Multiple processes in PHP
With the development of the Internet, more and more websites need to carry a large number of user access requests. When faced with high concurrency, a single-process server will quickly reach a bottleneck, causing users to be unable to access the website normally. Therefore, multi-process has become one of the effective solutions to solve high concurrency problems. This article will introduce the multi-process technology in PHP to improve the program's ability to handle concurrent requests while ensuring program quality.
1. Introduction to multi-process
In computer science, a process refers to an executing program instance. Each process has its own memory space and system resources. Multiprocessing refers to creating multiple processes in one program. Each process executes independently, has its own process number and process control block, and does not interfere with each other. Multi-process technology is widely used in server software, operating systems and other fields.
2. Multi-process in PHP
- Methods to implement multi-process
To implement multi-process in PHP, you can use pcntl extension or posix extension. Both extensions provide functions such as process creation, inter-process communication, signal handling, etc.
pcntl extension is PHP's process control function library, which provides many functions for creating, operating and managing processes. Using the pcntl function library to implement multiple processes can easily control the parent-child relationship, signal transmission, resource sharing, etc. of the process.
posix extension also provides process control, signal processing and other functions, which is a supplement to the pcntl extension. It also provides more system functions, such as file operations, system information query, etc.
- Create a child process
Create a child process in PHP by using the pcntl_fork() function. The return value of the fork() function is different, which can distinguish the parent process and the child process, thereby realizing the distinction between parent and child processes.
$pid = pcntl_fork(); // 创建子进程 if ($pid == -1) { die('fork failed'); } elseif ($pid == 0) { // 子进程 // 子进程代码 } else { // 父进程 // 父进程代码 }
After the fork() function is called, the operating system will create a new child process. At this time, the main process can continue to execute and the child process will start executing after the fork() function. The pid of the child process is 0, and the pid of the parent process is the pid value of the child process. After creating a subprocess, we can perform some time-consuming operations in the subprocess, or perform some tasks in parallel, thereby improving the concurrency performance of the entire processing system.
- The exit status of the child process
After the child process completes execution, we need to obtain its exit status. In the pcntl_waitpid() function, the first parameter is the process number waiting for the child process to end, and the second parameter is the location where the child process exit status is stored. When the return value is positive, it means that the child process has exited, otherwise it means that it was interrupted by a signal while waiting.
$pid = pcntl_fork(); if ($pid == -1) { die('fork failed'); } elseif ($pid == 0) { // 子进程 exit(0); // 子进程退出状态为0 } else { // 父进程 pcntl_waitpid($pid, $status); // 等待子进程退出 echo $status; // 输出子进程退出状态 }
3. Practical Case
The following uses a simple practical case to illustrate how to use multi-process technology in PHP.
Implement a script to obtain the contents of multiple URLs, obtain the URL contents simultaneously by creating multiple sub-processes, and save their contents to the database after successful acquisition.
<?php // 需要获取的URL列表 $urlList = [ 'https://www.baidu.com/', 'https://www.qq.com/', 'https://www.sina.com.cn/', 'https://www.163.com/', 'https://www.taobao.com/' ]; // 创建多个子进程并行获取URL内容 foreach ($urlList as $url) { $pid = pcntl_fork(); if ($pid == -1) { die('fork failed'); } elseif ($pid == 0) { // 子进程 $content = file_get_contents($url); // 获取URL内容 // 保存内容到数据库 $pdo = new PDO('mysql:host=localhost;dbname=test', 'root', ''); $stmt = $pdo->prepare("INSERT INTO url_content(url, content) VALUES(?, ?)"); $stmt->execute([$url, $content]); exit(0); } } // 等待所有子进程执行完毕 while (pcntl_waitpid(0, $status) != -1) { // do nothing } echo 'All child processes have terminated' . PHP_EOL;
In the above code, we traverse $urllist through foreach and create multiple sub-processes to obtain URL content in parallel. After obtaining the URL content through the file_get_contents() function, we save the content to the database. In the parent process, wait for the child process to complete execution through the while and pcntl_waitpid() functions.
4. Notes
When using multi-process technology, you need to pay attention to the following points:
- The child process must exit before the parent process ends, otherwise it will Create a zombie process.
- The child process will only make a copy when the parent process is created. Content modified by the child process will not affect the parent process, and content modified by the parent process will not affect the child process.
- When the child process is created, all variables and file descriptors of the parent process will be copied, but the streams opened by the parent process will not be copied.
- When using multi-process technology, you need to consider the system resource usage to avoid excessive consumption of system resources.
5. Summary
The application of multi-process technology in PHP can effectively improve the program's ability to handle concurrent requests. Through multi-process technology, multiple tasks can be processed in parallel and the response speed and concurrency of the system can be improved. On the premise of ensuring program quality, multi-process technology provides us with an efficient and simple solution.
The above is the detailed content of Multiple processes in PHP. For more information, please follow other related articles on the PHP Chinese website!
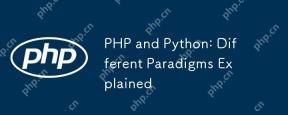
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
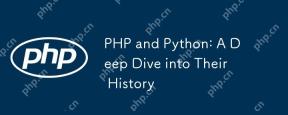
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
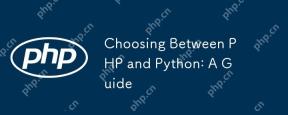
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
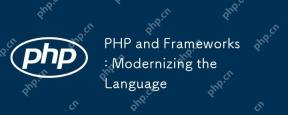
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
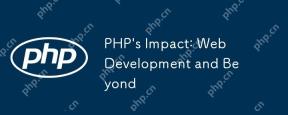
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
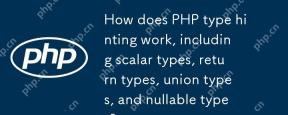
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
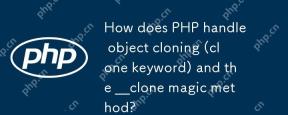
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
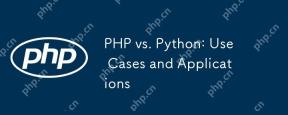
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
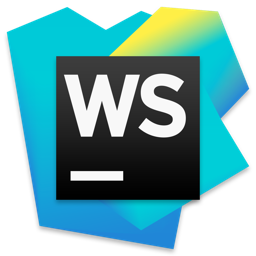
WebStorm Mac version
Useful JavaScript development tools

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.