PHP is a scripting language widely used in Web programming that supports a variety of data types and data structures. In PHP, arrays are one of the most commonly used data types, and three-dimensional arrays are a very common data structure. This article will introduce how to traverse a three-dimensional array in PHP.
1. What is a three-dimensional array?
In PHP, an array is a data structure that can hold multiple values. Arrays can be one-dimensional or multi-dimensional. Generally speaking, what we call "arrays" are one-dimensional arrays. For example, the following is an example of a one-dimensional array:
$fruit = array("apple", "banana", "orange");
And if we combine multiple one-dimensional arrays and add multiple elements to each one-dimensional array, then we get a two-dimensional array dimensional array. The following is an example of a two-dimensional array:
$food = array( array("apple", "banana", "orange"), array("steak", "chicken", "fish") );
In this example, we combine two one-dimensional arrays into a two-dimensional array. There are two elements in the $food array, each element is a one-dimensional array.
Similarly, if we combine multiple two-dimensional arrays and add multiple elements to each two-dimensional array, then we get a three-dimensional array. The following is an example of a three-dimensional array:
$store = array( array( array("apple", 2), array("banana", 3), array("orange", 1) ), array( array("steak", 5), array("chicken", 7), array("fish", 2) ) );
In this example, we combine two two-dimensional arrays into a three-dimensional array. There are two elements in the $store array, each element is a two-dimensional array.
2. How to traverse a three-dimensional array?
In PHP, to traverse an array, we usually use loop statements such as for and foreach.
For a three-dimensional array, we need to use multiple nested loop statements to access each element. The following is a sample code for traversing a three-dimensional array:
$store = array( array( array("apple", 2), array("banana", 3), array("orange", 1) ), array( array("steak", 5), array("chicken", 7), array("fish", 2) ) ); for ($i = 0; $i < count($store); $i++) { for ($j = 0; $j < count($store[$i]); $j++) { for ($k = 0; $k < count($store[$i][$j]); $k++) { echo $store[$i][$j][$k] . " "; } } }
In this example, we use three for loop statements. The $i loop variable is used to access the first level array, the $j loop variable is used to access the second level array, and the $k loop variable is used to access the third level array. Finally, we use the echo statement to output the value of each element.
3. Notes
When traversing a three-dimensional array, we need to pay attention to the following points:
- When using the count() function to calculate the length of the array, Just calculate the length of the outermost array.
- When traversing the array, the initial value of the loop variable should be 0, not 1. Because array subscripts start from 0.
- Nested loops of three-dimensional arrays will lead to reduced efficiency, so when processing large amounts of data, you should try to use other data structures, such as databases, etc.
4. Summary
In PHP, nested loop statements are required to traverse a three-dimensional array. Although using three-dimensional arrays can easily organize and access data, it can also easily reduce the efficiency of the program. Therefore, when processing large amounts of data, we should choose more appropriate data structures, such as databases, etc.
The above is the detailed content of Is php traversing a three-dimensional array?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
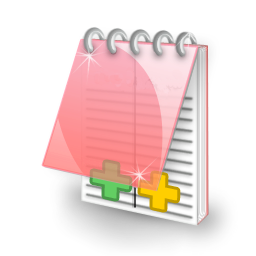
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
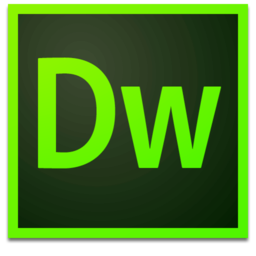
Dreamweaver Mac version
Visual web development tools
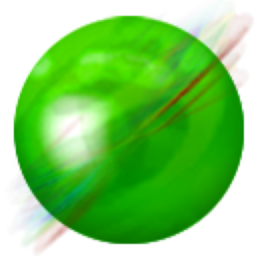
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
