the try-catch block. Code in the finally block will always be executed regardless of whether an exception occurs. In the finally block you can release resources or perform other similar operations.
Exception handling is especially important for web applications because they allow applications to better handle user input and other unpredictable situations. By using exception handling mechanisms, developers can write more robust code and diagnose and resolve problems in their programs faster.
It should be noted that excessive exception handling will have a negative impact on performance. Therefore, when writing exception handling code, you should keep it as concise and readable as possible. Use exceptions only when necessary to ensure the efficiency and maintainability of your code.
When developing web applications, exception handling is an essential technology that can help developers better handle problems that arise in the program. In PHP, the exception handling mechanism is very flexible and powerful, which can help programmers write more robust code and improve software quality and performance.
The above is the detailed content of Exception handling in PHP development. For more information, please follow other related articles on the PHP Chinese website!
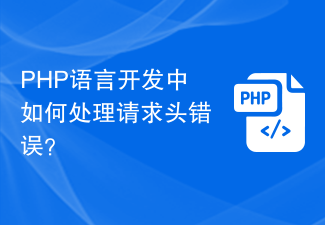
在PHP语言开发中,请求头错误通常是由于HTTP请求中的一些问题导致的。这些问题可能包括无效的请求头、缺失的请求体以及无法识别的编码格式等。而正确处理这些请求头错误是保证应用程序稳定性和安全性的关键。在本文中,我们将讨论一些处理PHP请求头错误的最佳实践,帮助您构建更加可靠和安全的应用程序。检查请求方法HTTP协议规定了一组可用的请求方法(例如GET、POS
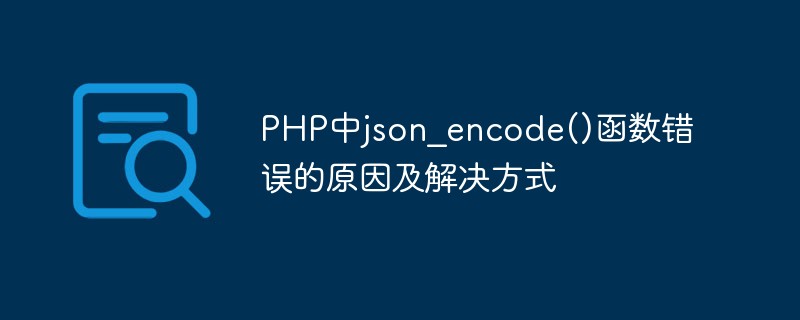
