PHP is a popular server-side programming language that is widely used to develop web applications. In PHP development, operating databases is one of the very common requirements. When we get data from the database, data duplication often occurs. If we need to deduplicate these duplicate data, we can use PHP's array deduplication method to achieve it. This article will introduce how to use PHP to remove duplicate data from arrays and apply these operations to the database.
1. PHP array deduplication
1. Use the array_unique() function
PHP provides the array_unique() function to implement array deduplication. This function returns a deduplicated array without changing the original input array. Usage examples are as follows:
$array = array('a', 'b', 'c', 'd', 'a', 'b'); $result = array_unique($array); print_r($result);
Output:
Array ( [0] => a [1] => b [2] => c [3] => d )
2. Use the array_flip() and array_keys() functions
Another way is to use the array_flip() function to convert the array Exchange keys and values, and then use the array_keys() function to retrieve the key array. Because the key names are unique, this method can also achieve array deduplication. Usage examples are as follows:
$array = array('a', 'b', 'c', 'd', 'a', 'b'); $result = array_keys(array_flip($array)); print_r($result);
Output:
Array ( [0] => a [1] => b [2] => c [3] => d )
The implementation principles of the two methods are slightly different, but they can both achieve array deduplication. For arrays with small data sizes, both methods have no performance issues. But if you need to deduplicate an array with a large amount of data, the second method will perform better.
2. PHP array deduplication is applied to the database
Now assume that there is a students table with duplicate records, and the PHP deduplication function is used to deduplicate it and store it in a new students table. The code example is as follows:
<?php //连接数据库 $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "myDB"; $conn = new mysqli($servername, $username, $password, $dbname); //检测连接 if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } //从students表中获取数据 $sql = "SELECT * FROM students"; $result = $conn->query($sql); //将数据存入一个数组 $array = array(); if ($result->num_rows > 0) { while($row = $result->fetch_assoc()) { $array[] = $row; } } //使用array_unique()函数进行数组去重 $unique = array_unique($array, SORT_REGULAR); //清空旧表 $sql = "TRUNCATE TABLE students"; $conn->query($sql); //将去重后的数据插入新表 foreach($unique as $row) { $sql = "INSERT INTO students (name,age,grade) VALUES ('" . $row['name'] . "'," . $row['age'] . "," . $row['grade'] . ")"; $conn->query($sql); } //关闭数据库连接 $conn->close(); ?>
In the code, we first connect to the database and obtain data from the students table, then store the data into an array, use the array_unique() function to deduplicate the array, and finally remove The repeated data is inserted into the new table.
3. Conclusion
This article introduces the method of using PHP array to remove duplicates, including using the array_unique() function and array_flip() function. These methods are effective means to achieve array deduplication. In addition, we also applied these methods to the database to complete the operation of removing duplicate records from the duplicate record table and storing them in a new table. This is a very common practical requirement in PHP development and can be used flexibly in actual development.
The above is the detailed content of php remove duplicate databases from array. For more information, please follow other related articles on the PHP Chinese website!
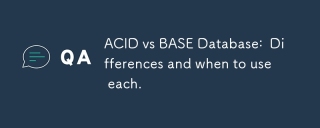
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
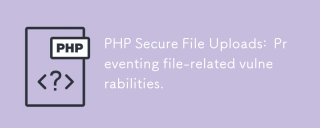
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
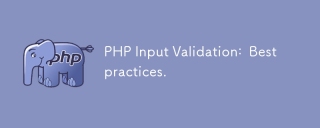
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
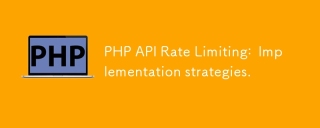
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
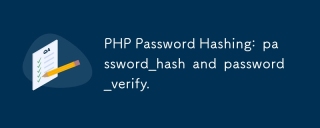
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
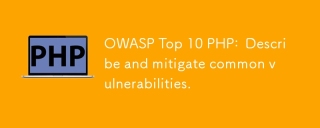
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
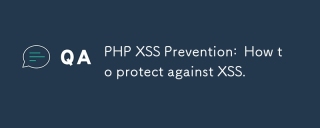
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
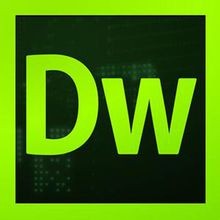
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool