Vue is a modern JavaScript framework for building interactive web applications. Vue's data binding and real-time responsiveness are becoming increasingly popular among developers. In Vue applications, since pictures are one of the common resources, it is also very important to change the src attribute of the picture in the application. This article will introduce how to use Vue to change the src attribute of the img tag.
Using the v-bind directive
Vue provides the v-bind directive to bind HTML attributes to expressions in Vue instances. Therefore, it is very easy to change the src attribute of img tag in Vue application. Here are some examples of using the v-bind directive:
<!-- 在模板中绑定img标签的src属性 --> <img src="/static/imghwm/default1.png" data-src="imageSrc" class="lazy" v-bind: alt="How to change the src attribute of img in vue" > <!-- 绑定一个计算属性的返回值到img标签的src属性 --> <img src="/static/imghwm/default1.png" data-src="getImageSrc" class="lazy" v-bind: alt="How to change the src attribute of img in vue" > <!-- 在组件中使用props来绑定img标签的src属性 --> <my-image v-bind:src="imageSrc"></my-image>
In these examples, we use the v-bind directive to bind the data of the Vue instance to the src attribute of the img tag. Among them, the v-bind:src directive binds the expression imageSrc to the src attribute of the img tag.
Computed properties
Vue also provides computed properties to reuse logic, which is also very useful for changing the src attribute of the img tag. For example, we can create a computed property that changes the src attribute of the img tag based on the state of the Vue instance. Here's an example:
<!-- 模板代码 --> <div> <button v-on:click="selectImage(1)">Image 1</button> <button v-on:click="selectImage(2)">Image 2</button> <button v-on:click="selectImage(3)">Image 3</button> <img src="/static/imghwm/default1.png" data-src="selectedImageSrc" class="lazy" v-bind: alt="How to change the src attribute of img in vue" > </div> <!-- Vue组件代码 --> <script> export default { data() { return { selectedImage: 1, imageUrls: [ 'https://example.com/image1.jpg', 'https://example.com/image2.jpg', 'https://example.com/image3.jpg' ] } }, methods: { selectImage(index) { this.selectedImage = index; } }, computed: { selectedImageSrc() { return this.imageUrls[this.selectedImage - 1]; } } } </script>
In this example, we've created three buttons to let the user select the image they want to see. Each button triggers the selectImage method and saves the selected image index into the selectedImage property of the Vue instance. We define a computed property selectedImageSrc that returns the corresponding image URL based on the selected image index. Finally, in the template we use the v-bind directive to bind the selectedImageSrc calculated property to the src attribute of the img tag.
Dynamic Components
Dynamic components are useful if your application loads components dynamically or needs to change components based on different routes or user interactions. Like other Vue components, dynamic components can dynamically bind props and any HTML attribute, including the src attribute of the img tag.
Here is an example:
<template> <div> <button v-on:click="selectImage('https://example.com/image1.jpg')">Image 1</button> <button v-on:click="selectImage('https://example.com/image2.jpg')">Image 2</button> <button v-on:click="selectImage('https://example.com/image3.jpg')">Image 3</button> <component v-bind:is="selectedImageComponent" v-bind:image-src="selectedImage" /> </div> </template> <script> import ImageOne from './ImageOne.vue' import ImageTwo from './ImageTwo.vue' import ImageThree from './ImageThree.vue' export default { data() { return { selectedImage: 'https://example.com/image1.jpg', selectedImageComponent: 'image-one' } }, methods: { selectImage(url) { this.selectedImage = url; switch (url) { case 'https://example.com/image1.jpg': this.selectedImageComponent = 'image-one'; break; case 'https://example.com/image2.jpg': this.selectedImageComponent = 'image-two'; break; case 'https://example.com/image3.jpg': this.selectedImageComponent = 'image-three'; break; default: this.selectedImageComponent = null; } } }, components: { ImageOne, ImageTwo, ImageThree } } </script>
In this example, we provide three buttons to let the user select the picture to be displayed. Each button triggers the selectImage method and saves the selected image URL to the selectedImage property of the Vue instance. We then use a switch statement to select the component name to display based on the selected image URL. Finally, in the template we use the v-bind directive to bind the selectedImage to the image-src attribute of each component, and the v-bind:is directive to dynamically place the components into the application.
In general, it is very easy to change the src attribute of the img tag in Vue. Whether using the v-bind directive, computed properties, or dynamic components, Vue provides simple and powerful ways to deal with this problem. So, in your next Vue application, try using these methods to manage images more easily and efficiently.
The above is the detailed content of How to change the src attribute of img in vue. For more information, please follow other related articles on the PHP Chinese website!

