In web development, PHP and JavaScript are the two most commonly used programming languages. Moreover, PHP and JavaScript have their own array types and cannot be used with each other. Therefore, the PHP array needs to be converted into a JavaScript array on the front end.
The following will introduce several methods of converting PHP arrays to JavaScript arrays.
Method 1: Manual conversion
Manual conversion is the most basic method and the easiest to operate. Assign the data in the PHP array to the JavaScript array one by one, as follows:
<?php $php_array = array(1,2,3); ?> <script type="text/javascript"> var js_array = new Array(); <?php foreach($php_array as $value) { echo "js_array.push('$value');"; } ?> console.log(js_array); </script>
In the above code, a PHP array is first defined, and then the data in the PHP array is assigned one by one through a foreach loop in the JavaScript code block. Assign the value to a JavaScript array and output it to the console.
Although this method is simple and easy to understand, it is less efficient when the amount of data in the PHP array is large.
Method 2: Use the json_encode() function
The json_encode() function can convert PHP arrays into JSON format data, and JavaScript can easily convert JSON data into arrays. The detailed usage is as follows:
<?php $php_array = array(1,2,3); $json_string = json_encode($php_array); ?> <script type="text/javascript"> var js_array = JSON.parse('<?php echo $json_string; ?>'); console.log(js_array); </script>
In the above code, first use the json_encode() function to convert the PHP array into JSON format data, and then use the JSON.parse() function in the JavaScript code block to convert the JSON data into JavaScript array and output to the console.
This method has less redundant code and is more efficient. It is a more suitable method to use.
Method 3: Use Ajax asynchronous request
When you need to pass a PHP array to another page, you can use Ajax asynchronous request. Convert the array into JSON format in the PHP file, and then pass the JSON data to the JavaScript page through an Ajax asynchronous request. Detailed usage is as follows:
<?php $php_array = array(1,2,3); $json_string = json_encode($php_array); ?> <script type="text/javascript"> $(function() { $.ajax({ type: "POST", url: "your_url.php", data: {json: '<?php echo $json_string; ?>'}, success: function(response) { var js_array = JSON.parse(response); console.log(js_array); } }); }); </script>
In the above code, an asynchronous request is sent through jQuery's ajax() function to transfer JSON data to the specified PHP file. Parse JSON data in PHP files and output the results through echo.
Finally, receive the JSON data returned by the PHP file through JavaScript code, convert it into a JavaScript array, and output it to the console.
Summary
The above are three methods of converting PHP arrays into JavaScript arrays. Method 1 is the most basic method, but it is more time-consuming. Method 2 uses the json_encode() function, which is highly efficient and has less code redundancy. Method 3 is suitable for passing a PHP array to another page. According to different needs, different methods can be chosen to achieve it.
The above is the detailed content of Convert php array to js array. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use
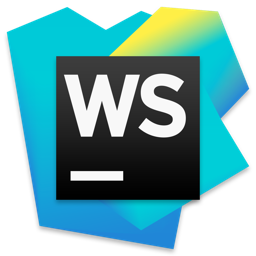
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
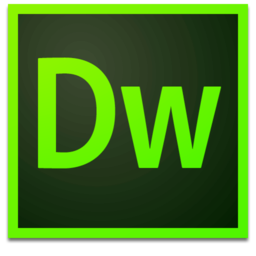
Dreamweaver Mac version
Visual web development tools
