


With the popularity of the Internet and the development of e-commerce, email has become the most commonly used communication method for people. However, email security issues have gradually come to the fore. In order to avoid the leakage of sensitive information and the breeding of spam, we need to perform security verification on emails. This article will introduce you to the detailed steps to implement the email security verification function in PHP.
1. Principle of email security verification
The principle of email security verification is to use public key encryption technology and digital signature technology to verify the key encryption and digital signature of the email. Security verification of emails.
2. Steps for PHP to implement email security verification
1. Generate public and private keys
First, we need to generate public and private keys. The public key is public and is used to encrypt emails; the private key is secret and is used to decrypt emails. You can use the openssl extension library to generate public and private keys:
$key = openssl_pkey_new(array( "digest_alg" => "sha512", "private_key_bits" => 2048, "private_key_type" => OPENSSL_KEYTYPE_RSA, ));
The generated $key array contains the public key and private key, where the public key is:
$keyDetails = openssl_pkey_get_details($key); $publicKey = $keyDetails["key"];
The private key is:
openssl_pkey_export($key, $privateKey);
2. Encrypted email
After the email is encrypted using the public key, only the holder of the private key can decrypt it. You can use the openssl_public_encrypt function in the openssl extension library to implement email encryption:
openssl_public_encrypt($message, $encrypted, $publicKey);
where $message is the email content to be encrypted, and $encrypted is the encrypted result.
3. Digital signature
Digital signature is an important means to verify the authenticity of emails. You can use the openssl_sign function in the openssl extension library to implement digital signature:
openssl_sign($message, $signature, $privateKey, OPENSSL_ALGO_SHA512);
where $ message is the content of the email to be signed, and $signature is the signed result.
4. Verify the email
After the recipient receives the email, he needs to verify the email, including decrypting the ciphertext of the email and verifying whether the digital signature of the email is correct. You can use the openssl_private_decrypt function in the openssl extension library to decrypt the email:
openssl_private_decrypt($encrypted, $decrypted, $privateKey);
where $encrypted is the encrypted email content and $decrypted is the decrypted result.
You also need to use the openssl_verify function in the openssl extension library to verify the digital signature of the email:
