In PHP development, scenes that often involve operating arrays are involved. However, sometimes we encounter some null values in the array, and these null values will affect our processing of data. Therefore, we need to remove the null values in these arrays. In this article, we will introduce how to use PHP to remove null values from an array.
- Use the array_filter function
The array_filter function is a built-in function in PHP, which can filter the data in the array and retain the required data. When operating an array, we can use the array_filter function to filter null values in the array. The following is a sample code:
$arr = array("", "apple", "banana", "", "pear"); $result = array_filter($arr); print_r($result);
The result of running the code is as follows:
Array ( [1] => apple [2] => banana [4] => pear )
You can see that after using the array_filter function, the null values in the array have been removed.
- Using foreach loop
In addition to using the array_filter function, we can also use foreach loop to remove null values in the array. The specific implementation is as follows:
$arr = array("", "apple", "banana", "", "pear"); $result = array(); foreach($arr as $value) { if(!empty($value)) { $result[] = $value; } } print_r($result);
The code running result is as follows:
Array ( [0] => apple [1] => banana [2] => pear )
In this example code, we first define an empty array $result, and then traverse each element in the original array $arr through a foreach loop elements, if the current element is not empty, add it to the $result array.
- Use the array_map function
In addition to the above two methods, we can also use the array_map function to remove null values in the array. The array_map function can execute a callback function for each element in the array, and the return value of the callback function is the processed new element value. The following is a sample code:
$arr = array("", "apple", "banana", "", "pear"); $result = array_map("trim", $arr); $result = array_filter($result); print_r($result);
The code running results are as follows:
Array ( [1] => apple [2] => banana [4] => pear )
In this sample code, we use two functions: array_map and array_filter. First, we use the array_map function to perform the trim function on each element in the array to remove spaces. Then, we use the array_filter function to remove null values in the array.
Summary
Removing null values in an array is a problem often encountered in PHP. We can easily implement this function by using methods such as array_filter, foreach loop, and array_map. In actual development, we should choose an appropriate method to handle null values in the array according to the actual situation to achieve the optimal effect.
The above is the detailed content of php removes array as empty. For more information, please follow other related articles on the PHP Chinese website!
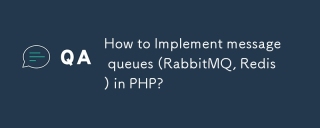
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
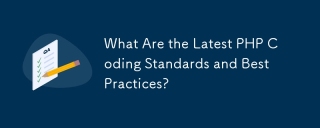
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
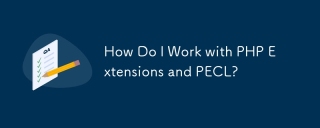
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
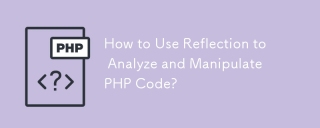
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
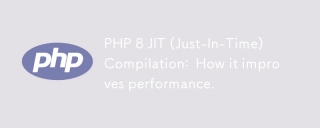
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
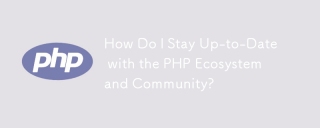
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
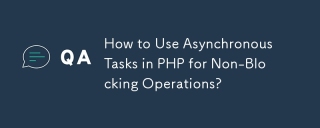
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
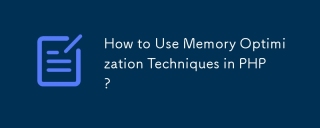
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor
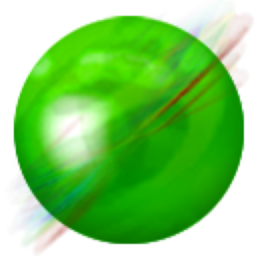
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
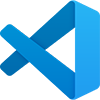
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
