Node.js is an event-driven asynchronous server-side JavaScript runtime environment. It is lightweight, power efficient and scalable. However, due to the asynchronous execution model of Node.js, there are some cases where wait/delay operations similar to synchronous operations need to be manually implemented. Sleep operations are often used to simulate long-running operations or in certain tasks that need to be executed after a period of time. This article will introduce how to implement sleep operations in Node.js.
Method 1: Use the setTimeout function
The setTimeout method is a built-in function of Node.js, which can be used to trigger a callback function after a specified number of milliseconds. We can use this feature to simulate sleep operations.
The following is an example:
function sleep(ms) { return new Promise(resolve => setTimeout(resolve, ms)); } async function main() { console.log('start'); await sleep(2000); // 睡眠2秒 console.log('end'); } main();
In the above code, we define an asynchronous function sleep, which will return a Promise object and call the resolve method after the specified number of milliseconds. The function main calls the sleep function and waits for 2 seconds before outputting a line of log. Through async/await syntax, we can implement sleep operations very conveniently.
Method 2: Use a custom Promise object
We can also implement a Promise object ourselves to implement sleep operations. This method is more flexible and you can customize the execution method of Promise objects according to your own needs.
The following is an example:
function sleep(ms) { return new Promise(resolve => { let startTime = new Date().getTime(); while (new Date().getTime() < startTime + ms); resolve(); }); } async function main() { console.log('start'); await sleep(2000); // 睡眠2秒 console.log('end'); } main();
In this example, we define a function sleep that will execute a loop (for simulation timing) within the specified number of milliseconds. When the loop ends, we call the resolve method to end the execution of the Promise. The function main calls the sleep function and waits for 2 seconds before outputting a line of log.
It should be noted that this method will also occupy CPU resources while implementing sleep operations. It is not recommended to be used in a production environment and is only used under special circumstances when long-running operations need to be simulated.
Conclusion
No matter which method is used, implementing sleep operations is simple. Although the asynchronous execution model of Node.js is good at handling high-concurrency requests, we also have a variety of methods to implement some operations that require waiting/delay. It is necessary to choose the appropriate implementation method according to actual needs.
The above is the detailed content of nodejs sleep method. For more information, please follow other related articles on the PHP Chinese website!

React is a JavaScript library for building modern front-end applications. 1. It uses componentized and virtual DOM to optimize performance. 2. Components use JSX to define, state and attributes to manage data. 3. Hooks simplify life cycle management. 4. Use ContextAPI to manage global status. 5. Common errors require debugging status updates and life cycles. 6. Optimization techniques include Memoization, code splitting and virtual scrolling.

React's future will focus on the ultimate in component development, performance optimization and deep integration with other technology stacks. 1) React will further simplify the creation and management of components and promote the ultimate in component development. 2) Performance optimization will become the focus, especially in large applications. 3) React will be deeply integrated with technologies such as GraphQL and TypeScript to improve the development experience.

React is a JavaScript library for building user interfaces. Its core idea is to build UI through componentization. 1. Components are the basic unit of React, encapsulating UI logic and styles. 2. Virtual DOM and state management are the key to component work, and state is updated through setState. 3. The life cycle includes three stages: mount, update and uninstall. The performance can be optimized using reasonably. 4. Use useState and ContextAPI to manage state, improve component reusability and global state management. 5. Common errors include improper status updates and performance issues, which can be debugged through ReactDevTools. 6. Performance optimization suggestions include using memo, avoiding unnecessary re-rendering, and using us

Using HTML to render components and data in React can be achieved through the following steps: Using JSX syntax: React uses JSX syntax to embed HTML structures into JavaScript code, and operates the DOM after compilation. Components are combined with HTML: React components pass data through props and dynamically generate HTML content, such as. Data flow management: React's data flow is one-way, passed from the parent component to the child component, ensuring that the data flow is controllable, such as App components passing name to Greeting. Basic usage example: Use map function to render a list, you need to add a key attribute, such as rendering a fruit list. Advanced usage example: Use the useState hook to manage state and implement dynamics

React is the preferred tool for building single-page applications (SPAs) because it provides efficient and flexible ways to build user interfaces. 1) Component development: Split complex UI into independent and reusable parts to improve maintainability and reusability. 2) Virtual DOM: Optimize rendering performance by comparing the differences between virtual DOM and actual DOM. 3) State management: manage data flow through state and attributes to ensure data consistency and predictability.

React is a JavaScript library developed by Meta for building user interfaces, with its core being component development and virtual DOM technology. 1. Component and state management: React manages state through components (functions or classes) and Hooks (such as useState), improving code reusability and maintenance. 2. Virtual DOM and performance optimization: Through virtual DOM, React efficiently updates the real DOM to improve performance. 3. Life cycle and Hooks: Hooks (such as useEffect) allow function components to manage life cycles and perform side-effect operations. 4. Usage example: From basic HelloWorld components to advanced global state management (useContext and

The React ecosystem includes state management libraries (such as Redux), routing libraries (such as ReactRouter), UI component libraries (such as Material-UI), testing tools (such as Jest), and building tools (such as Webpack). These tools work together to help developers develop and maintain applications efficiently, improve code quality and development efficiency.

React is a JavaScript library developed by Facebook for building user interfaces. 1. It adopts componentized and virtual DOM technology to improve the efficiency and performance of UI development. 2. The core concepts of React include componentization, state management (such as useState and useEffect) and the working principle of virtual DOM. 3. In practical applications, React supports from basic component rendering to advanced asynchronous data processing. 4. Common errors such as forgetting to add key attributes or incorrect status updates can be debugged through ReactDevTools and logs. 5. Performance optimization and best practices include using React.memo, code segmentation and keeping code readable and maintaining dependability


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
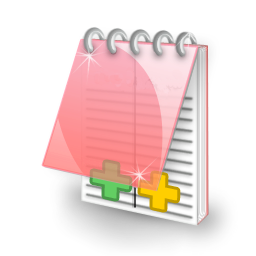
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool