In Laravel development, multi-table joint query is a common operation. Join query can combine data from multiple tables according to specific conditions and return a result set of the required data. To implement multi-table joint query in Laravel, you need to use the powerful functions provided by Eloquent ORM. This article will introduce how to use Laravel multi-table joint query.
- Eloquent ORM
Laravel's Eloquent ORM is an object-relational mapping (ORM) technology that provides a flexible and simple way to access and operate databases. Eloquent ORM achieves this by mapping database tables to objects. These objects can be manipulated by PHP code and have their state persisted to the database. The main advantage of ORM is that it converts complex SQL statements into simple object method calls, so developers can focus on code logic rather than SQL statements.
- Multiple table query
Laravel provides a variety of methods to implement multi-table joint query. Among them, the most common method is through the use of Eloquent correlation. Since Laravel's Eloquent ORM provides powerful correlation functions, it is very convenient to use Eloquent correlations in multi-table queries. The following are some commonly used multi-table query methods.
2.1 One-to-one association
One-to-one association means that there is only one matching row between the two tables. Implementing a one-to-one association in Laravel is very simple, just define the hasOne or belongsTo method in your model.
For example, consider the following two tables:
users
id | name | email | password | created_at | updated_at
profiles
id | user_id | phone | address | created_at | updated_at
To find a user's profile, you can define a hasOne relationship in the User model:
class User extends Model
{
public function profile() { return $this->hasOne('AppModelsProfile'); }
}
Then, define a belongsTo method in the Profile model to specify the association:
class Profile extends Model
{
public function user() { return $this->belongsTo('AppModelsUser'); }
}
Now you can use the following code to get the user and his profile:
$user = User::with('profile')->find(1);
In this example, the with method is used to perform Eager Loading. This will fetch the user and the profiles associated with them in one query, thus avoiding redundant queries. The find method is used to find the user with the specified ID. Note that when defining a relationship, Eloquent will name the foreign key as the name of the related model "_id" by default, such as the "user_id" field in this example.
2.2 One-to-many association
One-to-many association means that one row in one table can be associated with multiple rows in another table. Implementing a one-to-many association in Laravel is very simple, just define the hasMany or belongsTo method in the model.
For example, consider the following two tables:
users
id | name | email | password | created_at | updated_at
posts
id | user_id | title | body | created_at | updated_at
To find all articles of a user, you can define a hasMany relationship in the User model:
class User extends Model
{
public function posts() { return $this->hasMany('AppModelsPost'); }
}
Then, define a belongsTo method in the Post model to specify the association:
class Post extends Model
{
public function user() { return $this->belongsTo('AppModelsUser'); }
}
You can now use the following code to get the user and all his posts:
$user = User::with('posts')->find(1);
In this example, Eloquent will automatically find all posts with user_id equal to the current user ID and set them as the posts attribute of the user model. Note that when defining a relationship, Eloquent will name the foreign key as the name of the related model "_id" by default, such as the "user_id" field in this example.
2.3 Many-to-many association
Many-to-many association means that there can be multiple matching rows between two tables. To implement a many-to-many association in Laravel, you need to use the belongsToMany method.
For example, consider the following two tables:
users
id | name | email | password | created_at | updated_at
roles
id | name | label | created_at | updated_at
Intermediate table roles_users
id | user_id | role_id
To find all users with a specified role, you can in the Role model Define a belongsToMany relationship:
class Role extends Model
{
public function users() { return $this->belongsToMany('AppModelsUser'); }
}
Then, also define a belongsToMany method in the User model to specify the association:
class User extends Model
{
public function roles() { return $this->belongsToMany('AppModelsRole'); }
}
You can now use the following code to get all users with a specified role:
$users = Role ::with('users')->where('name', 'admin')->get();
In this example, the with method is used to execute Eager Loading, and the get method is used to get all users with the specified role. Note that a many-to-many association requires the existence of an intermediate table, which is used to save the relationship between the two tables into the database.