随着Web应用程序的不断发展,数据交互成为了一个非常重要的环节。其中,JSON(JavaScriptObjectNotation)是一种轻量级的数据交换格式,广泛用于前后端数据交互。在PHP中,json_encode()函数可以将PHP数组或对象转换为JSON格式字符串,json_decode()函数可以将JSON格式字符串转换为PHP数组或对象。然而,
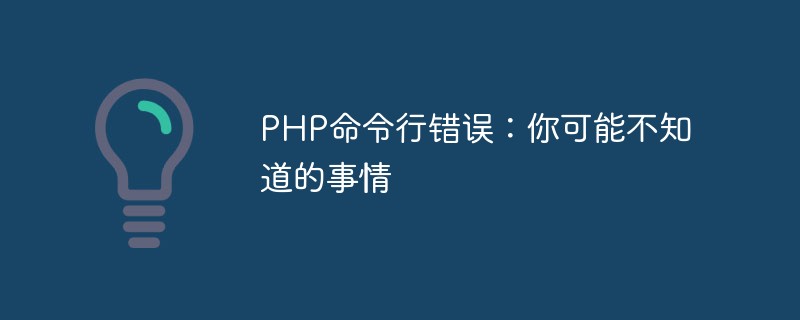
本文将介绍关于PHP命令行错误的一些你可能不知道的事情。PHP作为一门流行的服务器端语言,一般运行在Web服务器上,但它也可以在命令行上直接运行,比如在Linux或者MacOS系统下,我们可以在终端中输入“php”命令来直接运行PHP脚本。不过,就像在Web服务器中一样,当我们在命令行中运行PHP脚本时,也会遇到一些错误。以下是一些你可能不知道的有关PHP命
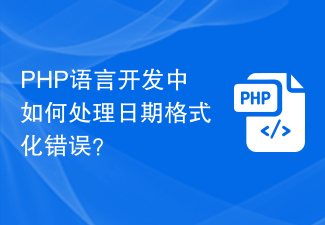
在PHP语言开发中,日期格式化错误是一个常见的问题。正确的日期格式对于程序员来说十分重要,因为它决定着代码的可读性、可维护性和正确性。本文将分享一些处理日期格式化错误的技巧。了解日期格式在处理日期格式化错误之前,我们必须先了解日期格式。日期格式是由各种字母和符号组成的字符串,用于表示特定的日期和时间格式。在PHP中,常见的日期格式包括:Y:四位数年份(如20
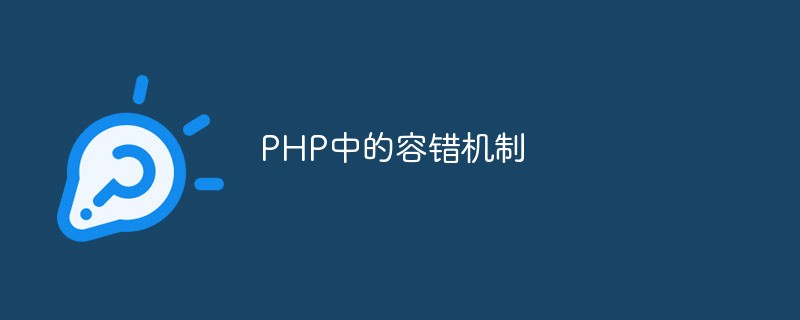
在编写程序时总会存在各种各样的错误和异常。任何编程语言都需要有良好的容错机制,PHP也不例外。PHP有许多内置的错误和异常处理机制,可以让开发者更好地管理其代码,并正确地处理各种问题。下面就让我们一起来了解一下PHP中的容错机制。错误级别PHP中有四个错误级别:致命错误、严重错误、警告和通知。每个错误级别都有一个不同的符号表示,以帮助识别和处理错误:E_ER
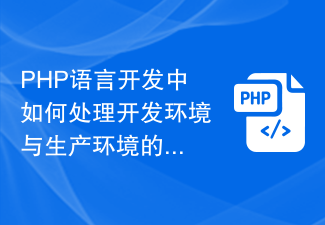
随着互联网的快速发展,开发人员的任务也随之多样化和复杂化。特别是对于PHP语言开发人员而言,在开发过程中面临的最常见问题之一就是在开发环境和生产环境中,数据不一致的错误问题。因此,在开发PHP应用程序时,如何处理这些错误是开发人员必须面对的一个重要问题。开发环境和生产环境的区别首先需要明确的是,开发环境和生产环境是不同的,它们有着不同的设置和配置。在开发环境
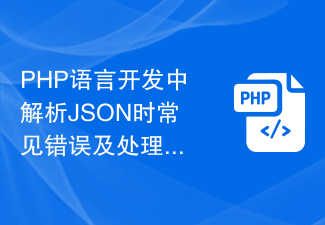
在PHP语言开发中,常常需要解析JSON数据,以便进行后续的数据处理和操作。然而,在解析JSON时,很容易遇到各种错误和问题。本文将介绍常见的错误和处理方法,帮助PHP开发者更好地处理JSON数据。一、JSON格式错误最常见的错误是JSON格式不正确。JSON数据必须符合JSON规范,即数据必须是键值对的集合,并使用大括号({})和中括号([])来包含数据。
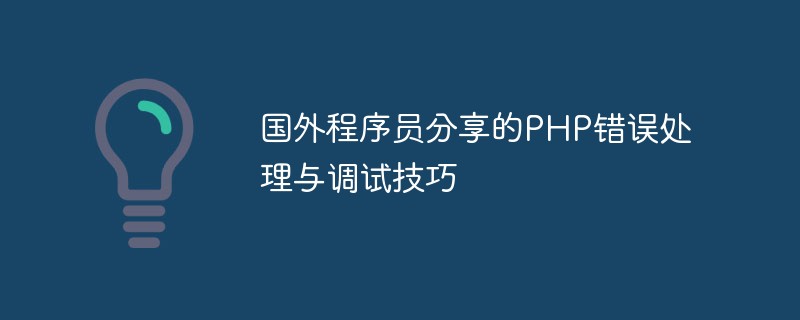
PHP(HypertextPreprocessor)是一种广泛用于Web开发的脚本语言。在开发PHP应用程序时,错误处理和调试被认为是非常重要的一块。国外程序员在经验中积累了许多PHP错误处理和调试技巧,下面介绍一些比较常见和实用的技巧。错误报告级别修改在PHP中,通过修改错误报告级别可以显示或禁止显示特定类型的PHP错误。通过设置错误报告级别为“E_AL


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
