As web development continues to evolve, testing has become an essential part. In web development, testing can help us ensure the quality of code and improve development efficiency. The PHPUnit framework is one of the most commonly used testing frameworks in PHP. It provides a wealth of testing tools and APIs, allowing developers to easily write and execute test cases. In this article, we will discuss how to use the PHPUnit framework for testing in PHP.
1. Install PHPUnit
PHPUnit is an independent PHP extension library that can be installed through Composer. Before installing PHPUnit, you need to ensure that Composer is installed. If it is not installed, please install it according to the instructions on the Composer official website.
Before installing PHPUnit, you need to install PHPUnit dependencies in the project:
composer require --dev phpunit/phpunit
This command will install the PHPUnit dependency package in the vendor
directory of the project as a development dependency Saved in the require-dev
section of the composer.json
file. After this command has finished running, we can use PHPUnit.
2. Create test cases
Before using PHPUnit for testing, you need to write test cases first. A test case is a collection of test codes for a specific function or method, used to verify the correctness of the code. In PHPUnit, a test case class can contain multiple test methods. Test methods start with test
and cannot accept any parameters.
Let's create a simple test case to verify the correctness of an addition function. First, we need to create a file named CalculatorTest.php
in the project root directory, and then write the test case in the file:
<?php use PHPUnitFrameworkTestCase; class CalculatorTest extends TestCase { public function testAddition() { $this->assertSame(2 + 2, 4); } }
In the above example, we wrote A test method named testAddition()
is used to verify whether 2 2 is equal to 4. Among them, we use the assertSame()
function to determine whether two values are equal. If the two values are not equal, the test case will fail.
3. Run the test case
After writing the test case, we can run the test case to verify the correctness of the code. In PHPUnit, you can run the test case through the following command:
./vendor/bin/phpunit CalculatorTest.php
After running the above command, PHPUnit will automatically run the test case we wrote in the CalculatorTest.php
file. If the test case runs successfully, a green symbol will be output; otherwise, a red symbol will be output and the reason for the test failure will be prompted.
4. Use the API provided by PHPUnit
PHPUnit provides many practical APIs, making it easier for us to write test cases.
- Assertion API
The most commonly used API in PHPUnit is the assertion API. It provides some functions for asserting test results, such as assertEmpty()
, assertNotNull()
, assertGreaterThan()
, etc. These functions can be used on test objects, strings, arrays, variables, and more.
The following are some commonly used assertion function examples:
$this->assertEquals(2 + 2, 4); // 两个值相等 $this->assertNotEmpty($array); // 非空数组 $this->assertInstanceOf(MyClass::class, $object); // 类的实例
- Dataset API
PHPUnit provides a Dataset API that can be used in the same test Test multiple sets of data in use cases. The way to use it is to use the test data as an array, and then use the @dataProvider