openssl_verify($message, $signature, $publicKey, OPENSSL_ALGO_SHA512);
where $message is the email content, $signature is the digital signature, and $publicKey is the public key .
5. Complete code
The following is a complete PHP code example:
// 生成公钥和私钥 $key = openssl_pkey_new(array( "digest_alg" => "sha512", "private_key_bits" => 2048, "private_key_type" => OPENSSL_KEYTYPE_RSA, )); $keyDetails = openssl_pkey_get_details($key); $publicKey = $keyDetails["key"]; openssl_pkey_export($key, $privateKey); // 加密邮件 $message = "Hello, this is a test message."; openssl_public_encrypt($message, $encrypted, $publicKey); // 数字签名 openssl_sign($message, $signature, $privateKey, OPENSSL_ALGO_SHA512); // 验证邮件 openssl_private_decrypt($encrypted, $decrypted, $privateKey); openssl_verify($message, $signature, $publicKey, OPENSSL_ALGO_SHA512);
3. Summary
Email security verification is to ensure that email transmission is safe and authentic important means of sex. This article introduces the detailed steps for PHP to implement email security verification functions, including generating public and private keys, encrypting emails, digital signatures, and verifying emails. Through these steps, we can achieve security verification of emails to ensure that sensitive information is not leaked and transmitted safely.
The above is the detailed content of Detailed steps to implement email security verification function in PHP. For more information, please follow other related articles on the PHP Chinese website!
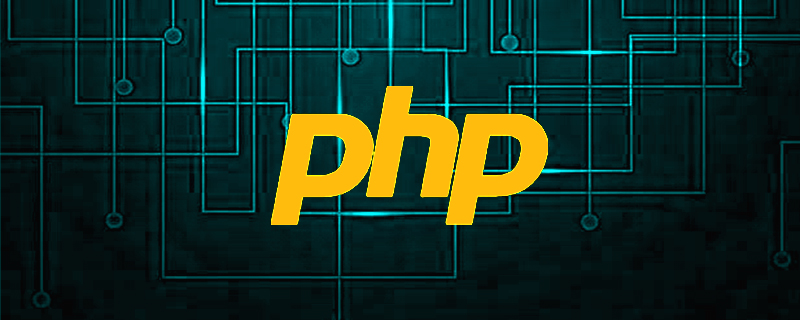
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
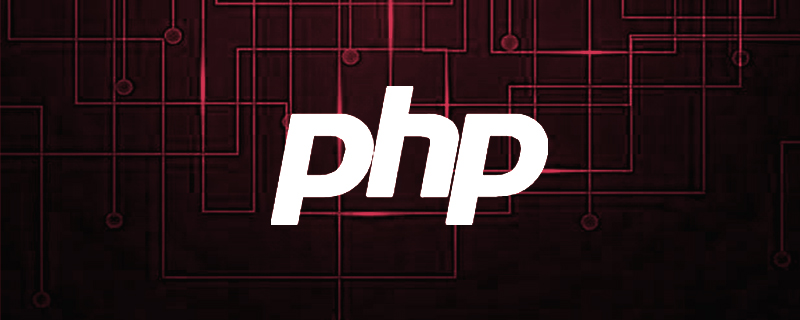
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
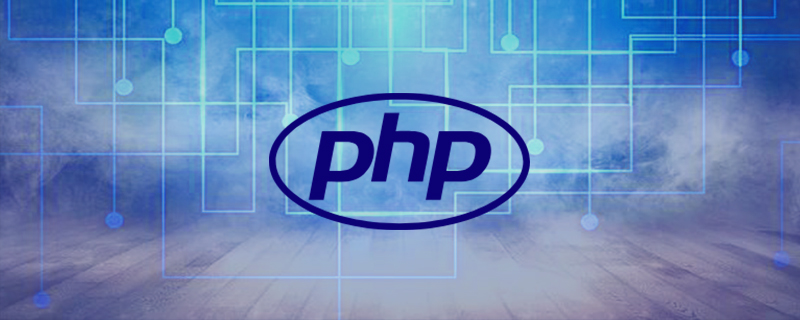
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
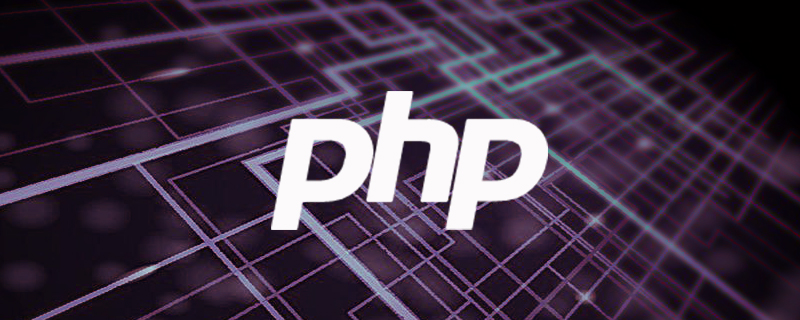
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
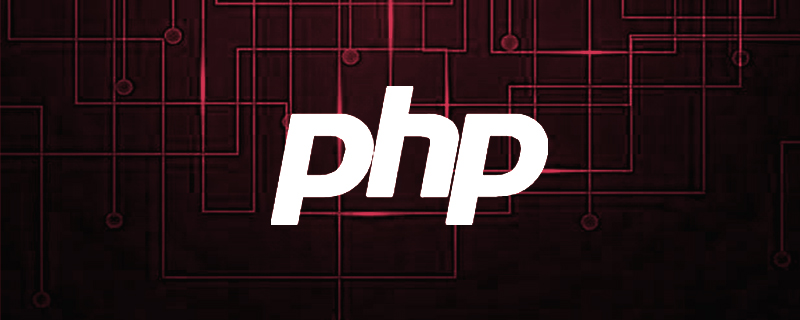
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
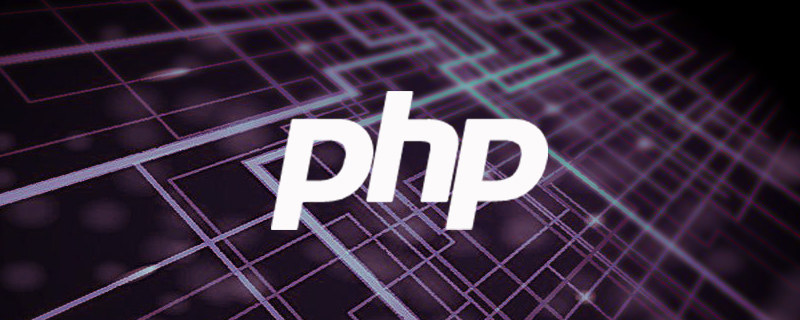
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
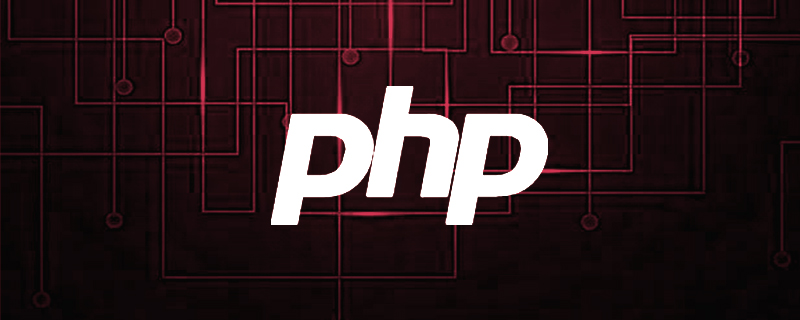
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
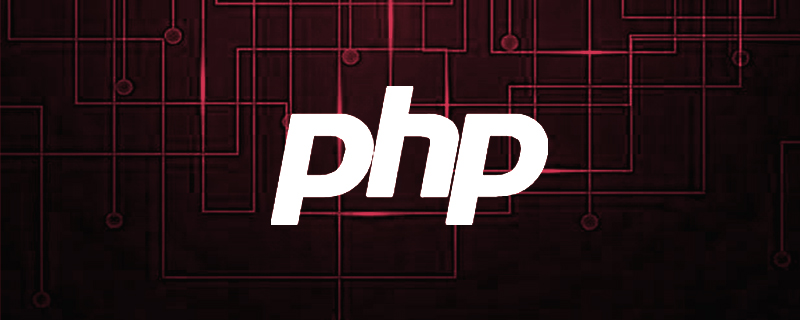
查找方法:1、用strpos(),语法“strpos("字符串值","查找子串")+1”;2、用stripos(),语法“strpos("字符串值","查找子串")+1”。因为字符串是从0开始计数的,因此两个函数获取的位置需要进行加1处理。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
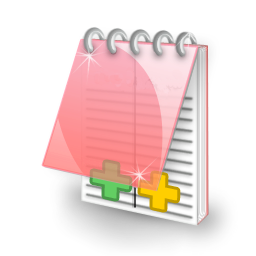
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
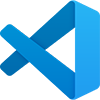
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
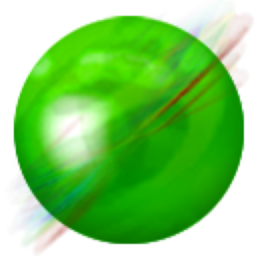
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
