


How to use PHP to implement the price protection function of the mall
With the continuous development of the e-commerce market, merchants often provide some preferential activities on their mall websites in order to attract more customers and increase sales. Common offers include discounts, gifts, and price reductions. Among them, price protection is a common preferential means, and its main function is to ensure that the price of goods purchased by buyers will not drop within a period of time. In this article, we will introduce how to use PHP to implement the price protection function of the mall.
- Design database table
Create a table named "price_protection" in the MySQL database, which contains the following fields:
- id INT(11): Primary key, self-increasing
- product_id INT(11): Product ID
- old_price FLOAT(10,2): Last price
- new_price FLOAT(10 ,2): New price
- start_time DATETIME: Price protection start time
- end_time DATETIME: Price protection end time
- Implement price protection function
In the product details page of the mall website, add a "Price Protection" button. Whenever the user clicks the button, a PHP script will be triggered that will retrieve price information from the database based on the item ID the user purchased.
If the timestamp stored in the "end_time" field has expired, return the old price, otherwise return the new price. If the old price is empty, it is set to the current price. If the price has dropped, update the old price and set the new price to the old price. If the price has not dropped, only the price protection period is updated.
The following is the PHP script sample code to implement this function:
<?php $mysqli = new mysqli('localhost', 'username', 'password', 'your_database'); $productId = $_GET['product_id']; $currentTime = date('Y-m-d H:i:s'); $sql = "SELECT * FROM price_protection WHERE product_id='$productId' AND end_time>'$currentTime'"; $result = $mysqli -> query($sql); if ($result -> num_rows == 0) { //如果没有价格保护记录,则返回商品原有价格 $sql = "SELECT price FROM products WHERE id='$productId'"; $result = $mysqli -> query($sql); $row = $result -> fetch_assoc(); echo $row['price']; } else { //如果存在价格保护记录,则返回价格保护的价格 $row = $result -> fetch_assoc(); if ($row['old_price'] == null) { $row['old_price'] = $row['new_price']; $sql = "UPDATE price_protection SET old_price=".$row['old_price']." WHERE id=".$row['id']; $mysqli -> query($sql); } echo $row['old_price']; if ($row['new_price'] < $row['old_price']) { $sql = "UPDATE price_protection SET old_price=".$row['new_price'].", new_price=".$row['new_price']." WHERE id=".$row['id']; $mysqli -> query($sql); } else { $sql = "UPDATE price_protection SET start_time='$currentTime', end_time=DATE_ADD('$currentTime', INTERVAL 7 DAY) WHERE id=".$row['id']; $mysqli -> query($sql); } } ?>
- Front-end implementation
In the JavaScript code of the product details page, protect the price A button is added to the page and when clicked it requests the current price from the server. This price should be displayed to the right of the price on the product page and marked with a price protection icon.
The following is the JavaScript sample code to implement the price protection function:
$(document).ready(function() { $.ajax({ url: 'get_price.php', dataType: 'json', success: function(data) { if (data.success) { $('#price').html('<span class="old-price">¥ '+data.data.old_price+'</span>¥ '+data.data.new_price+'<i class="icon-price-protection"></i>'); } else { $('#price').html('<span>¥ '+data.data.price+'</span><i class="icon-price-protection"></i>'); } } }); });
- Summary
This article introduces how to use PHP to implement price protection in the mall Function. Specific implementation methods are given from the aspects of database design, script implementation, front-end implementation, etc. I hope this article can be helpful to developers, implement the discount function of the mall, and provide buyers with a better shopping experience.
The above is the detailed content of How to use PHP to implement the price protection function of the mall. For more information, please follow other related articles on the PHP Chinese website!
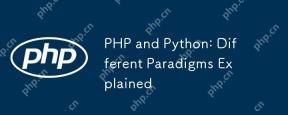
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
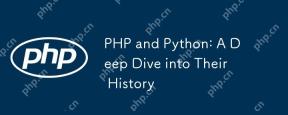
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
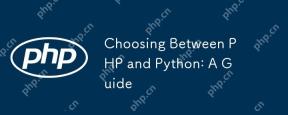
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
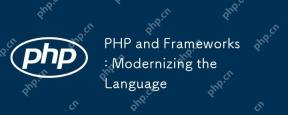
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
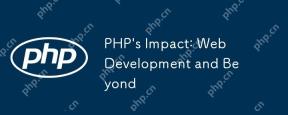
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
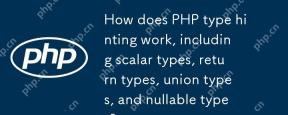
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
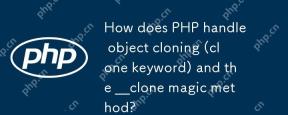
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
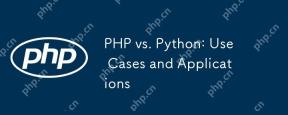
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
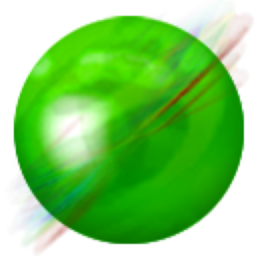
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
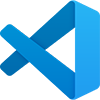
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.