Using ID selectors is not inherently bad in CSS, but should be used with caution. 1) ID selector is suitable for unique elements or JavaScript hooks. 2) For general styles, class selectors should be used as they are more flexible and maintainable. By balancing the use of ID and class, a more robust and efficient CSS architecture can be implemented.

HTML5'sgoalsin2024focusonrefinementandoptimization,notnewfeatures.1)Enhanceperformanceandefficiencythroughoptimizedrendering.2)Improveaccessibilitywithrefinedattributesandelements.3)Addresssecurityconcerns,particularlyXSS,withwiderCSPadoption.4)Ensur

HTML5aimedtoimprovewebdevelopmentinfourkeyareas:1)Multimediasupport,2)Semanticstructure,3)Formcapabilities,and4)Offlineandstorageoptions.1)HTML5introducedandelements,simplifyingmediaembeddingandenhancinguserexperience.2)Newsemanticelementslikeandimpr

IDsshouldbeusedforJavaScripthooks,whileclassesarebetterforstyling.1)Useclassesforstylingtoallowforeasierreuseandavoidspecificityissues.2)UseIDsforJavaScripthookstouniquelyidentifyelements.3)Avoiddeepnestingtokeepselectorssimpleandimproveperformance.4

Classselectorsareversatileandreusable,whileidselectorsareuniqueandspecific.1)Useclassselectors(denotedby.)forstylingmultipleelementswithsharedcharacteristics.2)Useidselectors(denotedby#)forstylinguniqueelementsonapage.Classselectorsoffermoreflexibili

IDsareuniqueidentifiersforsingleelements,whileclassesstylemultipleelements.1)UseIDsforuniqueelementsandJavaScripthooks.2)Useclassesforreusable,flexiblestylingacrossmultipleelements.

Using a class-only selector can improve code reusability and maintainability, but requires managing class names and priorities. 1. Improve reusability and flexibility, 2. Combining multiple classes to create complex styles, 3. It may lead to lengthy class names and priorities, 4. The performance impact is small, 5. Follow best practices such as concise naming and usage conventions.

ID and class selectors are used in CSS for unique and multi-element style settings respectively. 1. The ID selector (#) is suitable for a single element, such as a specific navigation menu. 2.Class selector (.) is used for multiple elements, such as unified button style. IDs should be used with caution, avoid excessive specificity, and prioritize class for improved style reusability and flexibility.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
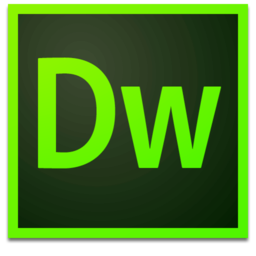
Dreamweaver Mac version
Visual web development tools
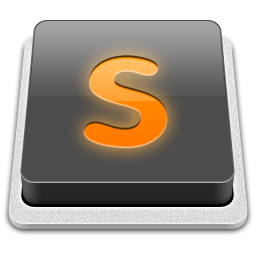
SublimeText3 Mac version
God-level code editing software (SublimeText3)
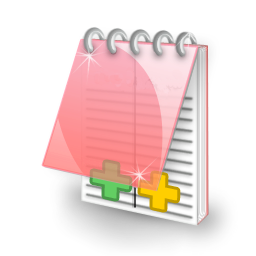
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
