JavaScript is a scripting language used to add interactivity and dynamic effects to web pages. In web development, tables are one of the commonly used elements. By using JavaScript, you can easily modify the style of a table to make it more beautiful and easier to read.
1. Add styles to the table
Before we start modifying the table style, we need to add styles to the table. We use CSS to define styles for the table, and then use JavaScript to modify those styles. The following example demonstrates how to add styles to a table:
<!DOCTYPE html> <html> <head> <style> table { border-collapse: collapse; width: 100%; } th, td { text-align: left; padding: 8px; border-bottom: 1px solid #ddd; } tr:nth-child(even) { background-color: #f2f2f2; } </style> </head> <body> <table> <tr> <th>姓名</th> <th>年龄</th> <th>性别</th> </tr> <tr> <td>张三</td> <td>25</td> <td>男</td> </tr> <tr> <td>李四</td> <td>32</td> <td>女</td> </tr> <tr> <td>王五</td> <td>18</td> <td>男</td> </tr> </table> </body> </html>
In the above example, we defined the following styles:
-
border-collapse: collapse;
means Merge the borders of cells. -
width: 100%;
Indicates that the table occupies 100% of the width of the parent container. -
th, td
Indicates the style of the table header and table data -
text-align: left;
Indicates that the data is left aligned. -
padding: 8px;
means the distance between the data and the border is 8 pixels. -
border-bottom: 1px solid #ddd;
Indicates that the border thickness at the bottom of each row of data is 1 pixel and the color is light gray. -
tr:nth-child(even)
means changing the background color every other line.
2. Use JavaScript to modify the table style
Now that we have defined the styles for the table, the next step is to use JavaScript to modify these styles. Here are some examples that demonstrate how to change the table style using JavaScript:
- Change the table background color:
document.getElementsByTagName("table")[0].style.backgroundColor = "#f9f9f9";
This example changes the background color of the table to light gray.
- Change the header background color:
var th = document.getElementsByTagName("th"); for (var i = 0; i < th.length; i++) { th[i].style.backgroundColor = "#ddd"; }
This example changes the background color of the header to light gray.
- Change the font size of all cells in the table:
var td = document.getElementsByTagName("td"); for (var i = 0; i < td.length; i++) { td[i].style.fontSize = "16px"; }
This example changes the font size of all cells in the table to 16 pixels.
- Change the font color of all even-numbered rows in the table:
var tr = document.getElementsByTagName("tr"); for (var i = 1; i < tr.length; i += 2) { var td = tr[i].getElementsByTagName("td"); for (var j = 0; j < td.length; j++) { td[j].style.color = "#999"; } }
This example changes the font color of all even-numbered rows in the table to light gray.
- Change the border style of the cells in the first column of the table:
var td = document.getElementsByTagName("td"); for (var i = 0; i < td.length; i += 3) { td[i].style.borderRight = "1px solid #ddd"; }
This example changes the right border of the cells in the first column of the table to 1 pixel lighter Gray border.
- Hide the last column in the table:
var table = document.getElementsByTagName("table")[0]; var td = table.getElementsByTagName("td"); for (var i = td.length - 1; i >= 0; i--) { if ((i + 1) % 3 === 0) { td[i].parentNode.removeChild(td[i]); } }
This example deletes the last column of cells in the table.
Summary
In this article, we learned how to modify table styles using JavaScript. By using these techniques, you can easily create and modify beautified tables. Depending on your needs, you can use JavaScript to add dynamic effects and interactivity to the table. Now that you have learned how to modify table styles, try using JavaScript to beautify your tables!
The above is the detailed content of How to modify table style with javascript. For more information, please follow other related articles on the PHP Chinese website!
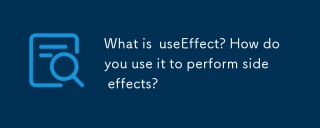
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
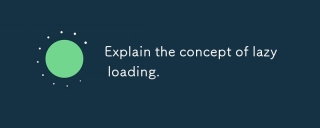
Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
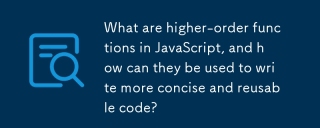
Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
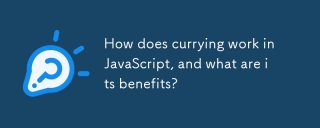
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
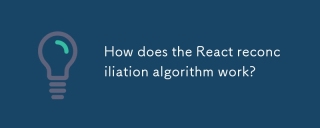
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
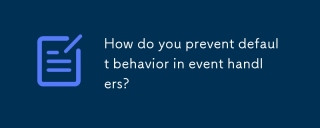
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
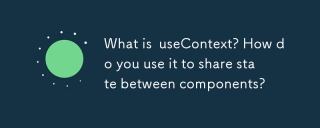
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
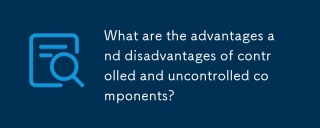
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
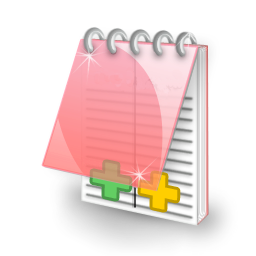
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
