PHP is a server-side programming language that can implement many functions in web applications. In this article, we will take an in-depth look at the basics of PUT requests and responses in PHP, which is very important for web developers.
PUT request is an HTTP request method, which means it is used to send information to the server. The main purpose of PUT request is to transfer data to the server. Using PUT request is very useful when we need to update or modify data to the server.
In PHP, we can use the curl library to send PUT requests. The curl library provides a simple way to send HTTP requests to the server. It is not only easy to use, but also very flexible. The following is a sample code to send a PUT request using the curl library:
<?php //设置数据项 $data = array('name' => 'John Doe', 'email' => 'johndoe@example.com'); //将数据转换为JSON格式 $data_string = json_encode($data); //设置请求URL $url = 'http://example.com/api/user/1'; //初始化curl请求 $curl = curl_init(); //设置curl选项 curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_CUSTOMREQUEST, "PUT"); curl_setopt($curl, CURLOPT_POSTFIELDS, $data_string); curl_setopt($curl, CURLOPT_RETURNTRANSFER, true); curl_setopt($curl, CURLOPT_HTTPHEADER, array( 'Content-Type: application/json', 'Content-Length: ' . strlen($data_string)) ); //执行curl请求 $response = curl_exec($curl); //关闭curl请求 curl_close($curl); //输出响应信息 echo $response; ?>
In the above code, we first set the data items and convert them into JSON format using the json_encode function. Then, we set the requested URL, initialize the request using the curl_init function, set the curl options through the curl_setopt function, and finally use the curl_exec function to execute the request and output the response information.
When PHP processes the PUT response, we need to parse the data returned by the server. Normally, the server will return the data in the form of JSON. We can use PHP's own json_decode function to parse JSON data. The following is a sample code that uses the json_decode function to parse JSON data:
