Go language (Golang) is a programming language similar to C language, mainly used for developing efficient and reliable programming applications. Compared with other programming languages, Golang's advantages include high performance, high development efficiency, high concurrency, and strong error handling capabilities. In actual development, we often need to operate data in JSON format. This article will introduce how to use Golang to modify data in JSON format.
1. Introduction to JSON
JSON stands for JavaScript Object Notation, which is a lightweight data exchange format. It is based on a subset of the JavaScript language standard (ECMA-262 version 3, December 1999). Due to the specification and readability of JSON format, it is widely used in web applications.
2. Using JSON in Golang
In Golang, we can use the encoding/json package to process JSON format. This package provides functions such as Marshal, Unmarshal, NewEncoder, and NewDecoder, which can implement JSON parsing and generation operations.
- Parsing JSON
Parsing JSON usually uses the json.Unmarshal() function. Suppose we have the following JSON string:
{ "name": "Tom", "age": 18, "gender": "male", "score": [88, 90, 95] }
We can define the following structure to represent JSON data:
type Student struct { Name string `json:"name"` Age int `json:"age"` Gender string `json:"gender"` Score []int `json:"score"` }
Use the Unmarshal() function to parse the JSON string into the Student structure:
var str = `{ "name": "Tom", "age": 18, "gender": "male", "score": [88, 90, 95] }` var std Student err := json.Unmarshal([]byte(str), &std) if err != nil { panic(err.Error()) } fmt.Println(std.Name, std.Age, std.Gender, std.Score)
Output after running:
Tom 18 male [88 90 95]
- Generate JSON
The json.Marshal() function is usually used to generate JSON. We can define a structure Student:
type Student struct { Name string `json:"name"` Age int `json:"age"` Gender string `json:"gender"` Score []int `json:"score"` }
and convert the structure into a JSON string:
std := Student{ Name: "Tom", Age: 18, Gender: "male", Score: []int{88, 90, 95}, } result, err := json.Marshal(std) if err != nil { panic(err.Error()) } fmt.Println(string(result))
Output:
{"name":"Tom","age":18,"gender":"male","score":[88,90,95]}
3. Modify JSON
In Golang, we can use some simple ways to modify data in JSON format. Suppose we have the following JSON data:
{ "name": "Tom", "age": 18, "gender": "male", "score": [88, 90, 95] }
We can first use the Unmarshal() function to parse the JSON string into Map format:
var str = `{ "name": "Tom", "age": 18, "gender": "male", "score": [88, 90, 95] }` var data map[string]interface{} err := json.Unmarshal([]byte(str), &data) if err != nil { panic(err.Error()) }
Next we can modify a value in the data:
data["score"] = []int{99, 98, 97}
Finally, use the Marshal() function to convert the Map into a JSON string:
result, err := json.Marshal(data) if err != nil { panic(err.Error()) } fmt.Println(string(result))
Output:
{"age":18,"gender":"male","name":"Tom","score":[99,98,97]}
We can also use struct to modify the JSON:
type Student struct { Name string `json:"name"` Age int `json:"age"` Gender string `json:"gender"` Score []int `json:"score"` } var str = `{ "name": "Tom", "age": 18, "gender": "male", "score": [88, 90, 95] }` var std Student err := json.Unmarshal([]byte(str), &std) if err != nil { panic(err.Error()) } std.Score = []int{99, 98, 97} result, err := json.Marshal(std) if err != nil { panic(err.Error()) } fmt.Println(string(result))
Output:
{"name":"Tom","age":18,"gender":"male","score":[99,98,97]}
4. Summary
This article mainly introduces the methods of processing JSON format in Golang, including parsing JSON, generating JSON, modifying JSON, etc. The JSON data format is a lightweight and easy-to-read data exchange format, and Golang provides strong support through the encoding/json package. In actual development, we should choose different operating methods as needed to improve the efficiency and maintainability of the program.
The above is the detailed content of golang modify json. For more information, please follow other related articles on the PHP Chinese website!
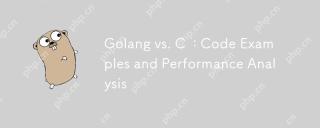
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
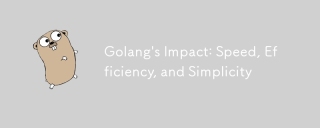
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
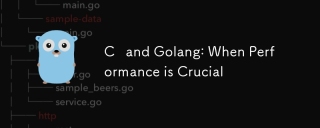
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
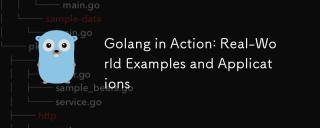
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
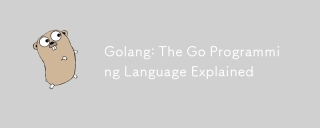
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
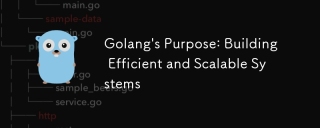
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
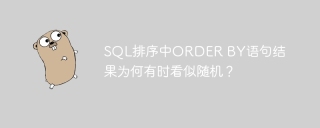
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
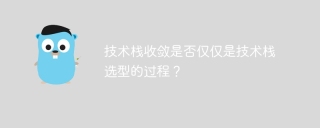
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
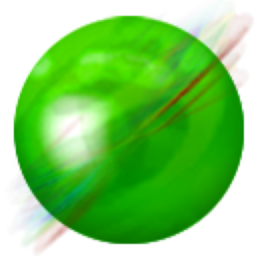
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
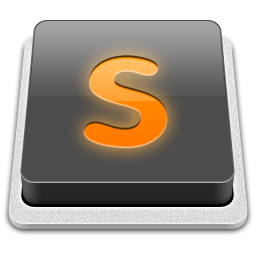
SublimeText3 Mac version
God-level code editing software (SublimeText3)