Buffer generation algorithm implementation javascript
JavaScript is a general-purpose scripting language widely used in web development and server-side applications. Buffers are generally used for processing binary data, such as images and audio. In this article, we will explore how to implement the buffer generation algorithm using JavaScript.
What is a buffer?
Buffer is a data structure used to store binary data. Unlike regular JavaScript types, buffers are fixed size and can only contain specific types of data. A buffer object is an instance of the ArrayBuffer class, which is a fixed-size structure of unsigned binary data.
How to create a buffer?
The buffer can be created by the following code:
let buffer = new ArrayBuffer(size);
where size is the size of the buffer in bytes. Using buffers we can process binary data, such as uploading image data to the server.
Implementing the buffer generation algorithm
The buffer algorithm is a common algorithm for processing binary data, such as hashing algorithms and encryption algorithms. In this section, we will implement the buffer generation algorithm, an algorithm for generating random buffers.
The algorithm for generating a random buffer is as follows:
- Create a buffer of the specified size.
- Generate a random number.
- Store the random number in the first byte of the buffer.
- Process the remaining bytes of the buffer, generating a random number for each byte until the entire buffer is filled.
Let's implement this algorithm:
function generateRandomBuffer(size) { // 创建指定大小的缓冲区 const buffer = new ArrayBuffer(size); // 获取缓冲区的总长度 const bufferLength = buffer.byteLength; // 生成第一个字节的随机数 const firstByte = Math.floor(Math.random() * 256); // 将随机数存储在第一个字节中 const uint8Array = new Uint8Array(buffer); uint8Array[0] = firstByte; // 处理缓冲区的其余字节 for (let i = 1; i < bufferLength; i++) { // 生成一个随机数 const randomByte = Math.floor(Math.random() * 256); // 将随机数存储在缓冲区的字节中 uint8Array[i] = randomByte; } // 返回生成的缓冲区 return buffer; }
In this example, we use the Uint8Array class to directly access the bytes in the buffer. The Uint8Array object can accept an ArrayBuffer object through the constructor and provides an array-like interface.
Now, we can use the following code to generate a random buffer:
const buffer = generateRandomBuffer(1024);
This will create a random buffer of size 1024 bytes.
Conclusion
In this article, we explored the process of implementing a buffer generation algorithm using JavaScript. We learned how to create and use buffers and implemented a simple buffer generation algorithm. The buffer is an important data structure for processing binary data. JavaScript provides us with tools to conveniently use buffers by providing the ArrayBuffer class and corresponding view types.
The above is the detailed content of Buffer generation algorithm implementation javascript. For more information, please follow other related articles on the PHP Chinese website!

Classesarebetterforaccessibilityinwebdevelopment.1)Classescanbeappliedtomultipleelements,ensuringconsistentstylesandbehaviors,whichaidsuserswithdisabilities.2)TheyfacilitatetheuseofARIAattributesacrossgroupsofelements,enhancinguserexperience.3)Classe

Classselectorsarereusableformultipleelements,whileIDselectorsareuniqueandusedonceperpage.1)Classes,denotedbyaperiod(.),areidealforstylingmultipleelementslikebuttons.2)IDs,denotedbyahash(#),areperfectforuniqueelementslikeanavigationmenu.3)IDshavehighe

In CSS style, the class selector or ID selector should be selected according to the project requirements: 1) The class selector is suitable for reuse and is suitable for the same style of multiple elements; 2) The ID selector is suitable for unique elements and has higher priority, but should be used with caution to avoid maintenance difficulties.

HTML5hasseverallimitationsincludinglackofsupportforadvancedgraphics,basicformvalidation,cross-browsercompatibilityissues,performanceimpacts,andsecurityconcerns.1)Forcomplexgraphics,HTML5'scanvasisinsufficient,requiringlibrarieslikeWebGLorThree.js.2)I

Yes,onestylecanhavemoreprioritythananotherinCSSduetospecificityandthecascade.1)Specificityactsasascoringsystemwheremorespecificselectorshavehigherpriority.2)Thecascadedeterminesstyleapplicationorder,withlaterrulesoverridingearlieronesofequalspecifici

ThesignificantgoalsofHTML5aretoenhancemultimediasupport,ensurehumanreadability,maintainconsistencyacrossdevices,andensurebackwardcompatibility.1)HTML5improvesmultimediawithnativeelementslikeand.2)ItusessemanticelementsforbetterreadabilityandSEO.3)Its

React'slimitationsinclude:1)asteeplearningcurveduetoitsvastecosystem,2)SEOchallengeswithclient-siderendering,3)potentialperformanceissuesinlargeapplications,4)complexstatemanagementasappsgrow,and5)theneedtokeepupwithitsrapidevolution.Thesefactorsshou

Reactischallengingforbeginnersduetoitssteeplearningcurveandparadigmshifttocomponent-basedarchitecture.1)Startwithofficialdocumentationforasolidfoundation.2)UnderstandJSXandhowtoembedJavaScriptwithinit.3)Learntousefunctionalcomponentswithhooksforstate


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
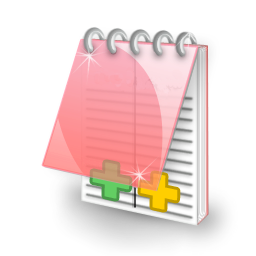
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
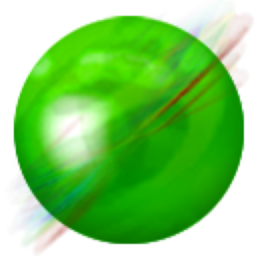
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
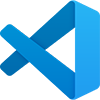
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
