Golang is a modern programming language that has been widely used in many fields through its concurrency capabilities and efficient memory management. In golang, sorting is one of the common operations, and the sorting algorithm is also a relatively basic data structure and algorithm.
Golang provides some built-in sorting functions, such as the common sort.Ints in the sort package, which can sort integer slices. Additionally, we can also use sort.Strings to sort string slices. But in some scenarios, we need to write our own sorting function to meet specific sorting requirements. In this case, we need to understand the sorting algorithm and the sorting method provided by golang.
- Built-in sorting function
The sorting functions provided in golang are very convenient. For example, sort.Ints and sort.Strings provided by the sort package are very common. The following is an example of using sort.Ints for sorting:
package main import ( "fmt" "sort" ) func main() { nums := []int{3, 2, 1, 4, 5, 7, 6} sort.Ints(nums) fmt.Println(nums) }
The output result is: [1 2 3 4 5 6 7]
We can also use sort.Strings to sort strings :
package main import ( "fmt" "sort" ) func main() { strs := []string{"a", "c", "b", "d", "f", "e"} sort.Strings(strs) fmt.Println(strs) }
The output result is: [a b c d e f]
- Bubble sort algorithm
Bubble sort is a basic sorting algorithm and a comparison Easy to understand and implement. The basic principle is to compare adjacent elements, and swap positions if the order is wrong. After one round of sorting, the maximum or minimum value will reach the end of the sequence, and this process is repeated until all elements are in order. The following is the bubble sort algorithm implemented using go:
package main import "fmt" func bubbleSort(nums []int) { for i := len(nums)-1; i > 0; i-- { for j := 0; j < i; j++ { if nums[j] > nums[j+1] { nums[j], nums[j+1] = nums[j+1], nums[j] } } } } func main() { nums := []int{3,2,1,4,5,7,6} bubbleSort(nums) fmt.Println(nums) }
The output result is: [1 2 3 4 5 6 7]
- Quick sort algorithm
Quick sort is another common sorting algorithm. The basic principle is to divide the sequence to be sorted into two parts through one sorting. One part is smaller than the reference element, the other part is larger than the reference element, and then the two parts are quickly processed separately. Sort and finally get an ordered sequence. In the Go language, quick sorting is also relatively easy to implement. The code is as follows:
package main import "fmt" func quickSort(nums []int) []int { if len(nums) <= 1 { return nums } pivot := nums[0] var left, right []int for _, num := range nums[1:] { if num < pivot { left = append(left, num) } else { right = append(right, num) } } left = quickSort(left) right = quickSort(right) return append(append(left, pivot), right...) } func main() { nums := []int{3, 2, 1, 4, 5, 7, 6} nums = quickSort(nums) fmt.Println(nums) }
The output result is: [1 2 3 4 5 6 7]
- Merge sort algorithm
Merge sort is another relatively fast sorting algorithm. The basic principle is to divide the sequence to be sorted into two sequences, sort them separately and then merge them. Compared with the quick sort algorithm, merge sort does not require element exchange, so it can achieve "stable" sorting results. In golang, we can use recursion to implement the merge sort algorithm. The code is as follows:
package main import "fmt" func mergeSort(nums []int) []int { if len(nums) <= 1 { return nums } mid := len(nums) / 2 left, right := nums[:mid], nums[mid:] left = mergeSort(left) right = mergeSort(right) return merge(left, right) } func merge(left, right []int) []int { merged := make([]int, 0, len(left)+len(right)) for len(left) > 0 && len(right) > 0 { if left[0] <= right[0] { merged = append(merged, left[0]) left = left[1:] } else { merged = append(merged, right[0]) right = right[1:] } } merged = append(merged, left...) merged = append(merged, right...) return merged } func main() { nums := []int{3, 2, 1, 4, 5, 7, 6} nums = mergeSort(nums) fmt.Println(nums) }
The output result is: [1 2 3 4 5 6 7]
Summary:
In golang, we can use the built-in sort function to perform basic sorting operations. For some more complex sorting requirements, we can choose the corresponding sorting algorithm to implement according to the specific situation. Common sorting algorithms include bubble sort, quick sort and merge sort. When implementing, attention should be paid to the complexity and stability of the algorithm.
The above is the detailed content of How to sort in golang. For more information, please follow other related articles on the PHP Chinese website!
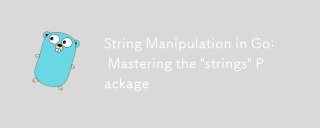
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
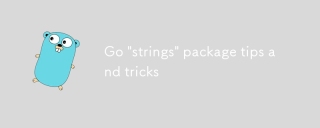
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
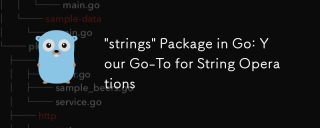
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
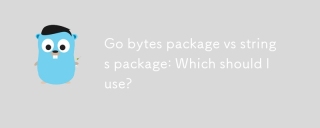
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
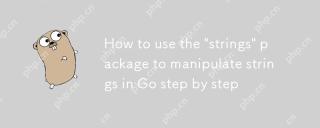
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
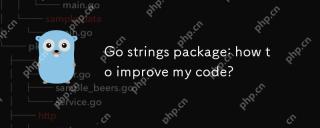
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
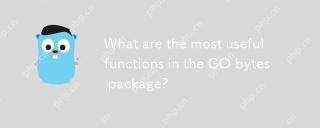
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
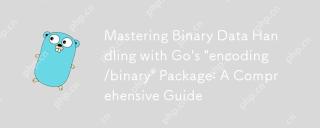
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
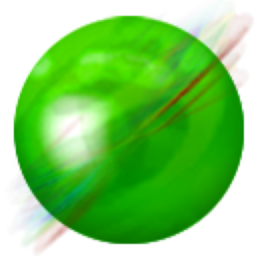
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