- Summary
Laravel is a powerful web development framework that provides many convenient features to simplify the development process, one of which is Eloquent ORM. Eloquent ORM provides a simple and powerful way to deal with databases, including multi-table join queries. If we are familiar with using Laravel's Eloquent correlation, implementing multi-table joint query is a simple matter.
The above is the detailed content of How to join multiple tables in laravel. For more information, please follow other related articles on the PHP Chinese website!
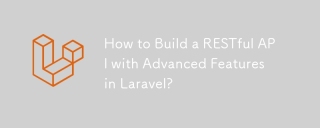
This article guides building robust Laravel RESTful APIs. It covers project setup, resource management, database interactions, serialization, authentication, authorization, testing, and crucial security best practices. Addressing scalability chall
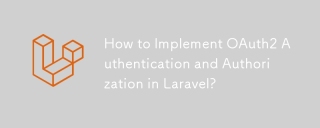
This article details implementing OAuth 2.0 authentication and authorization in Laravel. It covers using packages like league/oauth2-server or provider-specific solutions, emphasizing database setup, client registration, authorization server configu
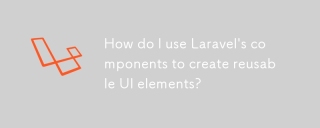
The article discusses creating and customizing reusable UI elements in Laravel using components, offering best practices for organization and suggesting enhancing packages.
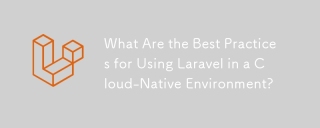
The article discusses best practices for deploying Laravel in cloud-native environments, focusing on scalability, reliability, and security. Key issues include containerization, microservices, stateless design, and optimization strategies.
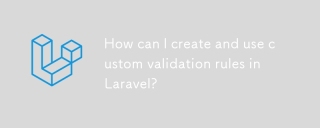
The article discusses creating and using custom validation rules in Laravel, offering steps to define and implement them. It highlights benefits like reusability and specificity, and provides methods to extend Laravel's validation system.
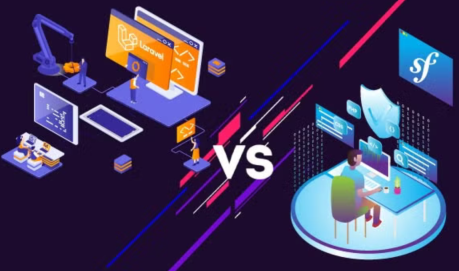
When it comes to choosing a PHP framework, Laravel and Symfony are among the most popular and widely used options. Each framework brings its own philosophy, features, and strengths to the table, making them suited for different projects and use cases. Understanding their differences and similarities is critical to selecting the right framework for your development needs.
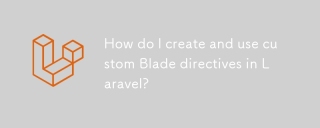
The article discusses creating and using custom Blade directives in Laravel to enhance templating. It covers defining directives, using them in templates, and managing them in large projects, highlighting benefits like improved code reusability and r
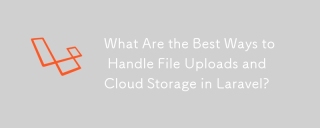
This article explores optimal file upload and cloud storage strategies in Laravel. It examines local storage vs. cloud providers (AWS S3, Google Cloud, Azure, DigitalOcean), emphasizing security (validation, sanitization, HTTPS) and performance opti


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
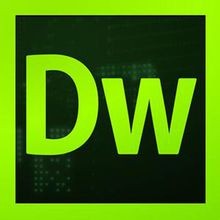
Dreamweaver CS6
Visual web development tools
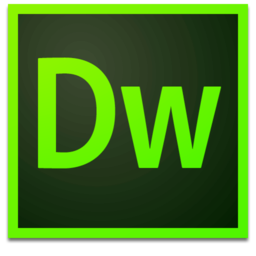
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.