Pixi.js is a lightweight JavaScript library for creating 2D games and interactive applications. Its API is simple and easy to use, providing many tools and features to make it faster and easier for developers to create beautiful interfaces and user experiences for games and applications.
Now, let’s take a look at how to use Pixi.js to create a simple interactive application.
Step 1: Download Pixi.js
To use Pixi.js, we first need to download it to your local computer. We can download the latest version of the library file on the official website (https://www.pixijs.com/). Once the download is complete, we unzip it and add the js file to our project.
Step 2: Add a reference to Pixi.js in the HTML file
Next, add a reference to Pixi.js in our HTML file. We can add Pixi.js to our HTML file using the script tag as shown below:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>使用Pixi.js创建交互式应用程序</title> <script src="pixi.min.js"></script> </head> <body> <div id="app"></div> </body> </html>
In the above example, we added the Pixi.js file to our HTML as the src attribute of the script tag in the file. We also added a div element with the id "app" which will be used to display our application.
Step 3: Create a Pixi.js Application
Now that we have added a reference to Pixi.js to our HTML file, let’s start building our Pixi.js application . In this application we will draw a simple shape with interactions.
First, we need to create an instance of the Pixi application and add it to our HTML document. We can do it using the following code:
// 创建一个应用程序实例 const app = new PIXI.Application({ width: 800, height: 600 }); // 将应用程序实例添加到我们的HTML文档中 document.getElementById('app').appendChild(app.view);
In the above code, we created a 800 using the new PIXI.Application({width: 800, height: 600})
statement An example of a Pixi application that is 600 pixels wide and 600 pixels high. We then added this instance to the HTML document using the document.getElementById('app').appendChild(app.view)
statement.
Step 4: Draw a Simple Shape
Now that we have created a Pixi application instance, we need to draw a simple shape on the canvas. We will draw a rectangle 200 pixels wide and 100 pixels high. We can accomplish this task using the following code:
// 创建一个矩形形状 const rectangle = new PIXI.Graphics(); rectangle.beginFill(0xFF0000); rectangle.drawRect(0, 0, 200, 100); rectangle.endFill(); // 将矩形形状添加到舞台上 app.stage.addChild(rectangle);
In the above code, we first create a PIXI.Graphics object named "rectangle". We use the rectangle.beginFill(0xFF0000)
statement to set the fill color of the rectangle to red. Then, we use the rectangle.drawRect(0, 0, 200, 100)
statement to draw a rectangle on the graphic. Finally, we use the rectangle.endFill()
statement to end the drawing of the graphic.
Step 5: Add interactivity to the shape
Now that we have drawn a simple shape, let’s make it more interactive by adding interactive features to it. We will enable the user to zoom in on the rectangle when the mouse pointer is over it and zoom out when the mouse button is pressed. We can achieve these effects using the following code:
// 将形状设置为可交互 rectangle.interactive = true; // 当鼠标指针悬停在矩形上时放大它 rectangle.on('mouseover', function() { rectangle.scale.set(1.2); }); // 当鼠标按钮被按下时缩小它 rectangle.on('mousedown', function() { rectangle.scale.set(1); });
In the above code, we first set the rectangle.interactive
property to true
to make it responsive User interaction events. We then use the rectangle.on('mouseover', function(){})
statement to add a callback function on the "mouseover" event that occurs when the mouse pointer hovers over the rectangle. When this event occurs, we use the rectangle.scale.set(1.2)
statement to enlarge the rectangle to 1.2 times its original size.
Similarly, we use the rectangle.on('mousedown', function(){})
statement to add a callback function on the "mousedown" event that occurs when the mouse button is pressed . When this event occurs, we use the rectangle.scale.set(1)
statement to shrink the rectangle to its original size.
Step 6: Run the application
Now, we have completed our Pixi.js application. In order to run it, we just need to open our HTML file in a browser. When we hover the mouse pointer over the rectangle, it will enlarge to 1.2 times its original size. When we press the mouse button it will shrink to the original size.
Pixi.js is an excellent JavaScript library for creating 2D games and interactive applications. Using Pixi.js, we can easily add complex interaction designs to our applications and provide a great user experience, which makes it ideal for creating high-quality browser applications.
The above is the detailed content of How to use pixi with JavaScript. For more information, please follow other related articles on the PHP Chinese website!
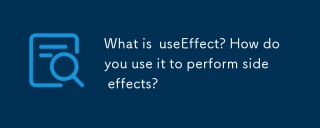
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
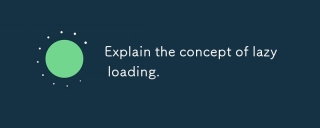
Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
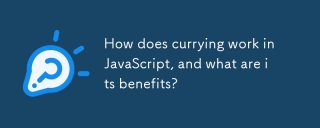
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
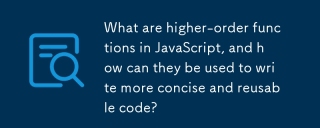
Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
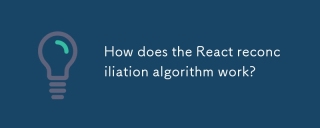
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
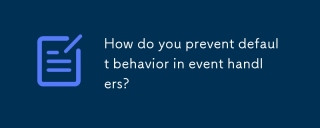
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
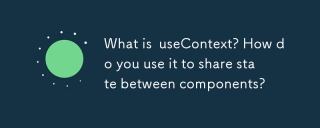
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
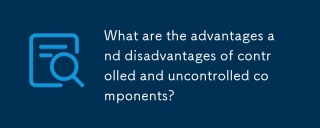
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version
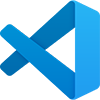
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
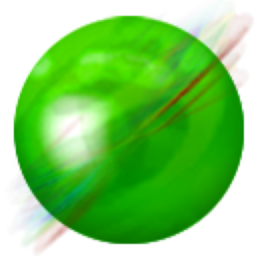
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