<?php //发送PUT请求到服务器 //..... //处理服务器返回的JSON数据 $json = '{"name": "John Doe", "email": "johndoe@example.com"}'; $data = json_decode($json, true); echo $data['name']; //输出John Doe echo $data['email']; //输出johndoe@example.com ?>
In the above code, we first simulated the JSON data returned by the server, and then used the json_decode function to parse it into a PHP array. We can then get the value by the key of the data item just like we do with PHP arrays.
To summarize, PUT requests and responses are very basic and important in Web development. Using the curl library can help us implement PUT requests and process PUT responses quickly and easily. I hope this PHP introductory guide can help everyone better understand the basic concepts of PUT requests and responses.
The above is the detailed content of Getting Started with PHP: PUT Requests and Responses. For more information, please follow other related articles on the PHP Chinese website!
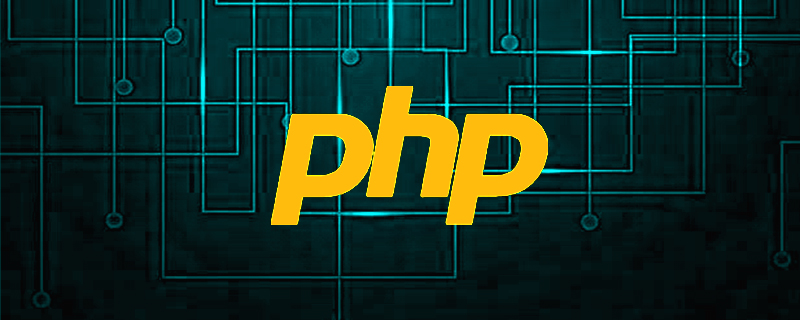
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
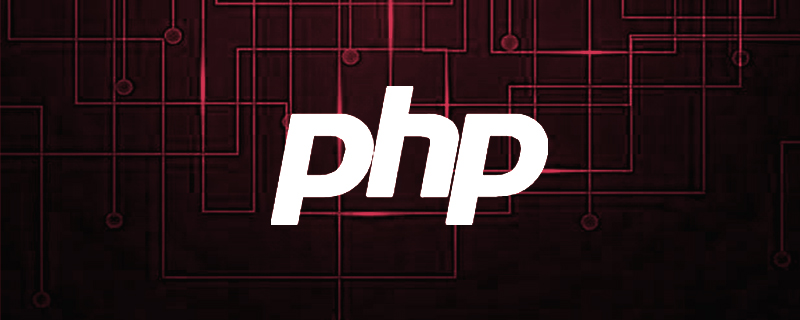
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
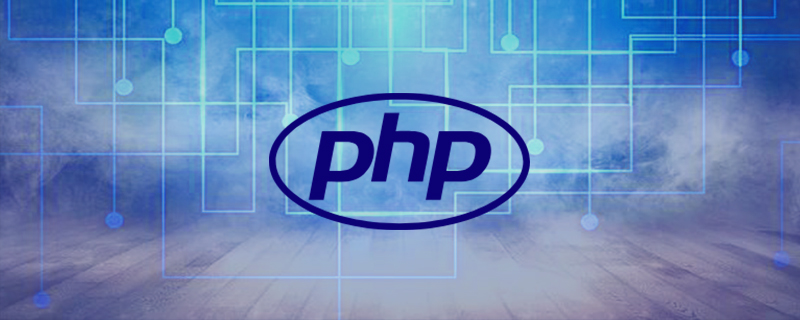
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
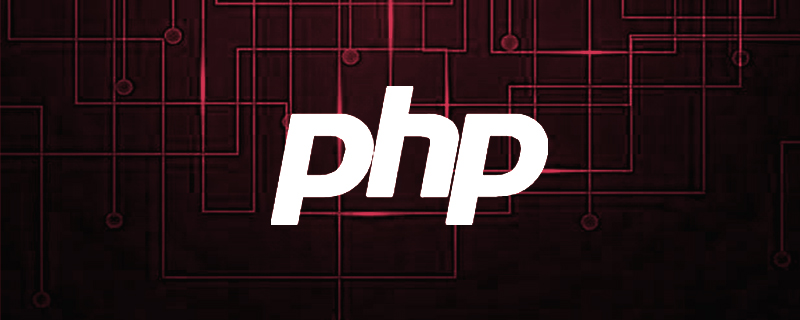
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
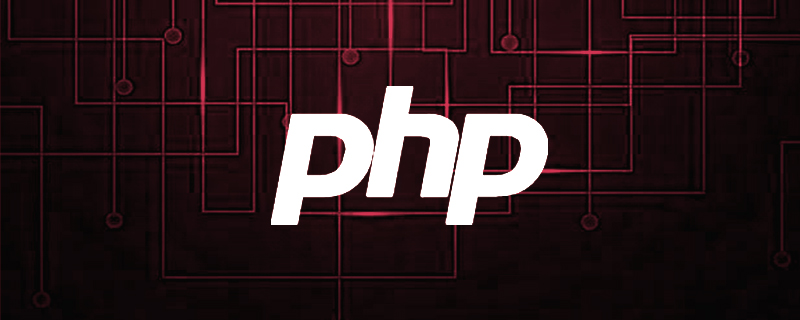
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
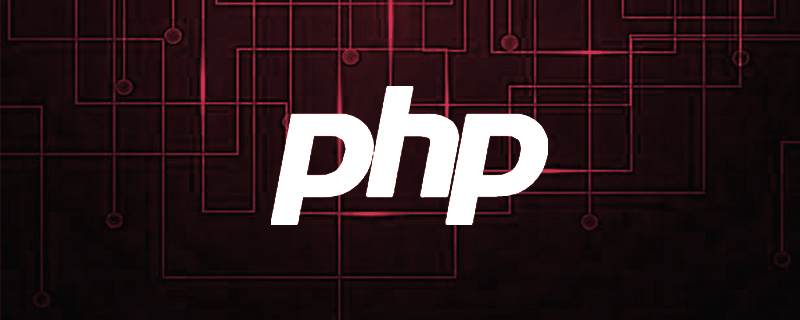
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。
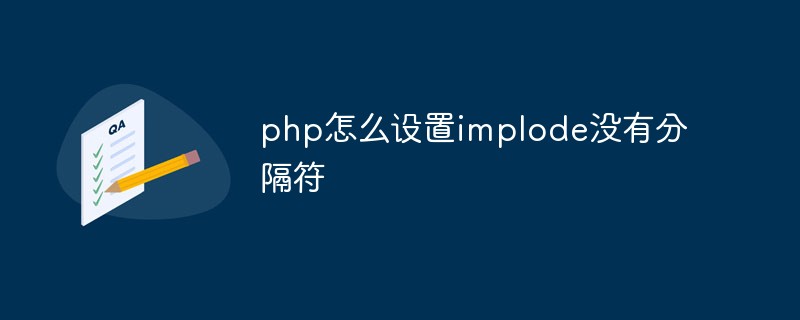
在PHP中,可以利用implode()函数的第一个参数来设置没有分隔符,该函数的第一个参数用于规定数组元素之间放置的内容,默认是空字符串,也可将第一个参数设置为空,语法为“implode(数组)”或者“implode("",数组)”。
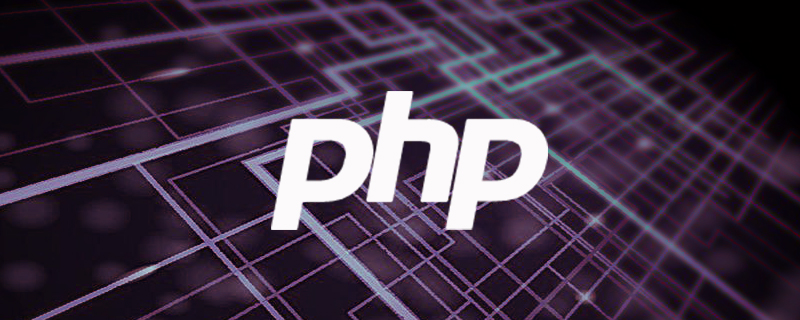
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
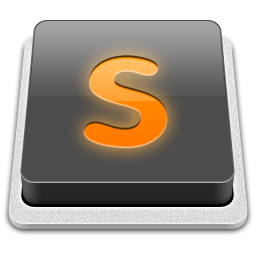
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
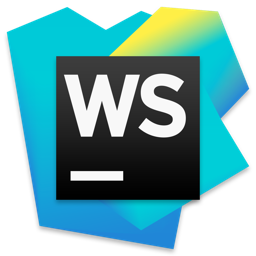
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
