The purpose and use of arrays in PHP
In PHP, arrays are a very important data type. Because PHP is a dynamic language, it does not require the type and size of variables to be declared before use, which makes arrays particularly important for storing and processing variable amounts of data. In this article, we will explore the purpose and usage of arrays in PHP.
- The concept of array
First of all, we need to understand what an array is. An array is a data type consisting of elements of the same type that can be accessed through a unique array name and one or more subscripts. In PHP, subscripts can be integers or strings.
- Uses of arrays
Arrays are very widely used in PHP. They can be used in the following aspects:
(1) Store data
Array is a very convenient data structure that can be used to store multiple values. For example, we could use an array to store a list of students' names and ages, or store temperature data for different cities.
(2) Accessing data
Because arrays can be accessed through a unique array name and one or more subscripts, you can use it to easily access data elements. For example, we can use arrays to access a paginated list of articles, or to access a list of products in an online store.
(3) Processing data
Using arrays can simplify data processing. For example, we can use array functions to sort, search, merge, etc. data stored in an array.
- Declaration and initialization of arrays
In PHP, you can declare and initialize an array in the following ways:
(1) Use array() Function
array() function is a common way to create an array. For example, the following code creates an array to store names:
$names = array("Tom", "Jerry", "Mike");
(2) Use square brackets
Brackets can be used to specify the element subscript of the array. For example, the following code creates an array that stores ages:
$ages[0] = 22;
$ages[1] = 25;
$ages[2] = 20;
- Access and operation of arrays
In PHP, you can access and operate arrays in the following ways:
(1) Use square brackets
You can use square brackets to specify the subscript of the array to access the array elements. For example, the following code outputs the second element of the $names array:
echo $names[1];
(2) Using foreach loop
foreach loop can be traversed all elements of the array. For example, the following code outputs all elements of the $names array:
foreach ($names as $name) {
echo $name;
}
(3) Using arrays Function
PHP provides many array functions to operate and process arrays. For example, the following code uses the sort() function to sort the $ages array:
sort($ages);
The above is the purpose and use of arrays in PHP. By mastering arrays, we can process and operate data more efficiently.
The above is the detailed content of The purpose and use of arrays in PHP. For more information, please follow other related articles on the PHP Chinese website!
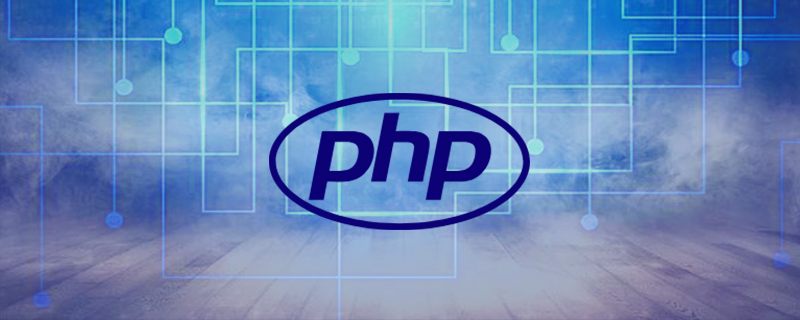
求和方法:1、用array_column()获取多维数组中指定一列的全部元素,语法“rray_column(数组, '指定列名')”,会返回一个包含全部元素的结果数组;2、用“array_sum(结果数组)”计算结果数组中所有元素的和即可。
