1. Question
Input : 1, 2, 3, 4, 5 Output :5, 4, 3, 2, 1
Input : 10, 20, 30, 40 Output : 40, 30, 20, 10
2. Method
Method 1: Use temporary array
-
Enter the size of the array and the elements of the array.
Consider a function reverse, which accepts parameters - an array (such as arr) and the size of the array (such as n)
In Inside the function, a new array (with the array size of the first array, arr) is initialized.
Array arr[] starts iteration from the first element, and each element of array arr[] is put into a new array from the back, that is, the new array starts iteration from the last element.
This puts all the elements of the array arr[] into the new array in reverse order.
public class reverseArray { // function that reverses array and stores it // in another array static void reverse(int a[], int n) { int[] b = new int[n]; int j = n; for (int i = 0; i < n; i++) { b[j - 1] = a[i]; j = j - 1; } // printing the reversed array System.out.println("Reversed array is: \n"); for (int k = 0; k < n; k++) { System.out.println(b[k]); } } public static void main(String[] args) { int [] arr = {10, 20, 30, 40, 50}; reverse(arr, arr.length); } }
The results are as follows:
Reversed array is: 50 40 30 20 10
Method 2: Use exchange
to exchange the elements of the array. The first element is swapped with the last element. The second element is swapped with the last element, and so on. For example, suppose you have an array with elements [1, 2, 3, ..., n-2, n-1, n]. Swap 1 and n, 2 and n-1, 3 and n-2, etc. one by one.
public class Test { public static void main(String[] args) { int [] arr = {10, 20, 30, 40, 50}; reverse(arr,arr.length); } static void reverse(int[] a,int n) { int i, k, t; for (i = 0; i < n / 2; i++) { t = a[i]; a[i] = a[n - i - 1]; a[n - i - 1] = t; } System.out.println("Reversed array is: \n"); for (k = 0; k < n; k++) { System.out.println(a[k]); } } }
The results are as follows:
Reversed array is: 50 40 30 20 10
Method 3: Use Collections.reverse() method
The method java.util.Collections.reverse(List list) reverses a given list .. Reverse an array by converting it to a list and then calling Collections.reverse(list).
public class Test { public static void main(String[] args) { Integer [] arr = {10, 20, 30, 40, 50}; reverse(arr); } static void reverse(Integer[] a) { Collections.reverse(Arrays.asList(a)); System.out.println(Arrays.asList(a)); } }
The results are as follows:
[50, 40, 30, 20, 10]
Method 4: Use StringBuilder.append() method
If you are using a String array, we can use StringBuilder and for each array A for loop is attached to the elements, starting from the length of the array and decrementing it, converting the StringBuilder to a string, and then splitting back into the array.
public class Test { public static void main(String[] args) { String[] arr = {"Hello", "World"}; StringBuilder reversed = new StringBuilder(); for (int i = arr.length-1; i >= 0; i--) { reversed.append(arr[i]).append(" "); }; String[] reversedArray = reversed.toString().split(" "); System.out.println(Arrays.toString(reversedArray)); } }
The results are as follows:
[World, Hello]
The above is the detailed content of What are the ways to reverse an array in Java?. For more information, please follow other related articles on the PHP Chinese website!
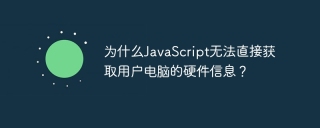
Discussion on the reasons why JavaScript cannot obtain user computer hardware information In daily programming, many developers will be curious about why JavaScript cannot be directly obtained...
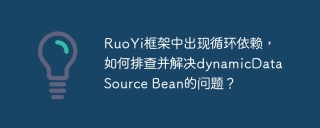
RuoYi framework circular dependency problem troubleshooting and solving the problem of circular dependency when using RuoYi framework for development, we often encounter circular dependency problems, which often leads to the program...

About SpringCloudAlibaba microservices modular development using SpringCloud...
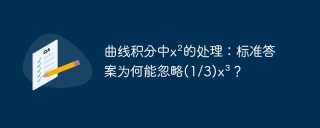
Questions about a curve integral This article will answer a curve integral question. The questioner had a question about the standard answer to a sample question...
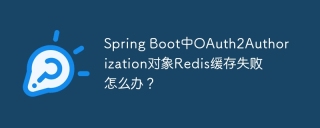
In SpringBoot, use Redis to cache OAuth2Authorization object. In SpringBoot application, use SpringSecurityOAuth2AuthorizationServer...
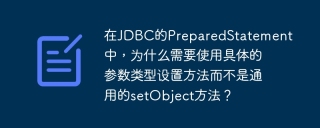
JDBC...
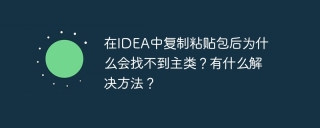
Why can't the main class be found after copying and pasting the package in IDEA? Using IntelliJIDEA...
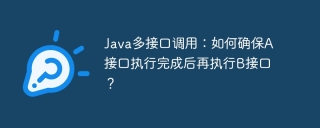
State synchronization between Java multi-interface calls: How to ensure that interface A is called after it is executed? In Java development, you often encounter multiple calls...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
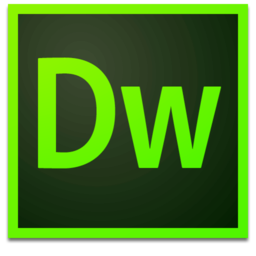
Dreamweaver Mac version
Visual web development tools
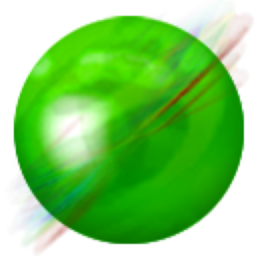
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
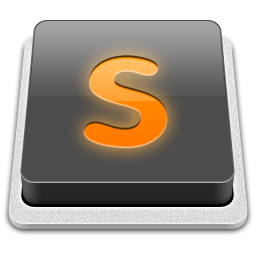
SublimeText3 Mac version
God-level code editing software (SublimeText3)