Javascript is a widely used programming language that provides developers with powerful operators and control flow to process and manipulate data. In Javascript, there are some operators that are not widely discussed and used. This article will introduce these operators that are not regular operators in Javascript.
- Ternary operator ( ? : )
The ternary operator is also called the conditional operator, it is a concise way to write if-else statement. In Javascript, its syntax is:
condition ? expr1 : expr2
When the condition is true, the expression expr1 is executed. When the condition is false, expression expr2 is executed. For example:
var age = 18;
var isAdult = age >= 18 ? true : false;
console.log(isAdult); // true
This paragraph The code will determine whether the adult is an adult based on the value of the variable age. If the age is greater than or equal to 18 years old, the isAdult variable is assigned a value of true, otherwise it is assigned a value of false.
- Comma operator ( , )
The comma operator is a way to separate multiple expressions. The comma operator is also called a sequence operator because it can combine multiple expressions into a sequence. In Javascript, commas are used to separate expressions, for example:
var x = 1, y = 2, z = 3;
console.log(x, y, z); // 1 2 3
In this example, the comma operator is used to declare multiple variables in one statement, and Assign values to them.
- void operator
The void operator is used to execute an expression but does not return any value. In Javascript, the void operator is followed by any expression and returns undefined. For example:
var result = void 0;
console.log(result); // undefined
In this example, the void 0 operator returns undefined and assigns it to the variable result.
- delete operator
The delete operator is used to delete attributes of an object or elements of an array. In Javascript, the syntax is as follows:
delete object.property; // Delete the properties of the object
delete array[index]; // Delete the elements of the array
For example:
var obj = {name: 'Jim', age: 18};
delete obj.age;
console.log(obj); // {name: 'Jim'}
In this example, the delete operator is used to delete the age attribute of object obj.
- instanceof operator
The instanceof operator is used to check whether an object is an instance of a certain class. In Javascript, the syntax is as follows:
object instanceof class
For example:
var arr = [1, 2, 3];
console.log(arr instanceof Array); // true
In this example, the instanceof operator is used to check whether arr is an instance of the Array class.
Summary
The above are less commonly used operators in Javascript, and they may be very useful in specific scenarios. When we master the use of these operators, we can better process and manipulate data. It should be noted that these operators may not be supported by all browsers or Javascript engines and need to be used with caution.
The above is the detailed content of Operators that are not part of javascript. For more information, please follow other related articles on the PHP Chinese website!

React is the tool of choice for building dynamic and interactive user interfaces. 1) Componentization and JSX make UI splitting and reusing simple. 2) State management is implemented through the useState hook to trigger UI updates. 3) The event processing mechanism responds to user interaction and improves user experience.

React is a front-end framework for building user interfaces; a back-end framework is used to build server-side applications. React provides componentized and efficient UI updates, and the backend framework provides a complete backend service solution. When choosing a technology stack, project requirements, team skills, and scalability should be considered.

The relationship between HTML and React is the core of front-end development, and they jointly build the user interface of modern web applications. 1) HTML defines the content structure and semantics, and React builds a dynamic interface through componentization. 2) React components use JSX syntax to embed HTML to achieve intelligent rendering. 3) Component life cycle manages HTML rendering and updates dynamically according to state and attributes. 4) Use components to optimize HTML structure and improve maintainability. 5) Performance optimization includes avoiding unnecessary rendering, using key attributes, and keeping the component single responsibility.

React is the preferred tool for building interactive front-end experiences. 1) React simplifies UI development through componentization and virtual DOM. 2) Components are divided into function components and class components. Function components are simpler and class components provide more life cycle methods. 3) The working principle of React relies on virtual DOM and reconciliation algorithm to improve performance. 4) State management uses useState or this.state, and life cycle methods such as componentDidMount are used for specific logic. 5) Basic usage includes creating components and managing state, and advanced usage involves custom hooks and performance optimization. 6) Common errors include improper status updates and performance issues, debugging skills include using ReactDevTools and Excellent

React is a JavaScript library for building user interfaces, with its core components and state management. 1) Simplify UI development through componentization and state management. 2) The working principle includes reconciliation and rendering, and optimization can be implemented through React.memo and useMemo. 3) The basic usage is to create and render components, and the advanced usage includes using Hooks and ContextAPI. 4) Common errors such as improper status update, you can use ReactDevTools to debug. 5) Performance optimization includes using React.memo, virtualization lists and CodeSplitting, and keeping code readable and maintainable is best practice.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.

React components can be defined by functions or classes, encapsulating UI logic and accepting input data through props. 1) Define components: Use functions or classes to return React elements. 2) Rendering component: React calls render method or executes function component. 3) Multiplexing components: pass data through props to build a complex UI. The lifecycle approach of components allows logic to be executed at different stages, improving development efficiency and code maintainability.
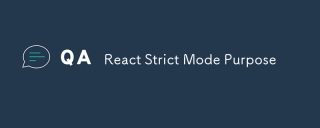
React Strict Mode is a development tool that highlights potential issues in React applications by activating additional checks and warnings. It helps identify legacy code, unsafe lifecycles, and side effects, encouraging modern React practices.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
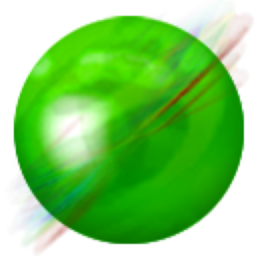
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
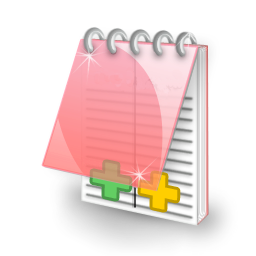
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
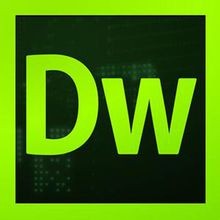
Dreamweaver CS6
Visual web development tools