How to do object-oriented programming in PHP7.0?
PHP 7.0 is a relatively important version of PHP, with many improvements in performance. One of the important features is support for richer and more advanced object-oriented programming techniques. This article will introduce in detail how to do object-oriented programming in PHP 7.0.
Why use object-oriented programming?
In object-oriented programming, data and corresponding behaviors are encapsulated together, which makes the code easier to maintain and expand, and also improves the reusability of the code. In addition, object-oriented programming also has the following characteristics:
- Abstraction - the ability to better abstract and model business logic and data.
- Inheritance - you can use existing code and extend its functionality.
- Interface - A specification that defines some or all of the behavior of a class.
- Polymorphism - Due to the existence of inheritance and interfaces, the same method can have different behaviors on different objects.
How to do object-oriented programming in PHP 7.0?
In PHP 7.0, we can use the Class keyword to define classes. The following is a simple example:
class Person{ private $name; public function setName($name){ $this->name = $name; } public function getName(){ return $this->name; } }
In this example, we define a class named Person. This class has a private variable $name and two public methods - setName and getName. Private variables can only be accessed within the class. Public methods can be called and accessed from outside the class.
The following is an example of using the Person class:
$p = new Person(); $p->setName('张三'); echo $p->getName(); // 输出“张三”
In the above example, we create a Person object named $p and use the setName and getName methods to set and get it name.
PHP 7.0 also supports namespaces (Namespaces), which is a very useful feature. Namespaces can group classes and functions into a separate namespace to avoid name conflicts. The following is an example of using namespaces:
namespace MyProject; class Person{ // ... } function doStuff(){ // ... }
In the above code, we put the Person class and doStuff function into a namespace called MyProject. When using this class and function, we can call it like this:
$p = new MyProjectPerson(); MyProjectdoStuff();
Trait feature was also introduced in PHP 7.0. Traits allow some methods and properties to be shared among multiple classes. Use traits to better organize your code and avoid copying and pasting. The following is an example of using Trait:
trait Logger{ protected function log($msg){ // ... } } class Person{ use Logger; public function doSomething(){ $this->log('doing something'); } }
In the above example, we defined a Trait named Logger, which contains a log method. The Person class uses this Trait and calls the log method in its doSomething method.
PHP 7.0 also introduced the concept of anonymous classes (Anonymous Classes). An anonymous class is a class without a name. Unlike named classes, anonymous classes do not need to define a class name and can be generated dynamically at runtime. Anonymous classes are often used to define and use simple callback functions and event handlers.
The following is an example of a simple anonymous class:
$myClass = new class { public function sayHello(){ echo 'Hello'; } }; $myClass->sayHello(); // 输出“Hello”
In the above example, we use the new class keyword to dynamically create an anonymous class and add a sayHello method to it.
Conclusion
PHP 7.0 has rich object-oriented programming features, including classes, namespaces, traits, anonymous classes, etc. Object-oriented programming allows you to better abstract and model business logic and data, and improve the maintainability, scalability, and reusability of your code. If you want to use PHP 7.0 to write large, complex applications, object-oriented programming will be an indispensable technology.
The above is the detailed content of How to do object-oriented programming in PHP7.0?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
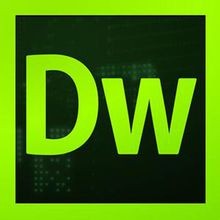
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Atom editor mac version download
The most popular open source editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
