Number processing techniques in PHP
PHP is a widely used programming language that can be used not only for web development and database design, but also for number processing. PHP has some very useful number-crunching techniques that can help developers optimize code performance and improve program readability. This article will introduce some common number processing techniques used in PHP, including:
- Use numerical comparisons instead of string comparisons
PHP can use comparison operators for numerical comparisons Compare with string. If you need to convert a string to a number in your code for comparison, you can use the following code:
if ((int) $var1 === (int) $var2) { // ... }
This will convert the string to an integer and then compare. However, if you are comparing pure numeric strings, you can compare directly using comparison operators, as shown below:
if ($var1 === $var2) { // ... }
This will avoid the additional overhead of converting the string to a number.
- Use bitwise operations instead of other operations
PHP has a rich set of operators and functions, but sometimes using bitwise operators can improve code performance. Bit operators can directly operate on binary bits, so they are more efficient. For example, you can use bitwise operators to perform modulo operations:
$modulus = $num & ($divisor - 1);
This is faster than using the modulo operator %
, especially when the divisor is a power of 2. Additionally, you can use bitwise operators instead of some functions, as shown below:
- Rounding: Use
$num >> 0
instead of(int)$num
- Absolute value: use
($num ^ ($num >> 31)) - ($num >> 31)
instead ofabs()
- Parity judgment: use
$num & 1
instead of$num % 2
It should be noted that, use Bitwise operators need to ensure that code readability is not affected.
- Use ternary operator instead of if-else statement
The if-else statement in PHP can be simplified by ternary operator. The ternary operator is also called the ternary operator, which can use a ternary condition to replace the regular if-else statement. For example, the following if-else statement:
if ($num > 0) { $result = "positive"; } else { $result = "non-positive"; }
can be simplified to:
$result = ($num > 0) ? "positive" : "non-positive";
- Use numeric constants instead of string constants
Can be used in PHP String constants and numeric constants. String constants require string parsing and memory allocation, which may reduce code performance. If you need to use constants in your code, it is recommended to use numeric constants. For example, the following string constant:
define("MODE_DEBUG", "debug");
can be replaced with a numeric constant:
define("MODE_DEBUG", 1);
This will reduce string parsing and memory allocation in the code, thus improving performance.
- Use displacement operations instead of multiplication and division operations
The displacement operations in PHP can be used to replace multiplication and division operations. For example, you can use a left shift operation instead of multiplication, as shown below:
$multiple = $num << 1; // 相当于 $num * 2
You can also use a right shift operation instead of division, as shown below:
$divide = $num >> 1; // 相当于 $num / 2
These tips can help you optimize the performance of your code, But be careful not to over-optimize. When writing code, always prioritize code readability and maintainability. If optimizing your code affects code quality, it's not a good practice.
The above is the detailed content of Number processing techniques in PHP. For more information, please follow other related articles on the PHP Chinese website!
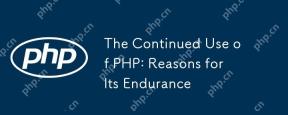
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
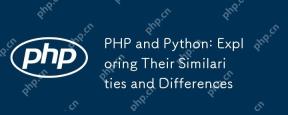
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
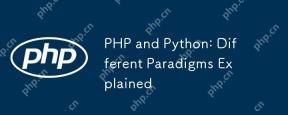
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
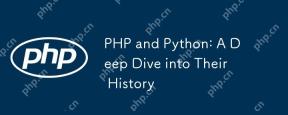
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
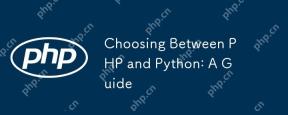
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
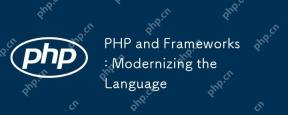
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
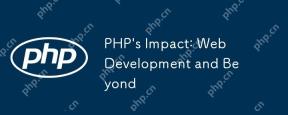
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
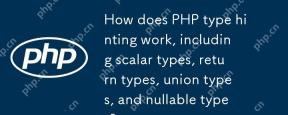
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
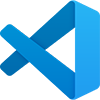
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Linux new version
SublimeText3 Linux latest version
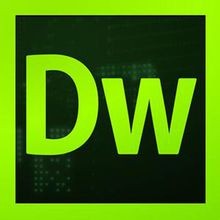
Dreamweaver CS6
Visual web development tools