annotation to specify the data provider function, as shown below:
/** * @dataProvider additionProvider */ public function testAddition($a, $b, $expected) { $this->assertSame($a + $b, $expected); } public function additionProvider() { return [ [0, 0, 0], [0, 1, 1], [1, 0, 1], [1, 1, 2], ]; }
In the above example, we used @ dataProvider
annotation, set the name of the test data providing function to additionProvider
. This function returns an array containing multiple sets of test data.
5. Summary
In this article, we introduced how to use the PHPUnit framework for testing in PHP. We first introduced the installation and use of PHPUnit, then demonstrated how to create a test case and run the test case, and finally introduced some practical APIs provided by PHPUnit. Using the PHPUnit framework can help us write and execute test cases more conveniently, thereby improving code quality and development efficiency.
The above is the detailed content of How to use PHPUnit framework for testing in PHP. For more information, please follow other related articles on the PHP Chinese website!
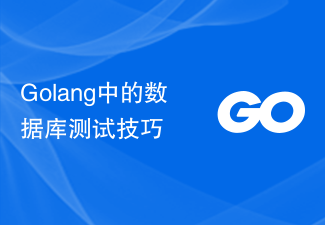
Golang中的数据库测试技巧引言:在开发应用程序时,数据库测试是一个非常重要的环节。合适的测试方法可以帮助我们发现潜在的问题并确保数据库操作的正确性。本文将介绍Golang中的一些常用数据库测试技巧,并提供相应的代码示例。一、使用内存数据库进行测试在编写数据库相关的测试时,我们通常会面临一个问题:如何在不依赖外部数据库的情况下进行测试?这里我们可以使用内存
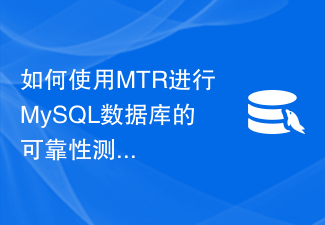
如何使用MTR进行MySQL数据库的可靠性测试?概述:MTR(MySQL测试运行器)是MySQL官方提供的一个测试工具,可以帮助开发人员进行MySQL数据库的功能和性能测试。在开发过程中,为了确保数据库的可靠性和稳定性,我们经常需要进行各种测试,而MTR提供了一种简单方便且可靠的方法来进行这些测试。步骤:安装MySQL测试运行器:首先,需要从MySQL官方网
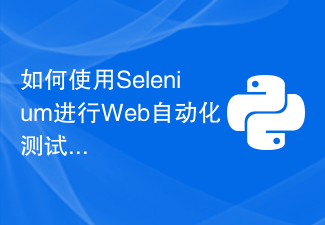
如何使用Selenium进行Web自动化测试概述:Web自动化测试是现代软件开发过程中至关重要的一环。Selenium是一个强大的自动化测试工具,可以模拟用户在Web浏览器中的操作,实现自动化的测试流程。本文将介绍如何使用Selenium进行Web自动化测试,并附带代码示例,帮助读者快速上手。环境准备在开始之前,需要安装Selenium库和Web浏览器驱动程
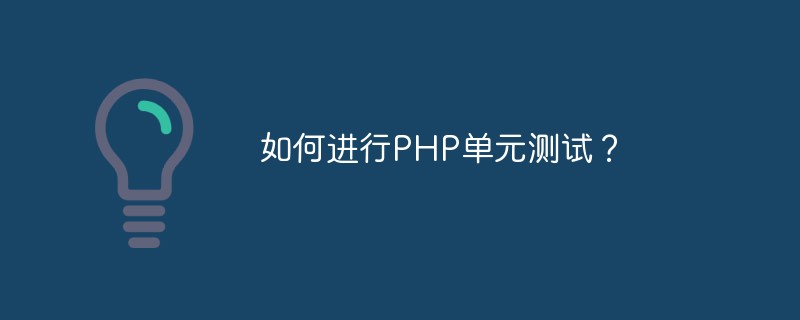
在Web开发中,PHP是一种流行的语言,因此对于任何人来说,对PHP进行单元测试是一个必须掌握的技能。本文将介绍什么是PHP单元测试以及如何进行PHP单元测试。一、什么是PHP单元测试?PHP单元测试是指测试一个PHP应用程序的最小组成部分,也称为代码单元。这些代码单元可以是方法、类或一组类。PHP单元测试旨在确认每个代码单元都能按预期工作,并且能否正确地与
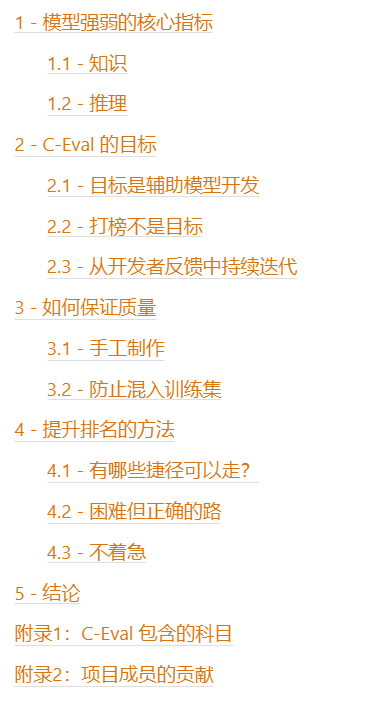
ChatGPT的出现,使中文社区意识到与国际领先水平的差距。近期,中文大模型研发如火如荼,但中文评价基准却很少。在OpenAIGPT系列/GooglePaLM系列/DeepMindChinchilla系列/AnthropicClaude系列的研发过程中,MMLU/MATH/BBH这三个数据集发挥了至关重要的作用,因为它们比较全面地覆盖了模型各个维度的能力。最值得注意的是MMLU这个数据集,它考虑了57个学科,从人文到社科到理工多个大类的综合知识能力。DeepMind的Gopher和Chinchi
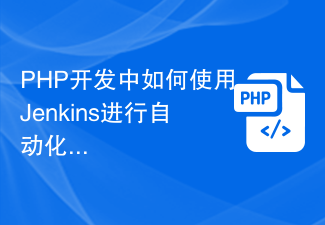
随着Web应用程序规模的不断扩大,PHP语言的应用也越来越广泛。在软件开发过程中,自动化测试可以大大提高开发效率和软件质量。而Jenkins是一个可扩展的开源自动化服务器,它能够自动执行软件构建、测试、部署等操作,今天我们来看一下在PHP开发中如何使用Jenkins进行自动化测试。一、Jenkins的安装和配置首先,我们需要在服务器上安
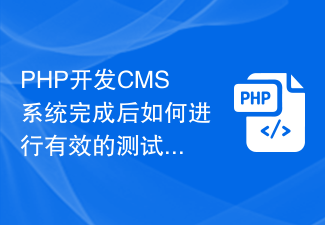
在日益发展的互联网时代中,CMS系统已经成为了网络建设中的一项重要工具。其中PHP语言开发的CMS系统因其简单易用,自由度高,成为了经典的CMS系统之一。然而,PHP开发CMS系统过程中的测试工作也是至关重要的。只有经过完善、系统的测试工作,我们才可以保证开发出的CMS系统在使用中更加稳定、可靠。那么,如何进行有效的PHP开发CMS系统测试呢?一、测试流程的
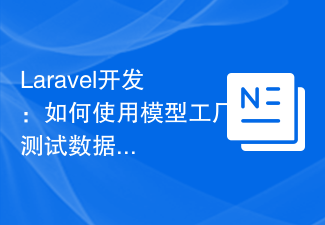
Laravel是一个流行的PHPWeb开发框架,以其简洁易用的API设计,丰富的函数库和强大的生态系统而著名。在使用Laravel进行项目开发时,测试是非常重要的一个环节。Laravel提供了多种测试工具和技术,其中模型工厂是其中的重要组成部分。本文将介绍如何在Laravel项目中使用模型工厂来测试数据库。一、模型工厂的作用在Laravel中,模型工厂是用


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
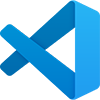
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
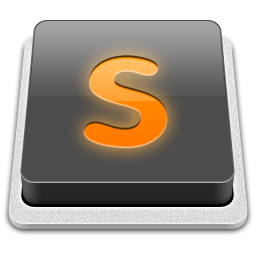
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Zend Studio 13.0.1
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