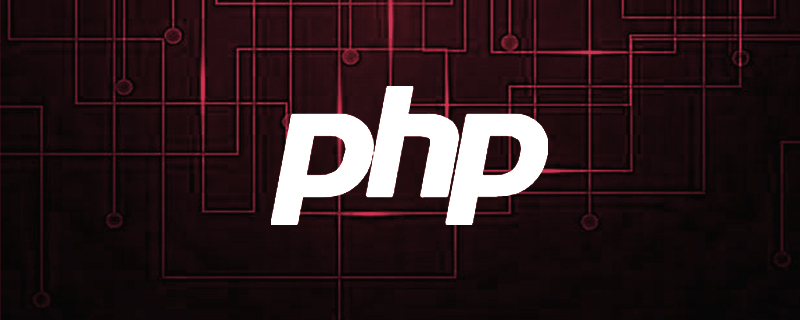
增加元素的方法:1、使用array_push()函数,语法“array_push(二维数组,值1,值2...);”;2、使用array_splice()函数,语法“array_splice(二维数组,count(二维数组),0,元素值)”。
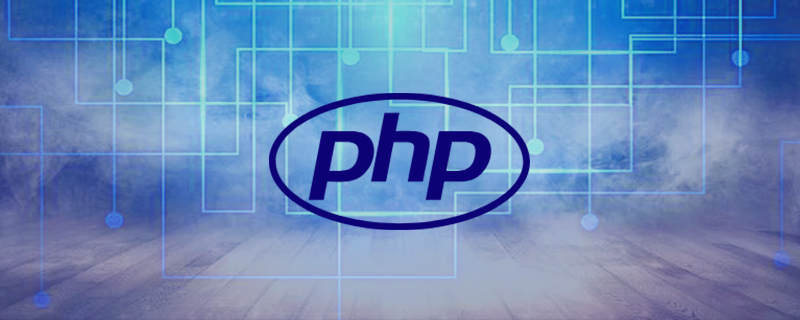
获取方法:1、用“array_values(数组)”将指定数组转为索引数组;2、用“array_search(数值,索引数组)”,在索引数组中搜索数值,返回对应的索引值(下标);3、用“索引值+1”语句获取元素在数组中的位置值。
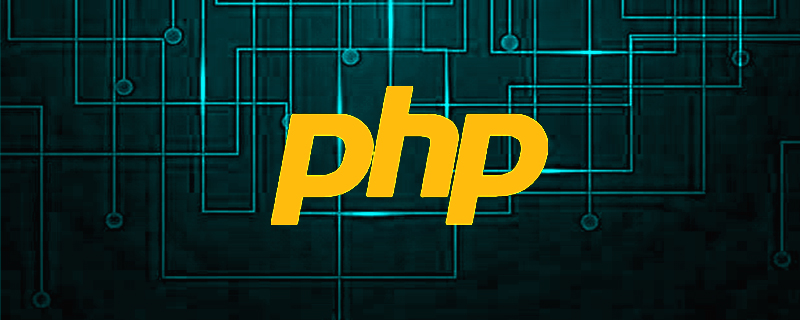
php数组里面可以放数组。PHP数组可以存储所有类型的数据,当然也包括数组本身;如果一个数组中的元素是另一个数组,就构成了包含数组的数组,即多维度数组。数组的不同维度标志着需用几个下标(索引)来获取对应的数组元素,比如二维数组需用两个下标。
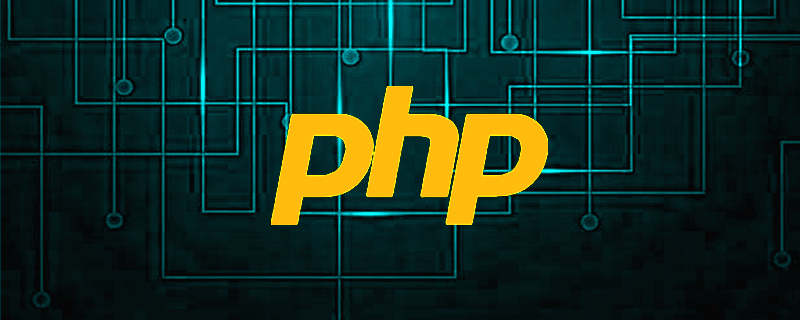
方法:1、循环遍历数组,语法“foreach($arr as $k=>$v){}”;2、循环体中,用“==”判断元素值是否为null,如果是则用unset()删除该元素,语法“if($v==null){unset($arr[$k]);}”。
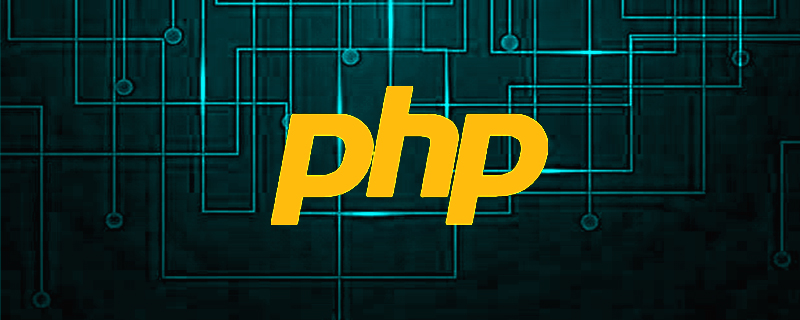
php中只比较值的数组交集函数是“array_intersect()”;该函数用于比较两个(或更多个)数组的键值,语法“array_intersect(数组1,数组2...)”,会返回一个交集数组,所包含的值是从被比较的数组(数组1)中取。
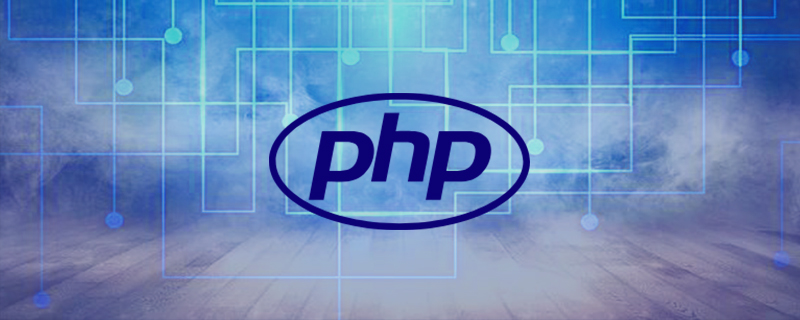
去掉方法:1、用“sort($arr)”对数组进行升序排序,排序后该数组的第一个元素就是最小值,最后一个元素就是最大值;2、用“array_pop($arr)”删除最后一个元素,用“array_shift($arr)”删除第一个元素即可。
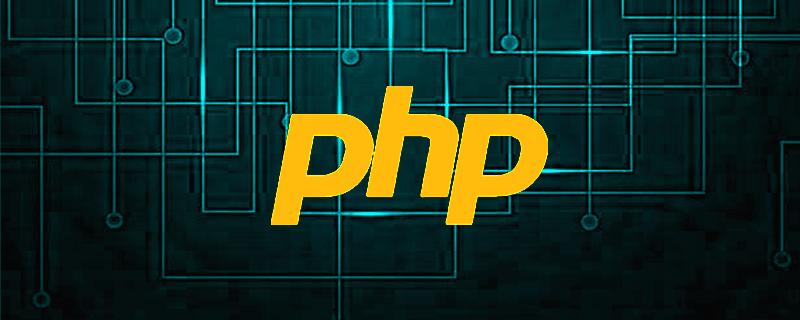
方法:1、对数组进行升序排序,并用“array_pop(数组)”和“array_shift(数组)”去除最大值和最小值;2、用“count(数组)”和“array_sum(数组)”获取数组长度与元素和;3、用“元素和/数组长度”计算平均数。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version
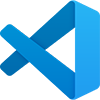
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Atom editor mac version download
The most popular open source editor
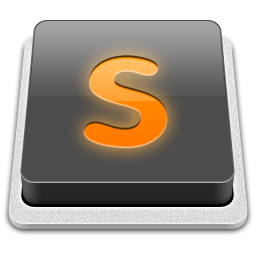
SublimeText3 Mac version
God-level code editing software (SublimeText3)
